Java Reference
In-Depth Information
Now that our class has this method, the preceding client code produces the following
output.
i is 42
s is hello
p is (0, 0)
Note that the client code didn't explicitly call the
toString
method; the compiler
did it automatically because the
Point
object was being concatenated with a
String
.
The
toString
method is also implicitly called when printing an object by itself, as
in the following code:
System.out.println(p);
In order for this implicit calling behavior to work properly, your
toString
method's signature must exactly match the one shown in this section. Changing the
name or signature even slightly (for example, naming the method
ToString
with a
capital T, or
convertToString
) will cause the class to produce the old output (e.g.,
"
Point@119c082
"
). The reason has to do with concepts called inheritance and over-
riding that we will explore in the next chapter.
It is also legal to call
toString
explicitly if you prefer. The following client code
uses an explicit
toString
call and produces the same output as the original client
code:
System.out.println("p is " + p.toString());
Sun's Java guidelines recommend writing a
toString
method in every class you
write.
Common Programming Error
println
Statement in
toString
Method
Since the
toString
method is closely related to printing, some students mistak-
enly think that they should place
println
statements in their
toString
meth-
ods, as in the following method:
// this toString method is flawed;
// it should return the String rather than printing it
public String toString() {
System.out.println("(" + x + ", " + y + ")");
return "";
}
Continued on next page
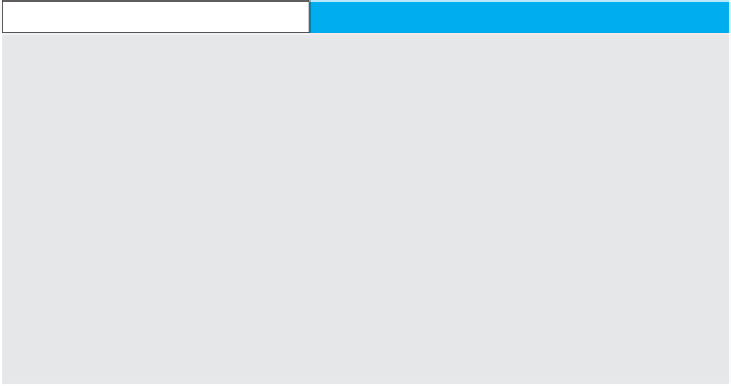

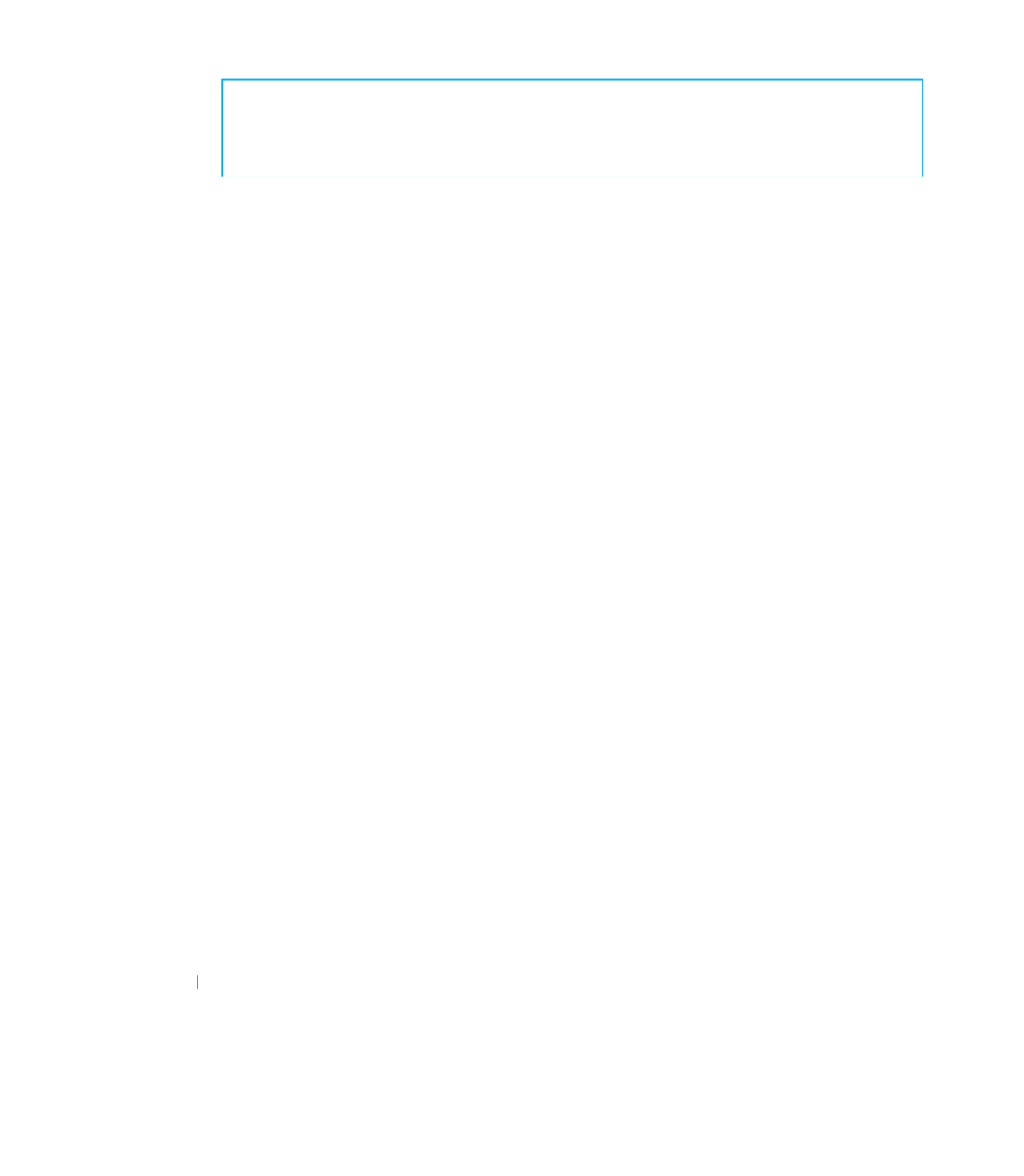
Search WWH ::

Custom Search