Java Reference
In-Depth Information
Section 5.3: The
boolean
Type
14.
Consider the following variable declarations:
int x = 27;
int y = -1;
int z = 32;
boolean b = false;
What is the value of each of the following Boolean expressions?
a.
!b
b.
b || true
c.
(x > y) && (y > z)
d.
(x == y) || (x <= z)
e.
!(x % 2 == 0)
f.
(x % 2 != 0) && b
g.
b && !b
h.
b || !b
i.
(x < y) == b
j.
!(x / 2 == 13) || b || (z * 3 == 96)
k.
(z < x) == false
l.
!((x > 0) && (y < 0))
15.
Write a method called
isVowel
that accepts a character as input and returns
true
if that character is a vowel (a, e, i,
o, or u). For an extra challenge, make your method case-insensitive.
16.
The following code attempts to examine a number and return whether that number is prime (i.e., has no factors other
than 1 and itself). A flag named
prime
is used. However, the Boolean logic is not implemented correctly, so the
method does not always return the correct answer. In what cases does the method report an incorrect answer? How
can the code be changed so that it will always return a correct result?
public static boolean isPrime(int n) {
boolean prime = true;
for (int i = 2; i < n; i++) {
if (n % i = = 0) {
prime = false;
} else {
prime = true;
}
}
return prime;
}
17.
The following code attempts to examine a
String
and return whether it contains a given letter. A flag named
found
is used. However, the Boolean logic is not implemented correctly, so the method does not always return the correct
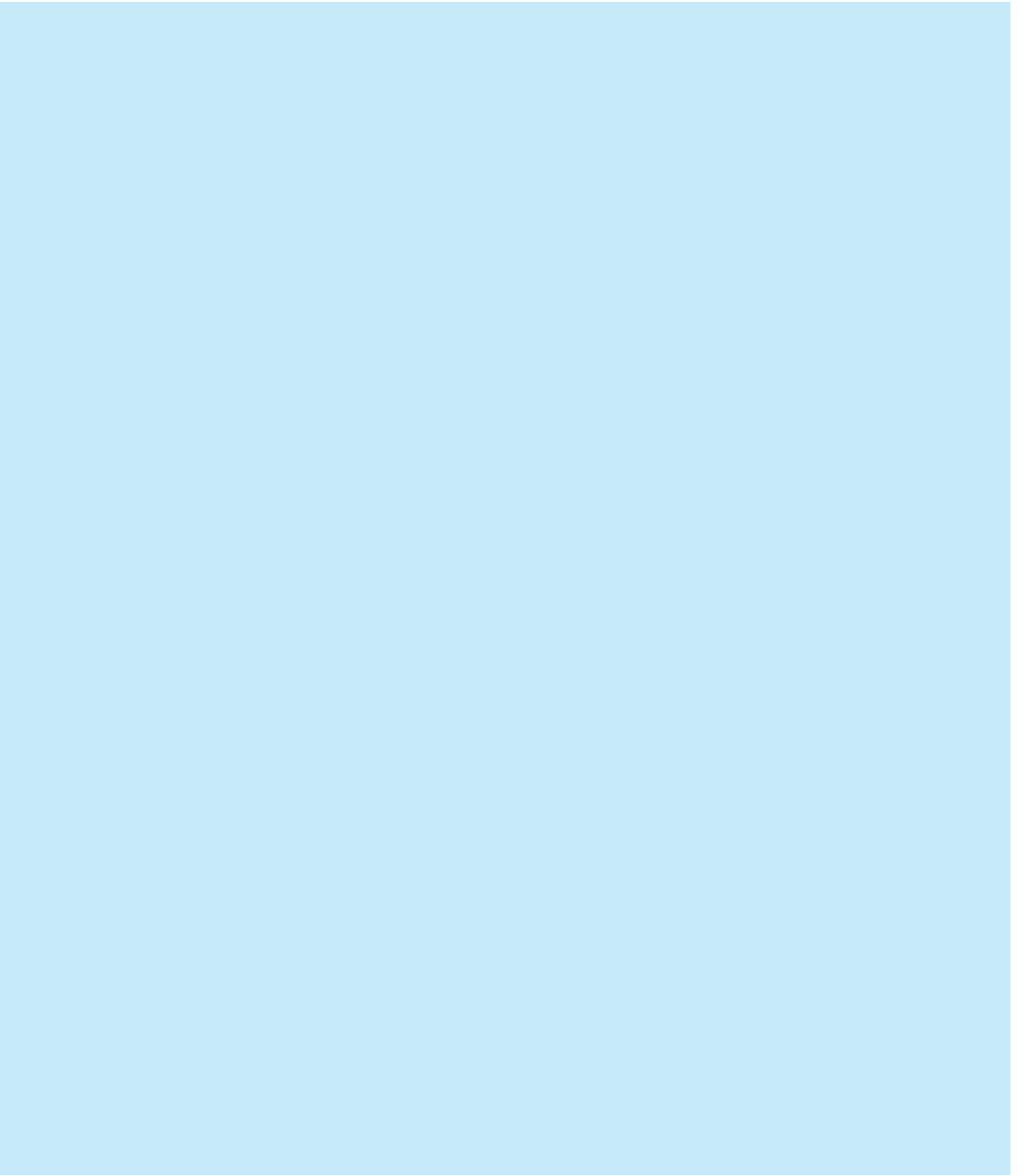
Search WWH ::

Custom Search