Java Reference
In-Depth Information
Table 5.2
Useful Methods of
Random
Objects
Method
Description
Random integer between
-2
31
and
(2
31
-1)
nextInt()
Random integer between
0
and
(max - 1)
nextInt(max)
Random real number between
0.0
(inclusive) and
1.0
(exclusive)
nextDouble()
Random logical value of
true
or
false
nextBoolean()
To create random numbers, you first construct a
Random
object:
Random r = new Random();
You can then call its
nextInt
method, passing it a maximum integer. The number
returned will be between 0 (inclusive) and the maximum (exclusive). For example, if
you call
nextInt(100)
, you will get a number between 0 and 99. You can add 1 to
the number to have a range between 1 and 100.
Let's look at a simple program that picks numbers between 1 and 10 until a particu-
lar number comes up. We'll use the
Random
class to construct an object for generating
our pseudorandom numbers.
Our loop should look something like this (where
number
is the value the user has
asked us to generate):
int result;
while (result != number) {
result = r.nextInt(10) + 1; // random number from 1-10
System.out.println("next number = " + result);
}
Notice that we have to declare the variable
result
outside the
while
loop,
because
result
appears in the
while
loop test. The preceding code has the right
approach, but Java won't accept it. The code generates an error message that the vari-
able
result
might not be initialized. This is an example of a loop that needs
priming.
Priming a Loop
Initializing variables before a loop to “prime the pump” and guarantee that
the loop is entered.
We want to set the variable
result
to something that will cause the loop to be
entered, but the value isn't important as long as it gets us into the loop. We do want to
be careful not to set it to a value the user wants us to generate, though. We are dealing
with values between 1 and 10 in this program, so we could set
result
to a value
such as
-1
that is clearly outside this range of numbers. We sometimes refer to this as


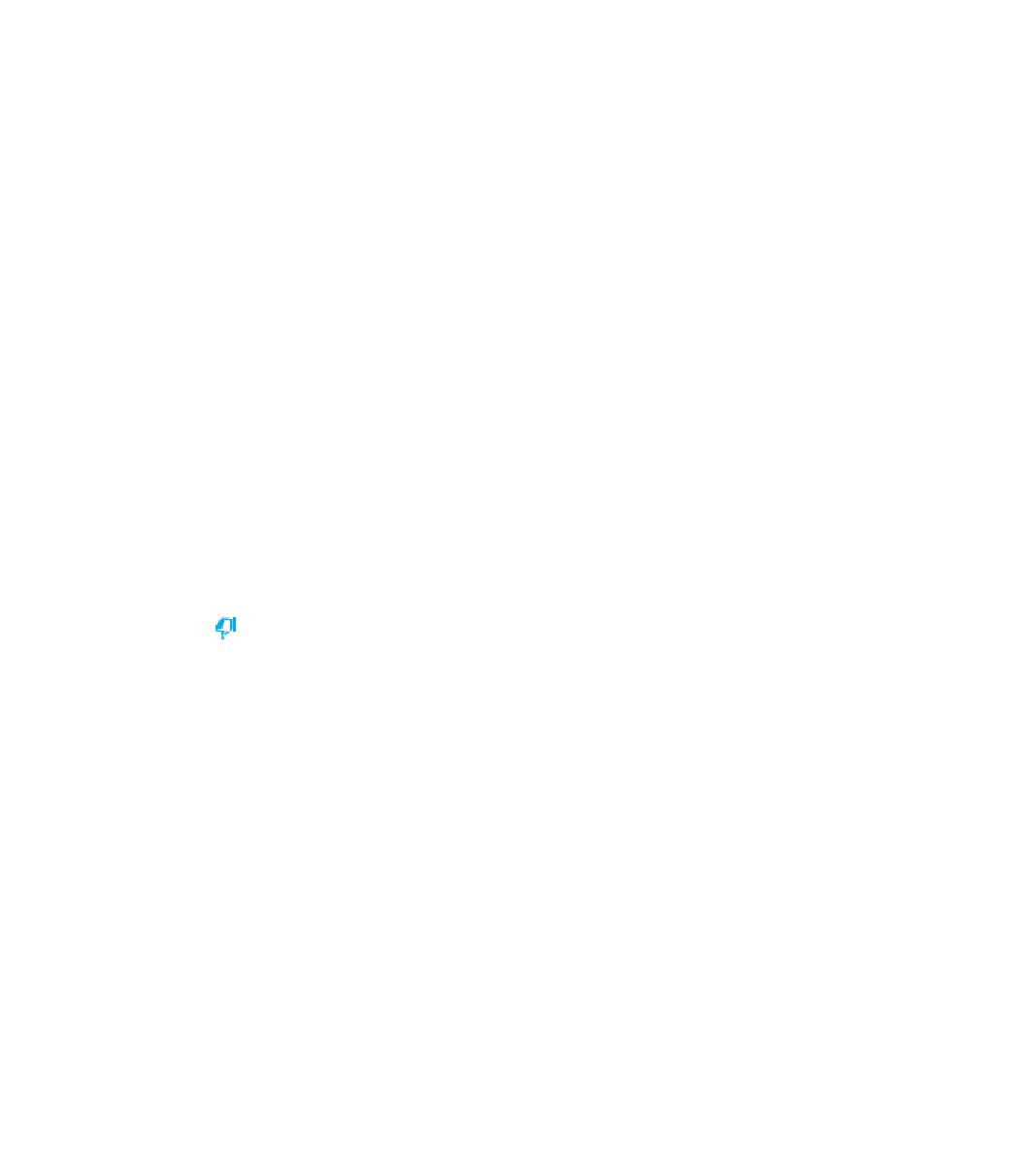
Search WWH ::

Custom Search