Java Reference
In-Depth Information
Continued from previous page
The following version of the code solves the infinite loop problem. The loop
contains an update step on each pass that divides the integer in half and stores its
new value. If the integer hasn't reached 0, the loop repeats:
// this code behaves correctly
int number = console.nextInt(); // moved out of loop
while (number > 0) {
number = number / 2; // update step: divide in half
System.out.println(number);
}
The key idea is that every
while
loop's body should contain code to update the
terms that are tested in the loop test. If the
while
loop test examines a variable's value,
the loop body should potentially reassign a meaningful new value to that variable.
We often want our programs to exhibit apparently random behavior. For example, we
want game-playing programs to make up a number for the user to guess, shuffle a
deck of cards, pick a word from a list for the user to guess, and so on. Programs are,
by their very nature, predictable and nonrandom. But we can produce values that
seem to be random. Such values are called
pseudorandom
because they are produced
algorithmically.
Pseudorandom Numbers
Numbers that, although they are derived from predictable and well-defined
algorithms, mimic the properties of numbers chosen at random.
Java provides several mechanisms for obtaining pseudorandom numbers. One
option is to call the
random
method from the
Math
class to obtain a random value of
type
double
that has the following property:
0.0
…
Math.random()
< 1.0
This method provides a quick and easy way to get a random number, and you can
use multiplication to change the range of the numbers the method produces. Java also
provides a class called
Random
that can be easier to use. It is included in the
java.util
package, so you have to include an import declaration at the beginning of
your program to use it.
Random
objects have several useful methods that are related to generating pseudo-
random numbers, listed in Table 5.2. Each time you call one of these methods, Java
will generate and return a new random number of the requested type.
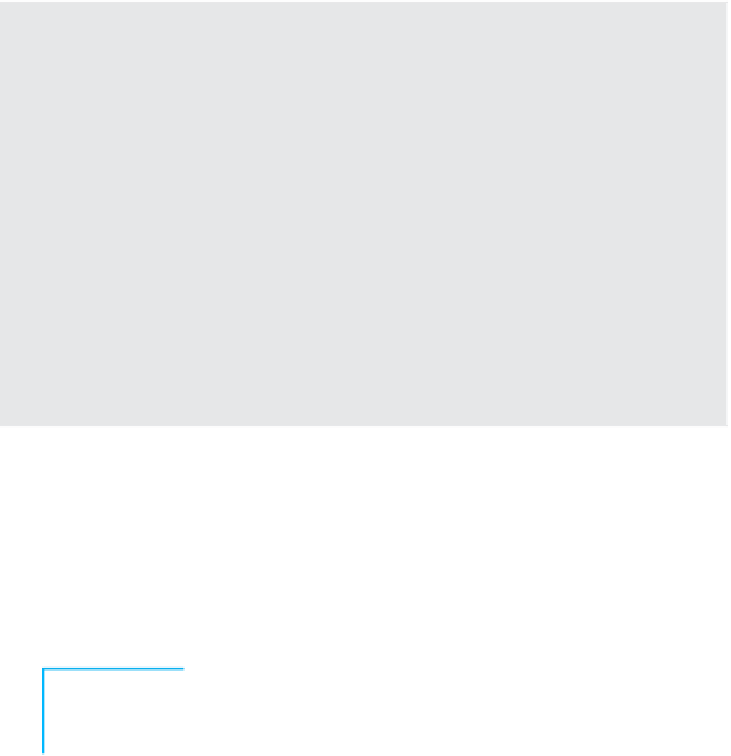
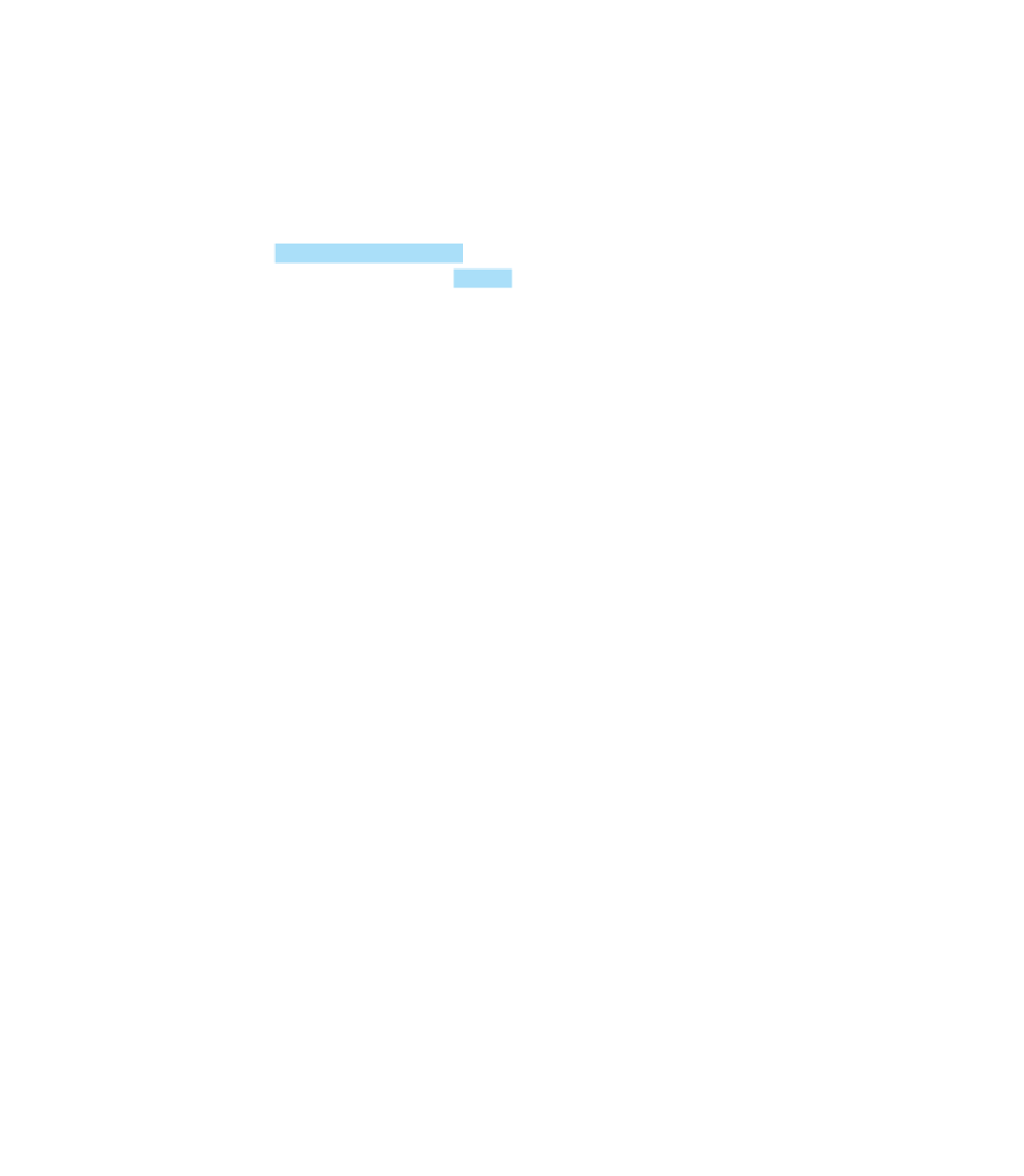
Search WWH ::

Custom Search