Java Reference
In-Depth Information
Sometimes you don't just want to draw the outline of a shape; you want to paint
the entire area with a particular color. There are variations of the
drawRect
and
drawOval
methods known as
fillRect
and
fillOval
that do exactly that, drawing
a rectangle or oval and filling it in with the current color of paint (the default is
black). Let's change two of the calls in the previous program to be “fill” operations
instead of “draw” operations:
1 // Draws and fills several shapes.
2
3
import
java.awt.*;
4
5
public class
DrawShapes2 {
6
public static void
main(String[] args) {
7
DrawingPanel panel =
new
DrawingPanel(200, 100);
8
9
Graphics g = panel.getGraphics();
10
g.fillRect(25, 50, 20, 20);
11
g.drawRect(150, 10, 40, 20);
12
g.drawOval(50, 25, 20, 20);
13
g.fillOval(150, 50, 40, 20);
14
}
15 }
Now we get the output shown in Figure 3G.5 instead.
Figure 3G.5
Output of DrawShapes2
All of the shapes and lines drawn by the preceding programs were black, and all of
the panels had a white background. These are the default colors, but you can change
the background color of the panel, and you can change the color being used by the
Graphics
object as many times as you like. To change these colors, you use the stan-
dard
Color
class, which is part of the
java.awt
package.
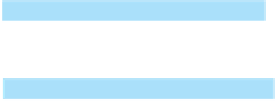
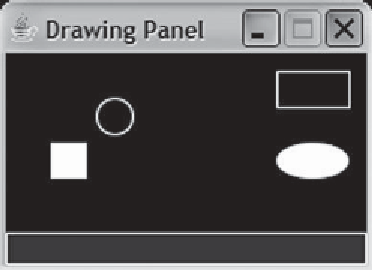

Search WWH ::

Custom Search