Java Reference
In-Depth Information
Notice that the first two values passed to
drawRect
and
drawOval
are coordi-
nates, while the next two values are a width and a height. For example, here is a short
program that draws two rectangles and two ovals:
1 // Draws several shapes.
2
3
import
java.awt.*;
4
5
public class
DrawShapes1 {
6
public static void
main(String[] args) {
7
DrawingPanel panel =
new
DrawingPanel(200, 100);
8
9
Graphics g = panel.getGraphics();
10
g.drawRect(25, 50, 20, 20);
11
g.drawRect(150, 10, 40, 20);
12
g.drawOval(50, 25, 20, 20);
13
g.drawOval(150, 50, 40, 20);
14
}
15 }
Figure 3G.4 shows the output of the program.
Figure 3G.4
Output of DrawShapes1
The first rectangle has its upper-left corner at the coordinates (25, 50). Its width
and height are each 20, so this is a square. The coordinates of its lower-right corner
would be (45, 70), or 20 more than the (x, y) coordinates of the upper-left corner. The
program also draws a rectangle with its upper-left corner at (150, 10) that has a width
of 40 and a height of 20 (wider than it is tall). The bounding rectangle of the first oval
has upper-left coordinates (50, 25) and a width and height of 20. In other words, it's a
circle. The bounding rectangle of the second oval has upper-left coordinates (150,
50), a width of 40, and a height of 20 (it's an oval that is wider than it is tall).
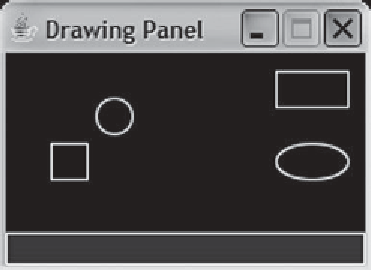
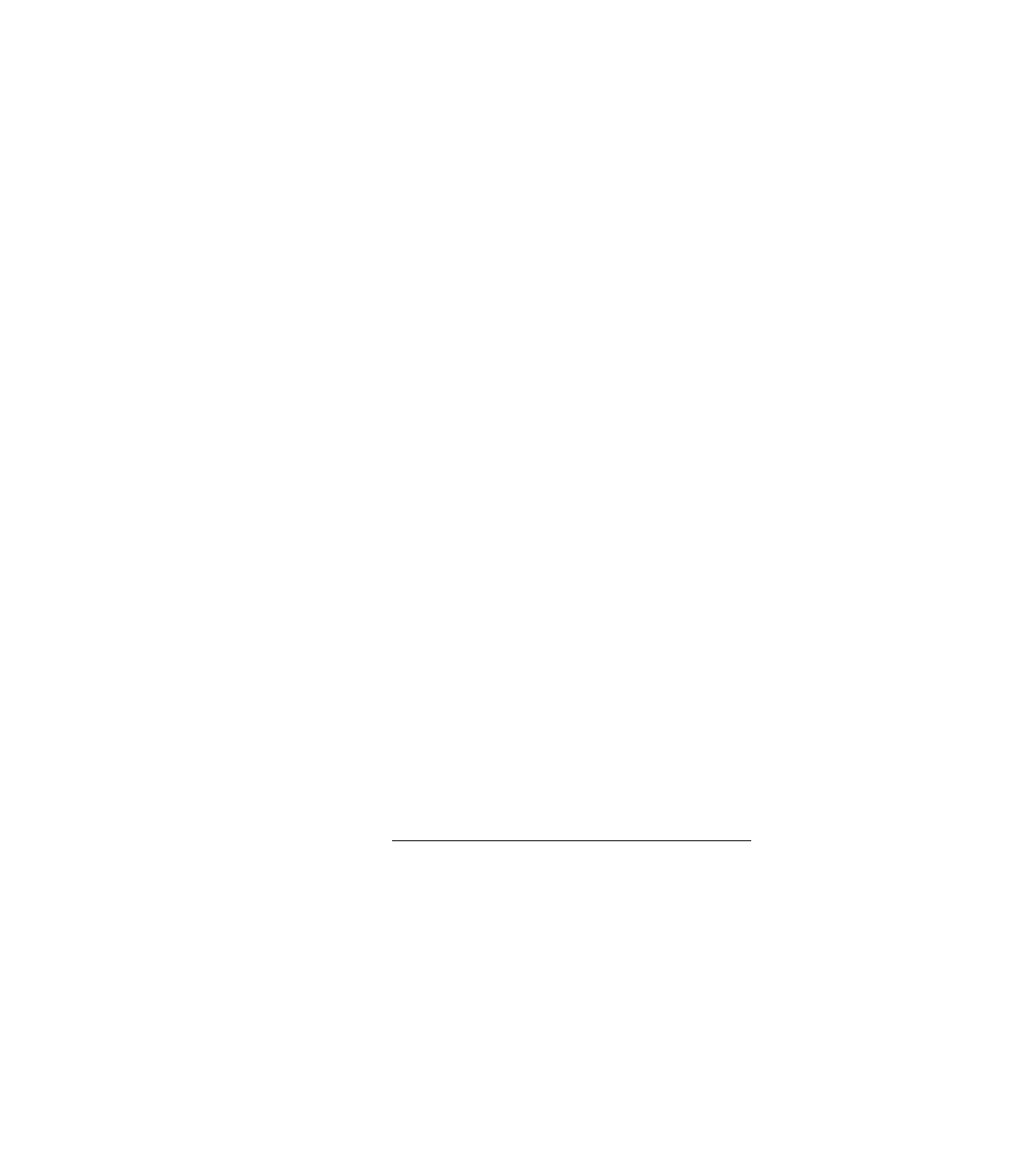
Search WWH ::

Custom Search