Graphics Reference
In-Depth Information
to
m
ik
. That would reduce variance, but it might also take longer. When we apply
this technique to solving the rendering equation, we actually
will
do some clever
things in that recursive part of the estimator. It just happens that in the matrix
model of the computation, there's no good analog for these subtleties.
Inline Exercise 31.10:
Make certain you really understand every piece of the
code in this section. Ask yourself things like, “Why is that 1
n
in the denomi-
nator?” and “Why do we multiply by
m
ik
rather than
m
ki
?” Only proceed to the
next section when you are confident of your understanding.
/
We'll now describe how to build a path tracer in analogy with the recursive version
of the linear equation solver, transforming a simple path tracer into one that's
increasingly tuned to use its sampling efficiently. The wrapper of the path tracer
is quite simple:
1
2
3
4
5
6
for each pixel
(
x
,
y
)
on the image plane:
v
=
ray from the eyepoint
E
to
(
x
,
y
)
result
=0
repeat
N
times:
result
+=
estimate of
L
(
E
,
−
v
)
pixel[
x
][
y
]=
result
/
N
;
There are variations on the wrapper. We could estimate the radiance through
various points associated with pixel
(
x
,
y
)
, and then weight the results by the mea-
surement equation to get a pixel value. We could, for a dynamic scene, estimate
the radiance at
(
x
,
y
)
for various different
times
and average them, generating
motion-blur effects, etc. But all of these have at their core the problem of estimat-
ing
L
(
E
,
Q
)
; we'll concentrate on this from now on, writing a procedure called
estimateL
to perform this task.
The first version of the estimation code is completely analogous with the
matrix equation solver: Because
L
is a sum of
L
e
and an integral, we'll use a
Monte Carlo estimator to average the two terms. We'll use the generic point
C
as
the one where we're estimating the radiance, but you should think of
C
as being
the eyepoint
E
, at least until the recursive call.
Figure 31.23 shows the relevant terms. The red arrow at the bottom is
L
e
(
P
,
−
v
v
i
v
C
P
)
(which for this scene happens to be zero, because the surface containing
P
is not
an emitter).
Figure 31.23: Names for some
points
v
and
paths
in
our
path
tracer.
// Single-sample estimate of radiance through a point
C
// in direction
1
2
3
4
5
6
7
8
9
10
11
12
v
.
define estimateL(
C
,
v
):
P
= raycast(
C
,
−
v
)
// find the surface this light came from
u
= uniform(0, 1)
if (
u
< 0.5):
return
L
e
v
)
/
0. 5
(
P
,
else:
v
i
= randsphere()
// unit vector chosen uniformly
integrand
= estimateL(
P
,
−
v
i
)
v
i
,
v
)
|
v
i
·
n
P
|
·
f
s
(
P
,
density
=
4
π
return
integrand
/ (0.5
*
density
)







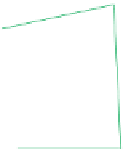

















