HTML and CSS Reference
In-Depth Information
Maria is pleased with the final version of the calendar() function. Because of the
way the function and the style sheets were designed, she can use this utility in many
other pages on the CCC Web site with only a minimal amount of recoding in the Web
documents.
Managing Program Loops and Conditional
Statements
Although you are finished with the calendar() function, you still should become familiar
with some features of program loops and conditional statements for future work with
these JavaScript structures. You'll examine three features in more detail—the
break
,
continue
, and
label
statements.
Exploring the
break
Command
Although you briefly saw how to use the
break
statement when creating a
switch
statement, the
break
statement can be used anywhere within program code. Its purpose
is to terminate any program loop or conditional statement. When a
break
statement is
encountered, control is passed to the statement immediately following it. It's most often
used to exit a program loop before the stopping condition is met. For example, consider
a loop that examines an array for the presence or absence of a particular value such as a
customer ID number or name. The code for the loop might look as follows:
for (var i = 0; i < names.length; i++) {
if (names[i] == “Valdez”) {
document.write(“Valdez is in the list”);
}
}
What would happen if the array had tens of thousands of entries? It would be time
consuming to keep examining the array once
Valdez
has been encountered. To address
this, the following
for
loop breaks off when it encounters the
Valdez
text string, keeping
the browser from needlessly examining the rest of the array:
for (var i = 0; i< names.length; i++) {
if (names[i] == “Valdez”) {
document.write(“Valdez is in the list”);
break; // stop processing the for loop
}
}
Exploring the
continue
Command
The
continue
statement
is similar to the
break
statement except that instead of stopping
the program loop altogether, the
continue
statement stops processing the commands in
the current iteration of the loop and continues on to the next iteration. For example, your
program might employ the following
for
loop to add the values from an array:
var total = 0;
for (var i = 0; i < data.length; i++) {
total += data[i];
}
Each time through the loop, the value of the current entry in the data array is added
to the
total
variable. When the
for
loop is finished, the
total
variable is equal to the
sum of the values in the data array. However, what would happen if this were a sparse
array containing several empty entries? In that case, when a browser encountered a
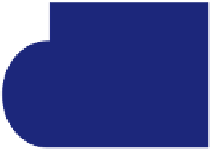