HTML and CSS Reference
In-Depth Information
Like
if
statements,
if else
statements can be nested as in the following code, which
chooses between three possible text strings to write to a document:
if (day == “Friday”) document.write(“Thank goodness it's Friday”)
else {
if (day == “Monday”) document.write(“Blue Monday”)
else document.write(“Today is “ + day);
}
Some programmers advocate always using curly braces even if the command block
contains only a single command. This practice visually separates one
else
clause from
another. Also, when reading through nested statements, it can be helpful to remember
that an
else
clause usually pairs with the nearest preceding
if
statement.
To make it easier to
interpret nested
if
state-
ments, always indent your
code, lining up all of the
commands for one set of
nested statements.
Using Multiple
else
if
Statements
For more complex scripts, you might need to choose from several alternatives. In these
cases, you can specify multiple
else
clauses, each with its own
if
statement. This is not
a new type of conditional structure, but rather a way of taking advantage of the syntax
rules inherent in the
if else
statement. The general structure for choosing from several
alternatives is
if (
condition 1
) {
first command block
} else if (
condition 2
) {
second command block
} else if (
condition 3
) {
third command block
...
} else {
default command block
}
where
condition 1
,
condition 2
,
condition 3
, and so on are the different condi-
tions to be tested. This construction always should include a final
else
clause that is run
by default if none of the preceding conditional expressions returns the value
true
.
When a browser runs a series of statements like this one, it stops examining the remain-
ing
else
clauses when it encounters the first true
else
clause because there no longer
is an
else
condition to investigate. The structure in the following example employs
multiple
else if
conditions:
To simplify code, keep
your nesting of multiple
if
statements to three or
less, if possible. For more
conditions, use the
case
/
switch
structure.
if (day == “Friday”) {
document.write(“Thank goodness it's Friday”);
} else if (day == “Monday”) {
document.write(“Blue Monday”);
} else if (day == “Saturday”) {
document.write(“Sleep in today”);
} else {
document.write(“Today is “ + day);
}


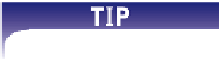
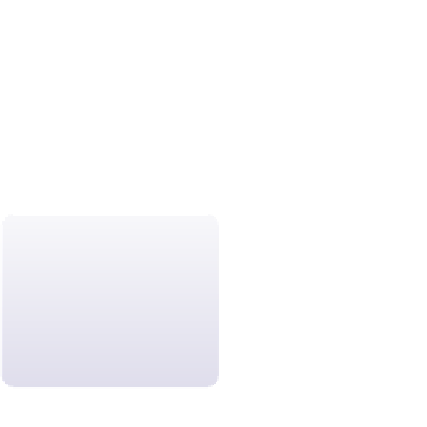
