HTML and CSS Reference
In-Depth Information
which is a general-purpose method for extracting and inserting array items, where
start
is the index of the array item at which to start extracting items from the array, and
size
is the number of items to extract. If no
size
value is specified, items are removed up
through the end of the array. The following statement demonstrates how to splice the
summer months from the
monthName
array:
summerMonths = monthName.splice(5, 3);
The important difference between the
slice()
and
splice()
methods is that the
splice()
method removes the selected items from the original array in addition to
extracting them. You should not use the
splice()
method if you want the original array
unaffected.
You also can use the
splice()
method to insert new items within an array by apply-
ing the command
array
.splice(
start
,
size
,
values
)
where
values
is a comma-separated list of new values to replace the old values in the
array. For example, to insert the first three month names with their abbreviations into the
monthName
array, you could apply the following
splice()
method:
monthName.splice(0, 3, “Jan”, “Feb”, “Mar”);
Using the
splice()
method in this fashion automatically increases the length of
the array.
Using Arrays as Data Stacks
Arrays can be used to store information in a data structure known as a
stack
in which
new items are added to the top of the stack—or to the end of the array—much like a per-
son clearing a dinner table adds dishes to the top of a stack of dirty plates. A stack data
structure employs the
last-in first-out
(
liFO
) principle in which the last items added to
the stack are the first ones removed. You encounter stack data structures when using the
Undo feature of some software applications, in which the last command you performed
is the first command that is undone.
JavaScript supports several methods to allow you to work with a stack of array
items. For example, the
push()
method appends new items to the end of an array. It has
the syntax
array
.push(
values
)
where
values
is a comma-separated list of values to be appended to the end of the
array. To remove—or
unstack
—the last item, you apply the
pop()
method, as follows:
array
.pop()
The following set of commands demonstrates how to use the
push()
and
pop()
methods to employ the LIFO principle by adding and then removing items from a
data stack:
var x = [“a”, “b”, “c”];
x.push(“d”, “e”); // x = [“a”, “b”, “c”, “d”, “e”]
x.pop(); // x = [“a”, “b”, “c”, “d”]
x.pop(); // x = [“a”, “b”, “c”]
In this code, the
push()
method adds two items to the end of the array, and then the
pop()
method removes those last items one at a time.
Similar to a stack is a
queue
, which employs the
first-in first-out
(
FiFO
) principle in
which the first item added to the data list is the first removed. You see this principle in
action in a line of people waiting to be served. For array data that should be treated as a
queue, you use the
shift()
method, which is similar to the
pop()
method except that it
removes the first array item, not the last. JavaScript also supports the
unshift()
method,
which inserts new items at the front of the array.
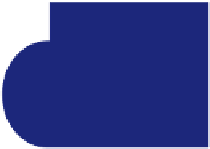