HTML and CSS Reference
In-Depth Information
After applying the
sort()
method with this compare function, the values in the
x
array would be sorted in the following order—
3
,
24
,
45
, and then
1234
. To sort the
array in decreasing numeric order, you'd need a compare function that subtracts the first
parameter from the second—returning the difference
b ‑ a
rather than
a ‑ b
. The compare
function would look like the following:
You also can sort an array
in descending order
by sorting it and then
applying the
reverse()
method to the sorted array.
function numSortDescending(a, b) {
return b-a;
}
Performing a Random Shuffle
For some applications, you'll want to randomly rearrange the contents of an array. For
example, you might be writing a program to simulate a randomly shuffled deck of cards.
You can shuffle an array using the same
sort()
method you use to place the array in
a defined order; however, to place the items in a random order, you use a compare
function that randomly returns a positive, negative, or 0 value. The following compare
function employs a simple approach to this problem:
function randOrder(){
return 0.5 - Math.random();
}
The following code demonstrates how this compare function could be used to randomly
shuffle an array of poker cards:
var pokerDeck = new Array(52);
pokerDeck[0] = “2H”;
pokerDeck[1] = “3H”;
...
pokerDeck.sort(randOrder)
After running this command, the contents of the
pokerDeck
array would be placed in
random order. To reshuffle the array, you'd simply rerun the
sort()
method with the
randOrder() function.
Extracting and Inserting Array Items
In some scripts, you might want to extract a section of an array, known as a
subarray
.
One way to create a subarray is with the
slice()
method
array
.slice(
start
,
stop
)
where
start
is the index of the array item at which the slicing starts and
stop
is the
index before which the slicing ends. The
stop
value is optional; if it is omitted, the array
is sliced to its end. The original contents of the array are unaffected after slicing, but the
extracted items can be stored in another array. For example, the following command
slices the
monthName
array, extracting only three summer months—June, July, August—
and storing them in the
summerMonths
array:
summerMonths = monthName.slice(5, 8);
Remember that arrays start with the index value 0, so the sixth month of the year (June)
has an index value of 5 and the ninth month of the year (September) has an index value
of 8.
Related to the
slice()
method is the
splice()
method
array
.splice(
start
,
size
)

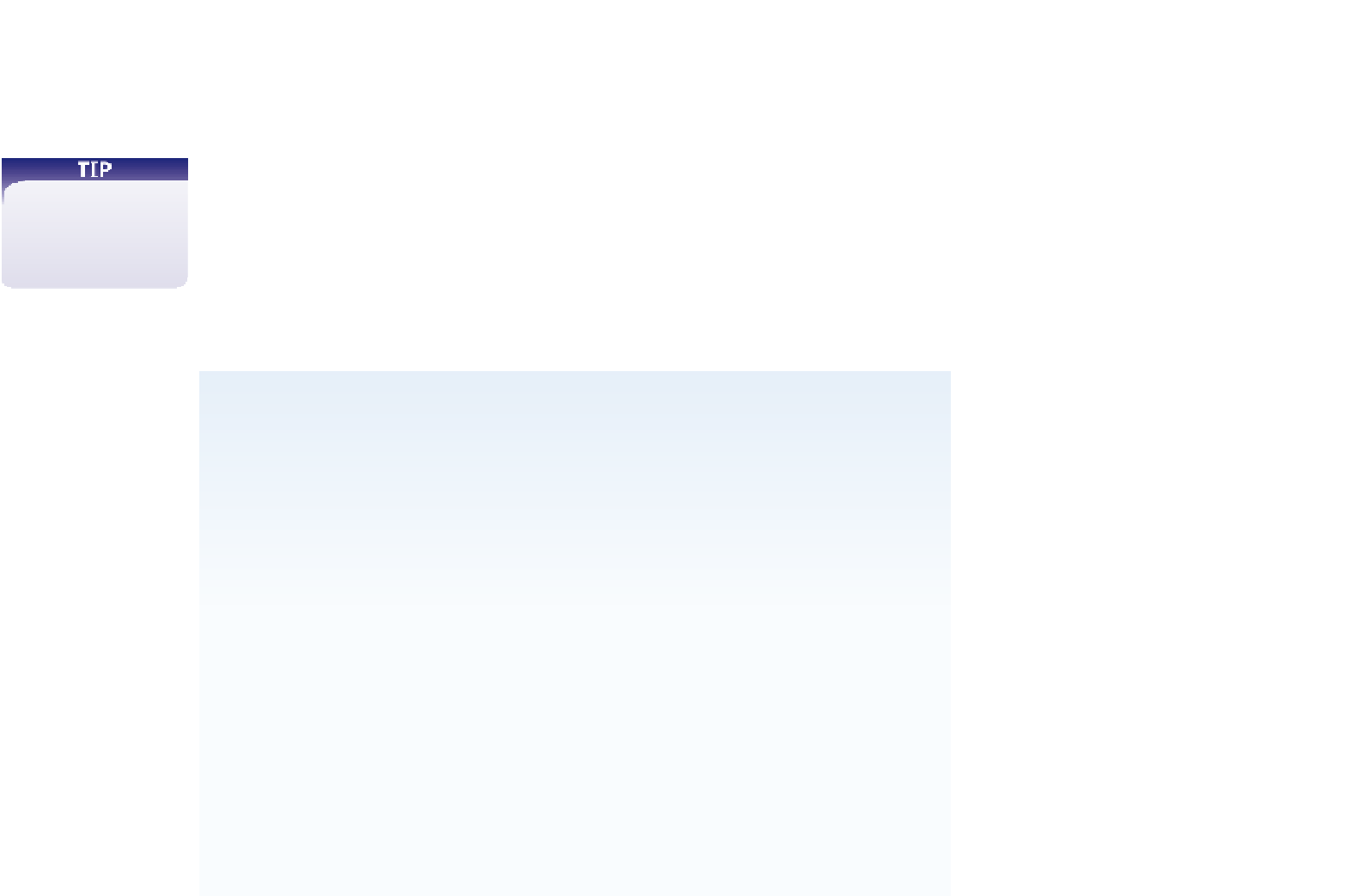

