Graphics Reference
In-Depth Information
Note that the table above still applies to convert the values from the noise
texture into something useful. The only difference is that a 2D noise texture is
indexed by a
vec2
while the 3D noise texture is indexed by a
vec3
. But both
return a
vec4
.
Using Noise with the Built-In GLSL Functions
If you are using a system where the GLSL built-in noise functions work, here
is how you would use them. There are four built-in GLSL noise functions:
noise1( )
,
noise2( )
,
noise3( )
, and
noise4( )
. They each return a
float
,
vec2
,
vec3
, and
vec4
, respectively, whose values are between −1. and 1. They
each can accept as their single argument
any
of those four types of inputs,
depending on how you want to index into the noise. Thus, where we might
have said
uniform sampler3D Noise3;
. . .
vec3 stp = ...
vec4 nv = texture( Noise3, stp );
float sum = nv.r + nv.g + nv.b + nv.a;
sum = ( sum - 2. );
for
glman
, using the GLSL built-in noise functions we could accomplish the
same thing by saying
float sum = 0.;
float size = 1.;
for( int i = 0; i < 4; i++ )
{
sum += noise1( size*stp ) / size;
size *= 2.;
}
Turbulence
Turbulence
is a special effect created from a noise function.
2
It can give you
a “sharper” appearance than a simple noise function. Turbulence is created
by taking the absolute value of each noise octave about the midpoint before
summing them. It is simple to produce if you have a good noise function.
Introducing the absolute value operation can add sharp changes in the func-
2.
Turbulence is the term used in computer graphics for what we are about to describe. Note, however,
this is not the same as fluid turbulence. The overloading of the term is unfortunate.
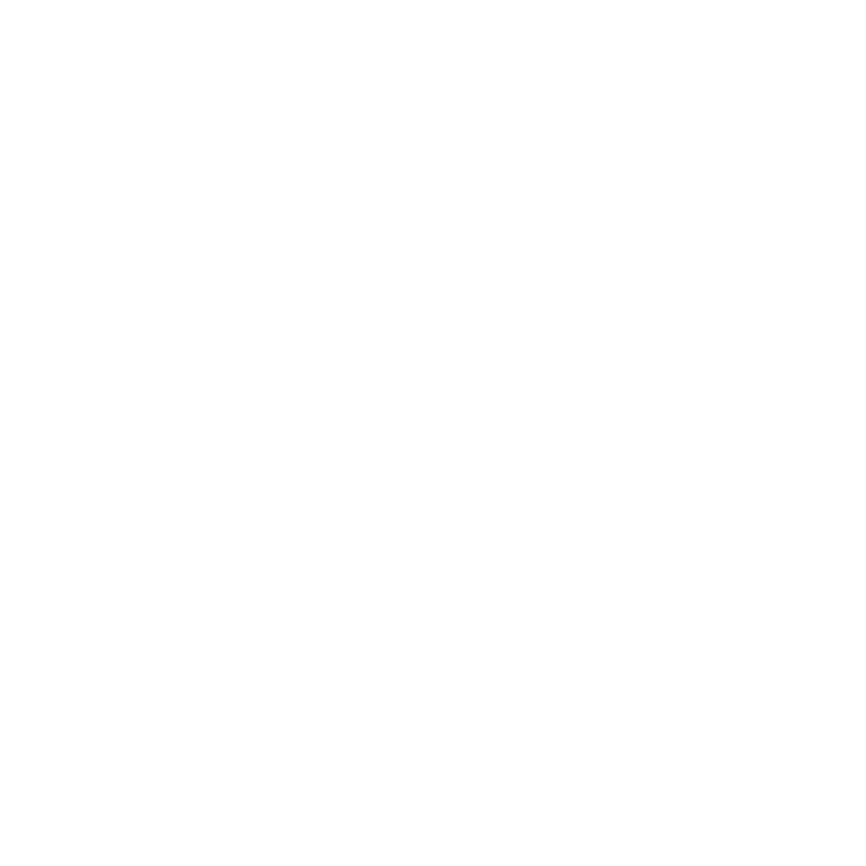

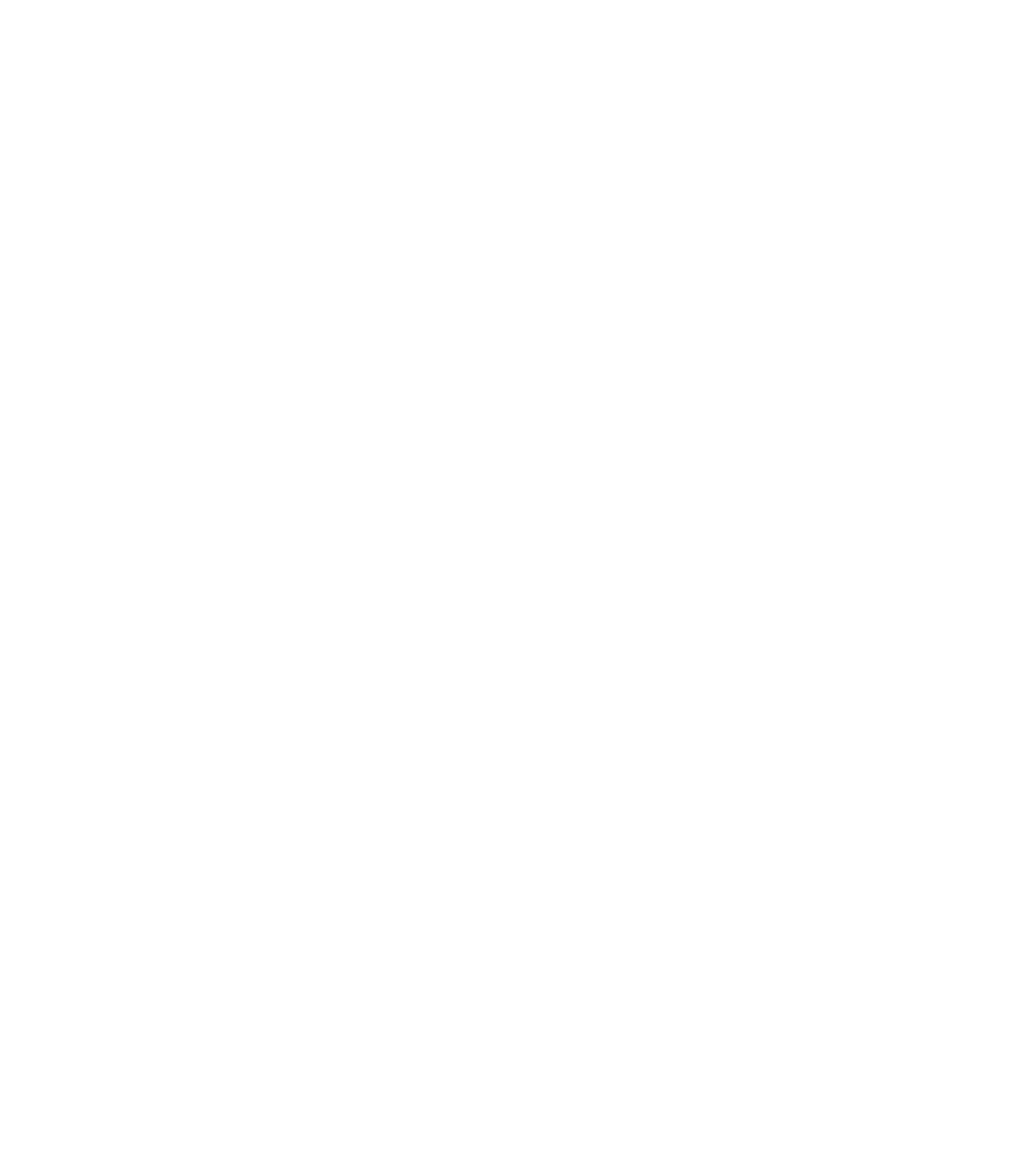
