Graphics Reference
In-Depth Information
vec3 ADSLightModel( in vec3 myNormal, in vec4 myPosition )
{
...
}
void main( )
{
float dfdx = 2.*0.3*vMyXY.x*cos(vMyXY.x*vMyXY.x +
vMyXY.y*vMyXY.y);
float dfdy = 2.*0.3*vMyXY.y*cos(vMyXY.x*vMyXY.x +
vMyXY.y*vMyXY.y);
vec3 xtangent = vec3( 1., 0., dfdx );
vec3 ytangent = vec3( 0., 1., dfdy );
vec3 thisNormal = normalize( cross( xtangent, ytangent ) );
vec3 color = ADSLightModel( thisNormal, vPos);
fFragColor = vec4( color, 1. );
}
As a quick aside, the code above was writen to correspond closely to the
equations that it represents. But one could be a litle more cryptic, and a litle
more efficient, by coding the expression
vMyXY.x*vMyXY.x + vMyXY.y*vMyXY.y
as
dot( vMyXY.xy, vMyXY.xy )
Anisotropic Shading
The examples of shading above have all been
isotropic
, that is, the light reflected
from the surface at a point has been assumed to be the same in all directions.
However, this is not true of all surfaces. Anisotropic shading models light
that is reflected differently in different directions [19]. This is a property of a
surface, and examples include hair (see the left image in Figure 8.8), brushed
metallic surfaces, scored surfaces, or surfaces made up of oriented threads. A
fur-covered surface can also be treated as an isotropic surface.
Anisotropic shading does not simply use the usual angles, the angle from
the normal of the diffuse reflection and the angle from the reflected light in the
specular reflection. Instead, it computes the angle with which light is reflected
from a surface. This may be a direct calculation, as it is in the example below,
or it may use a function called the
bidirectional reflection distribution function
(or
BRDF
) to determine how much light is reflected toward the eye. This function
typically depends on both the latitude Θ and longitude Φ angle of the eye and

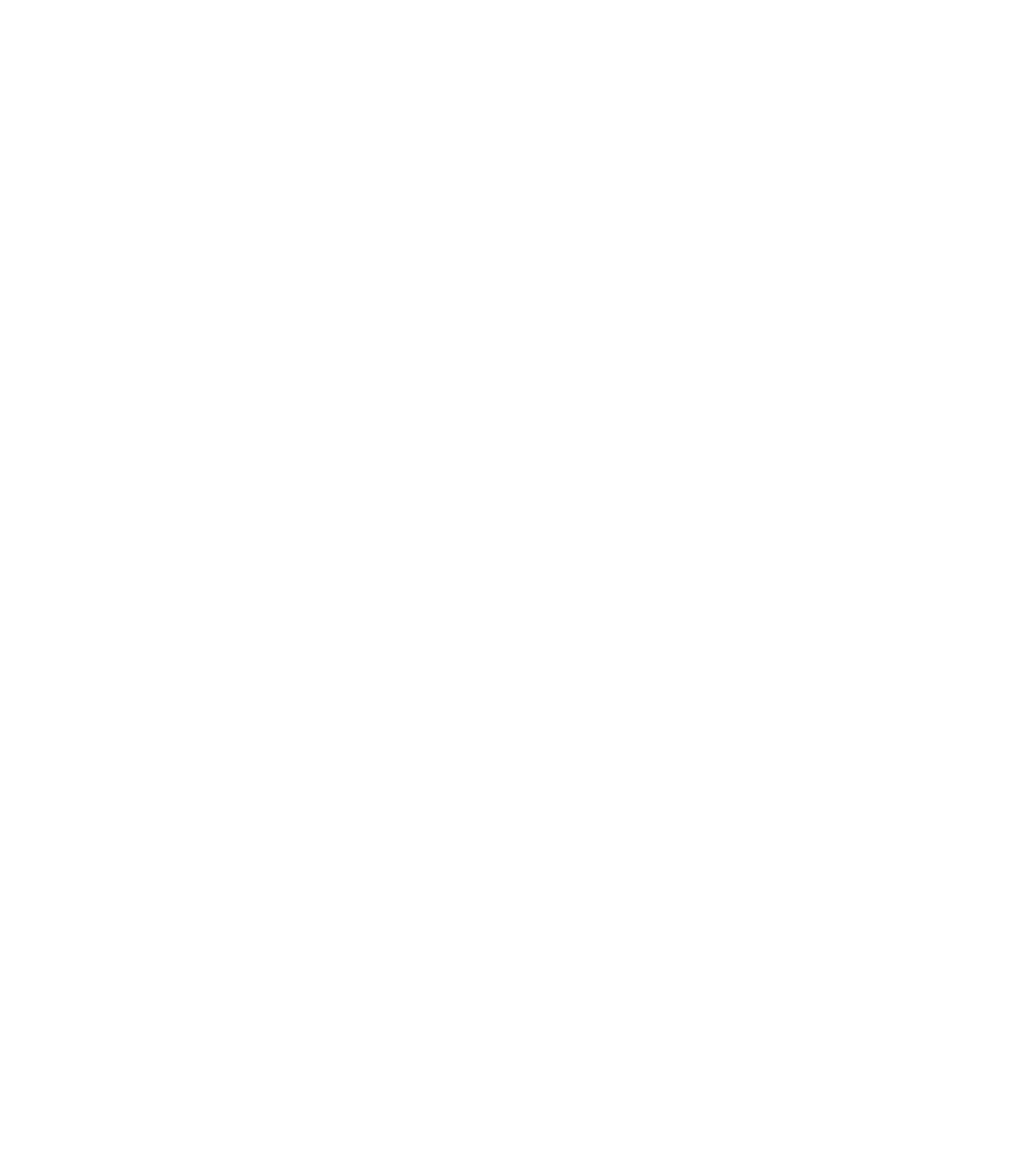