Java Reference
In-Depth Information
Table 3-6 displays the general form of an assignment statement. Note that the
assignment operator, which looks like an equal sign, never is used to represent
equality in Java. The equality operator (= =) will be discussed later in the
chapter.
Table 3-6
Assignment Statements
General form:
1. identifier = value; //simple assignment
2. dataType identifier = value; //declaration and assignment
3. identifier = formula; //assigning the result of a formula
4. identifier = differentIdentifier; //copying data between
identifiers
5. identifier = object.method(); //assigning the result of a
method call
6. identifier = identifier + value; //accumulator
Purpose:
To assign a value to a storage location
Examples:
1. userAge = 21;
2. boolean flag = false;
3. totalCharge = sales + tax;
4. newLocation = oldData;
5. inches = Integer.parseInt(height);
6. counter = counter + 1;
Programmers sometimes declare their data and assign it at the same time, as
shown in example 2. When using this type of assignment statement, the literal
data must match the data type of the identifier. When a formula is used for
assignment, as in example 3, the formula is placed on the right side of the
assignment operator and the location to store the result is on the left. In example
4, the assignment is a reference to another storage location; therefore, its data is
copied from one location to another. In example 5, the assignment is the result
of a method call.
Because Java evaluates the code on the right side of the assignment operator
before assigning the result to the left side, a special case may arise in which a
value is manipulated and then stored back in the same storage location, as
shown in example 6. For instance, if you want to track the number of hits on a
Web page, you might write code to add 1 to the current counter value each time
the page is downloaded from the Web server. The code then stores the updated
counter value back in the original counter storage location. The result is an accu-
mulated total called an
accumulator,
or
counter
. Every time Java executes the
line of code, a value of 1 will be added to the current counter total. You will
learn more about accumulators and counters in a later chapter.
The step on the next page enters code to prompt the user, accept input,
and then parse the String responses. If your code runs over to two lines in the
TextPad coding window, let the text wrap to the next line; do not press the
ENTER
key to split the code into two lines. Even if the code wraps to two or more lines
in the coding window, Java looks for the semicolon (;) to indicate the end of the
line.
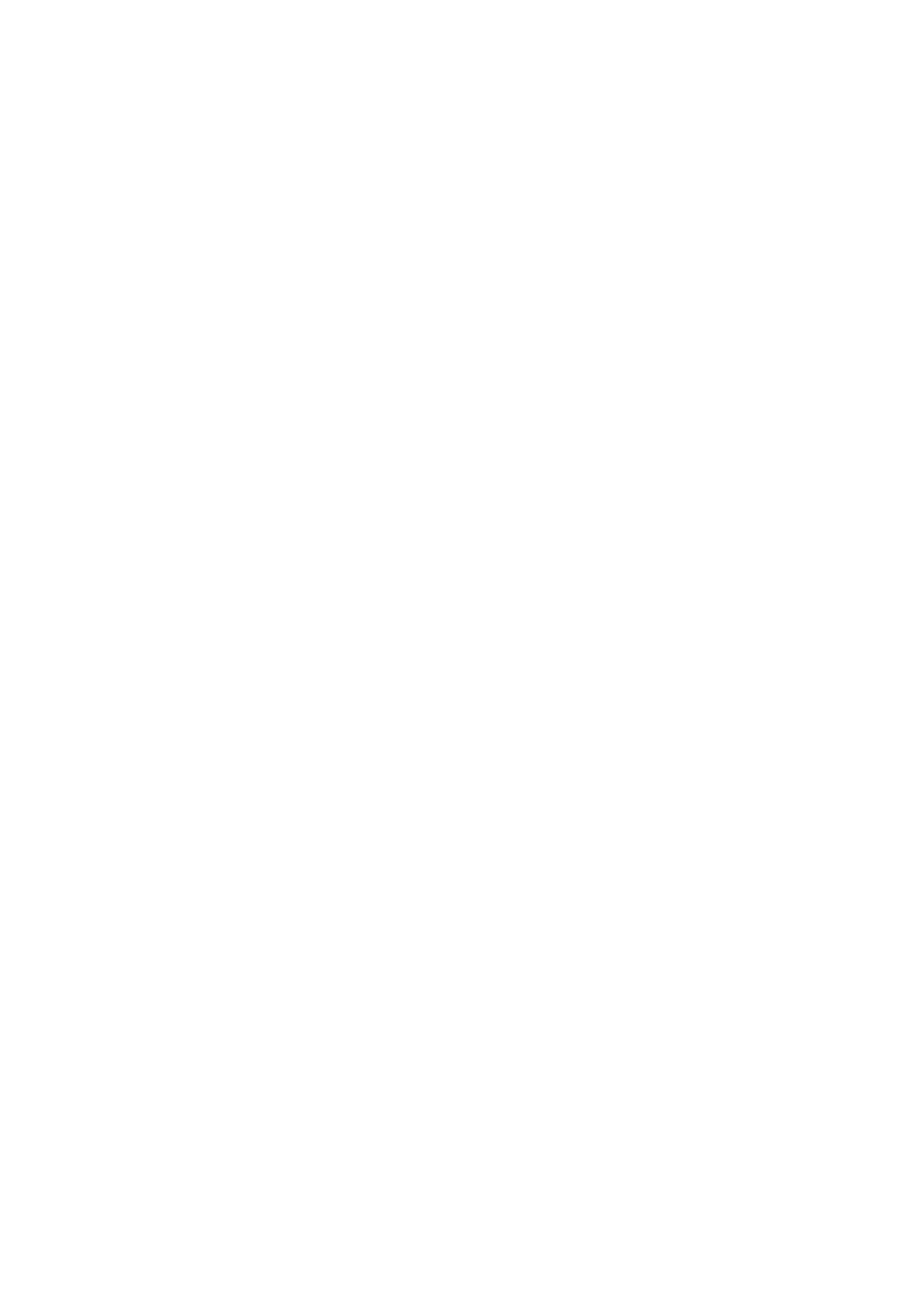
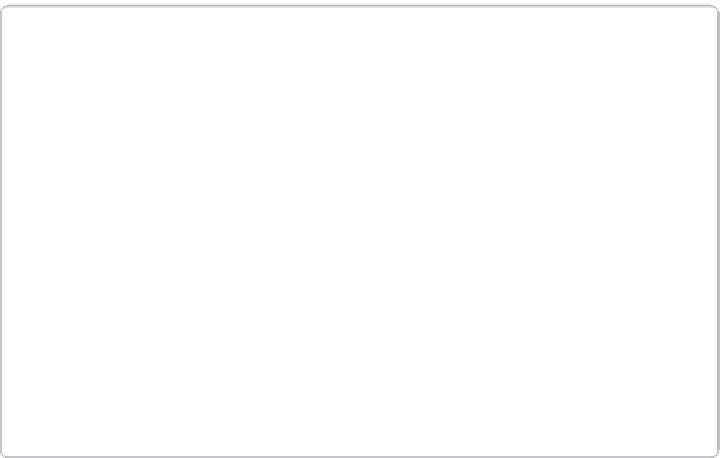



Search WWH ::

Custom Search