Java Reference
In-Depth Information
The buffer stores input data one character at a time from the keyboard and
then delivers a stream of characters to the program wrapped as a String object.
Data read from the buffer with the method, readLine(), thus is a string of char-
acters; therefore, it must be declared as a String object. A String is appropriate
for data such as a person's name, but Java cannot perform mathematical opera-
tions on a String. Consequently, any input data that a programmer plans to use
in formulas must be converted from a String to a numeric data type.
In the java.lang package, each primitive data type may use its associated
wrapper class
to provide a way to help primitive data types, such as ints and
doubles, conform to their Object class counterparts, such as Strings. Unlike the
String class of objects, primitive data types in Java are not objects. Each wrapper
class wraps, or packages, the primitive data type value so that it is treated as an
object. The Integer class of objects, for example, wraps an int value in an object
so that the program can manipulate it as though it were an object. In addition,
the Integer class provides several methods for converting a String to an int data
type and vice versa.
One of the methods associated with the wrapper classes is the
parse()
method
, which allows programmers to convert Strings to a numeric data type.
The parse() method is unique to each of its data types. The parseInt() method,
as shown in lines 27 and 30 of Figure 3-14 on the previous page, belongs to the
Integer class of objects, while the parseDouble() method belongs to the Double
class of objects. The basic syntax of any parse() method is to list the object class
followed by a period, followed by the specific method name, such as parseInt()
or parseDouble(). The String variable to be converted is placed inside the
method's parentheses.
For each of the parse() methods, the end result is the same: a String is con-
verted to a number. The difference is the data type of the converted number and
the object name listed before the parse method.
Note that lines 27 and 30 are indented below the lines of code that include
the println() and print() methods used to display input prompts to the user.
Typically, the lines of code that accept and manipulate data in response to user
input are indented to show their relationship to the prompt.
J
ava 2
J
ava 2
v
5
.
0
JAVA UPDATE
v
5
.
0
A new class of Scan-
ner objects used for
data entry is avail-
able in version 5.0. A
Scanner object can
accept user input
from System.in and
then call predefined
methods to extract
the data. Methods
such as
nextInt()
,
nextDouble(), and
nextBoolean() allow
direct assignment to
the corresponding
variable without
parsing.
The
Scanner
class is
part of the java.util
package that must
be imported in pro-
grams that use
Scanner.
If you want to
amend the BodyMass
program to incorpo-
rate Scanner, replace
lines 11, 20, 27, and
30, then comment
out lines 26 and 29
as shown in the fol-
lowing code.
Assignment Statements
The parseInt() methods in lines 27 and 30 assign the parsed value on the
right of the
assignment operator
(=) to the String variable on the left. An
assignment statement
is a line of code beginning with a location, followed by
the assignment operator (=), followed by the new data, method, or formula.
11
import
java.util.*;
20 Scanner scannerIn=
new
Scanner(
System
.in);
26
//height = dataIn.readLine();
27 inches = scannerIn.nextInt
()
;
29
//weight = dataIn.readLine();
30 pounds = scannerIn.nextInt
()
;
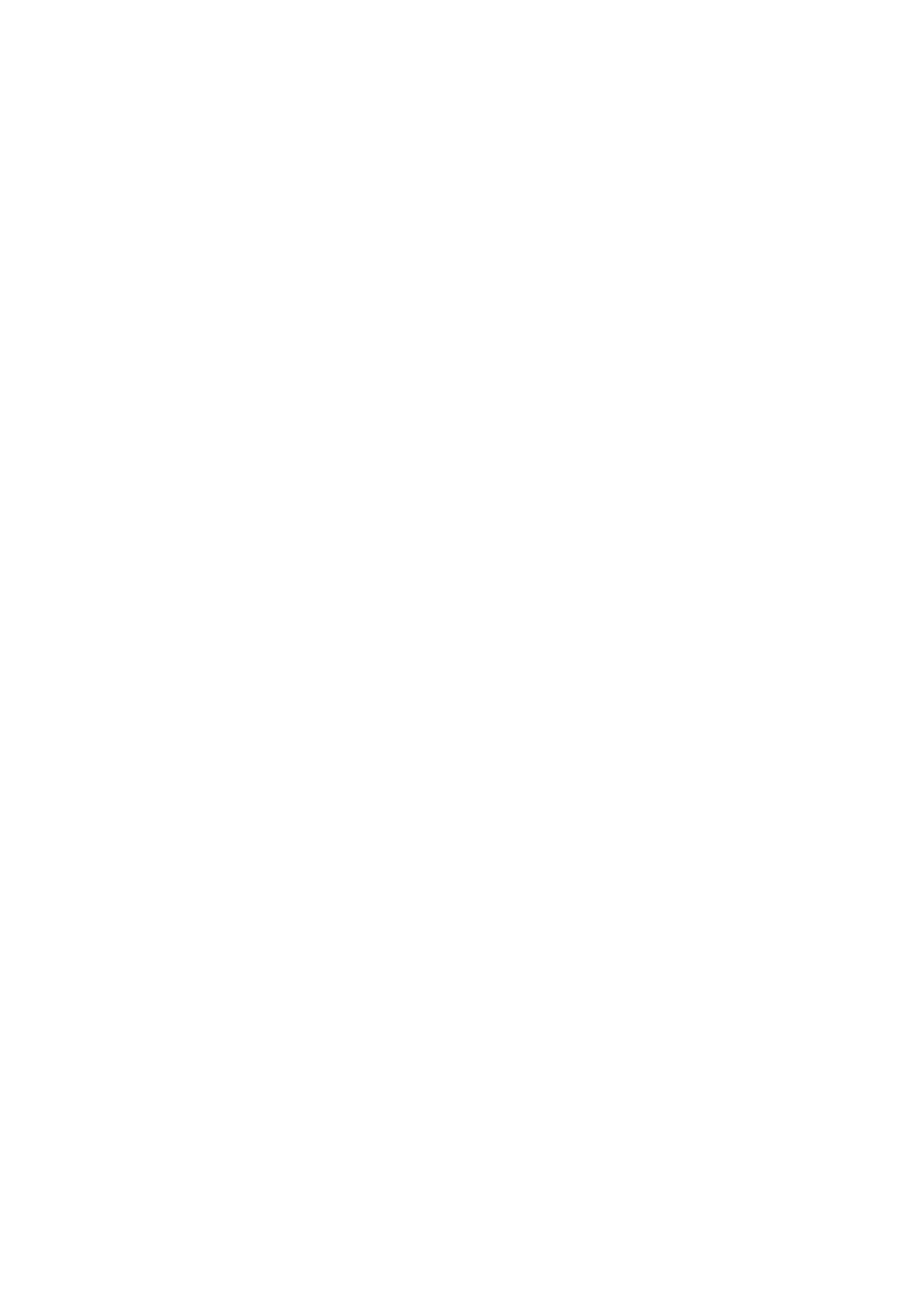

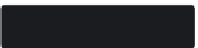





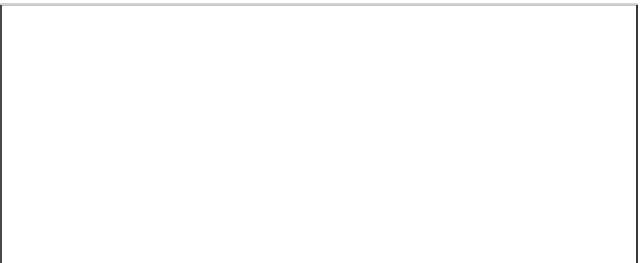


Search WWH ::

Custom Search