Java Reference
In-Depth Information
figure 2.11
Simple program to
illustrate exceptions
1
import java.util.Scanner;
2
3
public class DivideByTwo
4
{
5
public static void main( String [ ] args )
6
{
7
Scanner in = new Scanner( System.in );
8
int x;
9
10
System.out.println( "Enter an integer: " );
11
try
12
{
13
String oneLine = in.nextLine( );
14
x = Integer.parseInt( oneLine );
15
System.out.println( "Half of x is " + ( x / 2 ) );
16
}
17
catch( NumberFormatException e )
18
{ System.out.println( e ); }
19
}
20
}
1.
If the
try
block executes without exception, control passes to
the
finally
block. This is true even if the
try
block exits prior to
the last statement via a
return
,
break
, or
continue
.
2.
If an uncaught exception is encountered inside the
try
block,
control passes to the
finally
block. Then, after executing the
finally
block, the exception propagates.
3.
If a caught exception is encountered in the
try
block, control
passes to the appropriate
catch
block. Then, after executing the
catch
block, the
finally
block is executed.
2.5.3
common exceptions
There are several types of standard exceptions in Java. The
standard run-
time exceptions
include events such as integer divide-by-zero and illegal
array access. Since these events can happen virtually anywhere, it would
be overly burdensome to require exception handlers for them. If a
catch
block is provided, these exceptions behave like any other exception. If a
catch
block is not provided for a standard exception, and a standard excep-
tion is thrown, then it propagates as usual, possibly past
main
. In this case,
it causes an abnormal program termination, with an error message. Some
of the common standard runtime exceptions are shown in Figure 2.12.
Generally speaking, these are programming errors and should not be
Runtime excep-
tions
do not have to
be handled.
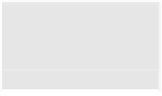


Search WWH ::

Custom Search