Java Reference
In-Depth Information
Arrays are indexed
starting at zero. The
number of items
stored in the array is
obtained by the
length
field. No
parentheses are
used.
array
a
can always be obtained by
a.length
. Note that there are no parentheses.
A typical array loop would use
for( int i = 0; i < a.length; i++ )
2.4.1
declaration, assignment, and methods
An array is an object, so when the array declaration
int [ ] array1;
is given, no memory is yet allocated to store the array.
array1
is simply a name
(reference) for an array, and at this point is
null
. To have 100
int
s, for exam-
ple, we use
new
:
To allocate an array,
use
new
.
array1 = new int [ 100 ];
Now
array1
references an array of 100
int
s.
There are other ways to declare arrays. For instance, in some contexts
int [ ] array2 = new int [ 100 ];
is acceptable. Also, initializer lists can be used, as in C or C++, to specify ini-
tial values. In the next example, an array of four
int
s is allocated and then ref-
erenced by
array3
.
int [ ] array3 = { 3, 4, 10, 6 };
The brackets can go either before or after the array name. Placing them before
makes it easier to see that the name is an array type, so that is the style used
here. Declaring an array of reference types (rather than primitive types) uses
the same syntax. Note, however, that when we allocate an array of reference
types, each reference initially stores a
null
reference. Each also must be set to
reference a constructed object. For instance, an array of five buttons is con-
structed as
Button [ ] arrayOfButtons;
arrayOfButtons = new Button [ 5 ];
for( int i = 0; i < arrayOfButtons.length; i++ )
arrayOfButtons[ i ] = new Button( );
Figure 2.4 illustrates the use of arrays in Java. The program in Figure 2.4
repeatedly chooses numbers between 1 and 100, inclusive. The output is the
number of times that each number has occurred. The
import
directive at line 1
will be discussed in Section 3.8.1.





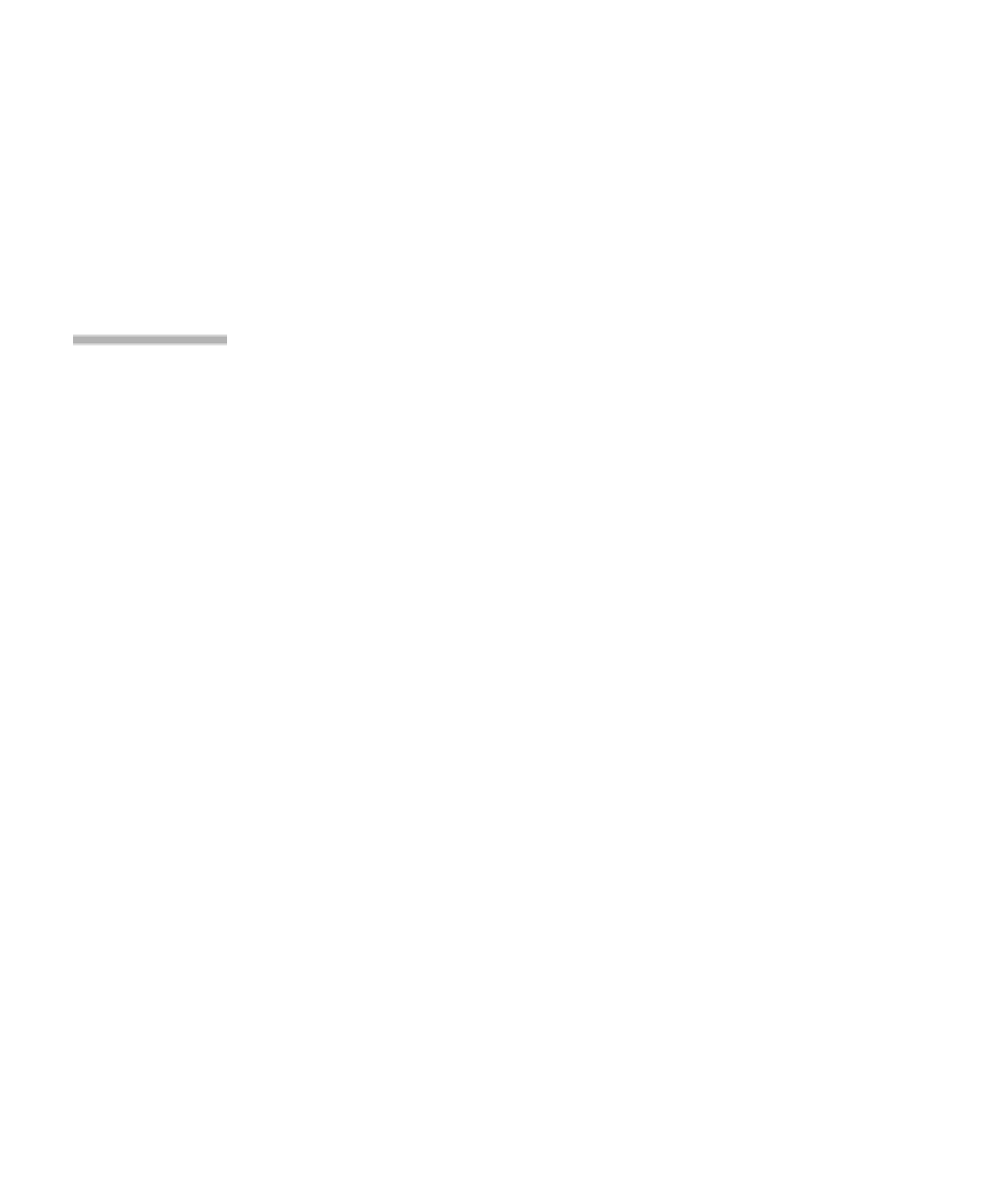
Search WWH ::

Custom Search