Java Reference
In-Depth Information
String greeting = "hello";
int len = greeting.length( ); // len is 5
char ch = greeting.charAt( 1 ); // ch is 'e'
String sub = greeting.substring( 2, 4 ); // sub is "ll"
2.3.5
converting other types to strings
String concatenation provides a lazy way to convert any primitive to a
String
.
For instance,
""+45.3
returns the newly constructed
String "45.3"
. There are
also methods to do this directly.
The method
toString
can be used to convert any primitive type to a
String
. As an example,
Integer.toString(45)
returns a reference to the newly
constructed
String "45"
. All reference types also provide an implementation
of
toString
of varying quality. In fact, when operator
+
has only one
String
argument, the non
String
argument is converted to a
String
by automatically
applying an appropriate
toString
. For the integer types, an alternative form of
Integer.toString
allows the specification of a radix. Thus
toString
converts
primitive types (and
objects) to
String
s.
System.out.println( "55 in base 2: " + Integer.toString( 55, 2 ) );
prints out the binary representation of 55.
The
int
value that is represented by a
String
can be obtained by calling
the method
Integer.parseInt
. This method generates an exception if the
String
does not represent an
int
. Exceptions are discussed in Section 2.5.
Similar ideas work for a
double
s. Here are some examples:
int x = Integer.parseInt( "75" );
double y = Double.parseDouble( "3.14" );
arrays
2.4
An
aggregate
is a collection of entities stored in one unit. An
array
is the
basic mechanism for storing a collection of identically typed entities. In Java
the array is not a primitive type. Instead, it behaves very much like an object.
Thus many of the rules for objects also apply to arrays.
An
array
stores a
collection of identi-
cally typed entities.
Each entity in the array can be accessed via the
array indexing operator
[]
. We say that the
[]
operator
indexes
the array, meaning that it specifies
which object is to be accessed. Unlike C and C++, bounds-checking is per-
formed automatically.
In Java, arrays are always indexed starting at zero. Thus an array
a
of three
items stores
a[0]
,
a[1]
, and
a[2]
. The number of items that can be stored in an
The
array indexing
operator
[]
pro-
vides access to any
object in the array.
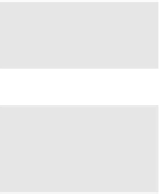






Search WWH ::

Custom Search