Java Reference
In-Depth Information
1
package weiss.util;
2
3
/**
4
* AbstractCollection provides default implementations for
5
* some of the easy methods in the Collection interface.
6
*/
7
public abstract class AbstractCollection<AnyType> implements Collection<AnyType>
8
{
9
/**
10
* Tests if this collection is empty.
11
* @return true if the size of this collection is zero.
12
*/
13
public boolean isEmpty( )
14
{
15
return size( ) == 0;
16
}
17
18
/**
19
* Change the size of this collection to zero.
20
*/
21
public void clear( )
22
{
23
Iterator<AnyType> itr = iterator( );
24
while( itr.hasNext( ) )
25
{
26
itr.next( );
27
itr.remove( );
28
}
29
}
30
31
/**
32
* Adds x to this collections.
33
* This default implementation always throws an exception.
34
* @param x the item to add.
35
* @throws UnsupportedOperationException always.
36
*/
37
public boolean add( AnyType x )
38
{
39
throw new UnsupportedOperationException( );
40
}
figure 15.10
Sample implementation of
AbstractCollection
(part 1)
of
contains
and
remove
. Both implementations use a sequential search, so they
are not efficient, and need to be overridden by respectable implementations of
the
Set
interface.
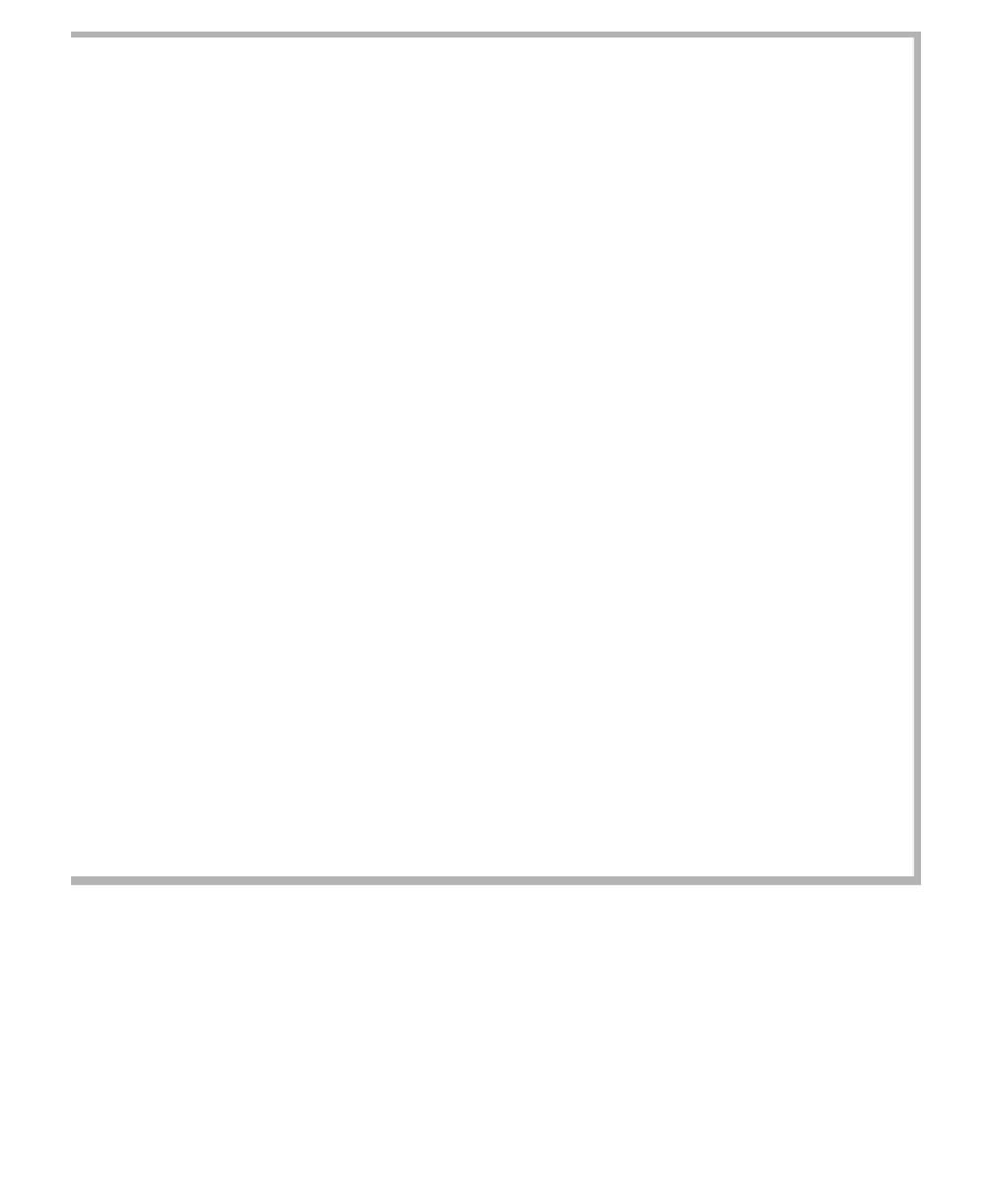
Search WWH ::

Custom Search