Java Reference
In-Depth Information
classes may not have static fields or methods, except for static final fields.
Inner classes may have nested classes or interfaces. Finally, when you com-
pile the above example, you will see that the compiler generates a class file
named
MyContainer$LocalIterator.class
, which would have to be included in
any distribution to clients. In other words, each inner and nested class is a
class and has a corresponding class file. Anonymous classes use numbers
instead of names.
the
AbstractCollection
class
15.3
Before we implement the
ArrayList
class, observe that some of the methods in the
Collection
interface can be easily implemented in terms of others. For instance,
isEmpty
is easily implemented by checking if the size is 0. Rather than doing so in
ArrayList
,
LinkedList
, and all the other concrete implementations, it would be
preferable to do this once and use inheritance to obtain
isEmpty
. We could even
override
isEmpty
if it turns out that for some collections there is a faster way of
performing
isEmpty
than computing the current size. However, we cannot imple-
ment
isEmpty
in the
Collection
interface; this can only be done in an abstract
class. This will be the
AbstractCollection
class. To simplify implementations,
programmers designing new
Collections
classes can extend the
AbstractCollec-
tion
class rather than implementing the
Collection
interface. A sample imple-
mentation of
AbstractCollection
is shown in Figures 15.10 to 15.12.
The Collections API also defines additional classes such as
AbstractList
,
AbstractSequentialList
, and
AbstractSet
. We have chosen not to implement
those, in keeping with our intention of providing a simplified subset of the
Collections API. If, for some reason, you are implementing your own collec-
tions and extending the Java Collections API, you should extend the most spe-
cific abstract class.
In Figure 15.10, we see implementations of
isEmpty
,
clear
, and
add
. The
first two methods have straightforward implementations. Certainly the imple-
mentation of
clear
is usable, since it removes all items in the collection, but
there might be more efficient ways of performing the
clear
, depending on the
type of collection being manipulated. Thus this implementation of
clear
serves as a default, but it is likely to be overridden. There is no sensible way
of providing a usable implementation for
add
. So the two alternatives are to
make
add
abstract (which is clearly doable, since
AbstractCollection
is
abstract) or to provide an implementation that throws a runtime exception.
We have done the latter, which matches the behavior in
java.util
. (Further
down the road, this decision also makes it easier to create the class needed to
express the values of a map). Figure 15.11 provides default implementations
The
Abstract-
Collection
imple-
ments some of the
methods in the
Collection
interface.
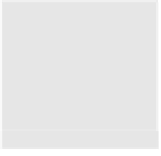
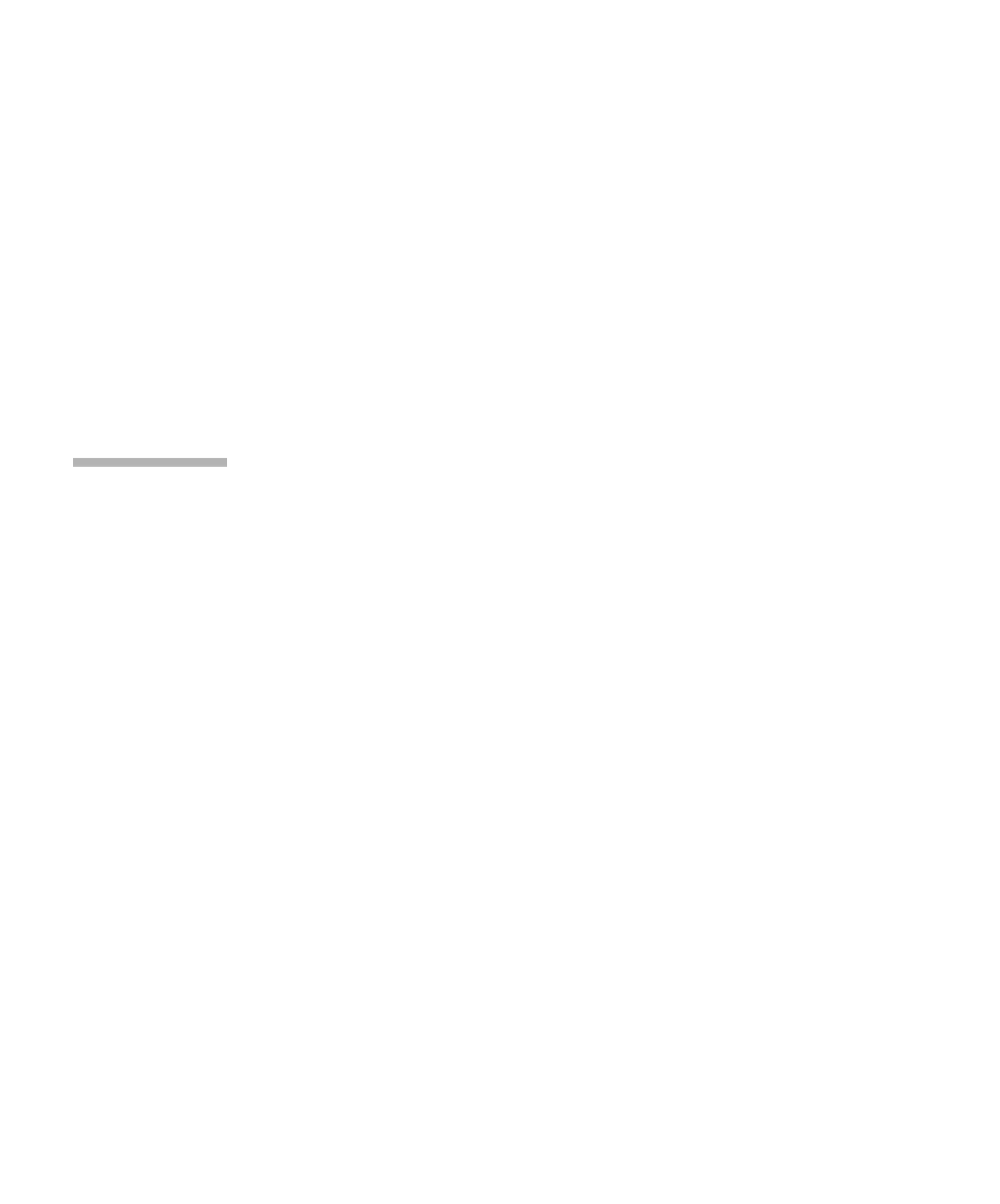
Search WWH ::

Custom Search