Java Reference
In-Depth Information
figure 4.38
The
SimpleRectangle
class, which does not
implement the
Comparable
interface
1
// A simple rectangle class.
2
public class SimpleRectangle
3
{
4
public SimpleRectangle( int len, int wid )
5
{ length = len; width = wid; }
6
7
public int getLength( )
8
{ return length; }
9
10
public int getWidth( )
11
{ return width; }
12
13
public String toString( )
14
{ return "Rectangle " + getLength( ) + " by "
15
+ getWidth( ); }
16
17
private int length;
18
private int width;
19
}
primitive values and references. So we appear not to have a way of passing a
function.
However, recall that an object consists of data and functions. So we can
embed the function in an object and pass a reference to it. Indeed, this idea
works in all object-oriented languages. The object is called a
function object
,
and is sometimes also called a
functor
.
Functor
is another
name for a func-
tion object.
The function object often contains no data. The class simply contains a
single method, with a given name, that is specified by the generic algorithm
(in this case,
findMax
). An instance of the class is then passed to the algo-
rithm, which in turn calls the single method of the function object. We can
design different comparison functions by simply declaring new classes.
Each new class contains a different implementation of the agreed-upon sin-
gle method.
In Java, to implement this idiom we use inheritance, and specifically we
make use of interfaces. The interface is used to declare the signature of the
agreed-upon function. As an example, Figure 4.39 shows the
Comparator
inter-
face, which is part of the standard
java.util
package. Recall that to illustrate
how the Java library is implemented, we will reimplement a portion of
java.util
as
weiss.util
. Before Java 5, this class was not generic.
The interface says that any (nonabstract) class that claims to be a
Comparator
must provide an implementation of the
compare
method; thus any
object that is an instance of such a class has a
compare
method that it can call.
The function object
class contains a
method specified
by the generic
algorithm. An
instance of the
class is passed to
the algorithm.
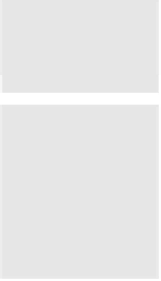




Search WWH ::

Custom Search