Java Reference
In-Depth Information
arrays of parameterized types
Instantiation of arrays of parameterized types is illegal. Consider the following
code:
Instantiation of
arrays of parame-
terized types is
illegal.
ArrayList<String> [ ] arr1 = new ArrayList<String>[ 10 ];
Object [ ] arr2 = arr1;
arr2[ 0 ] = new ArrayList<Double>( );
Normally, we would expect that the assignment at line 3, which has the wrong
type, would generate an
ArrayStoreException
. However, after type erasure, the
array type is
ArrayList[]
, and the object added to the array is
ArrayList
, so
there is no
ArrayStoreException
. Thus, this code has no casts, yet it will even-
tually generate a
ClassCastException
, which is exactly the situation that gener-
ics are supposed to avoid.
the functor (function objects)
4.8
In Sections 4.6 and 4.7, we saw how interfaces can be used to write generic
algorithms. As an example, the method in Figure 4.36 can be used to find the
maximum item in an array.
However, the
findMax
method has an important limitation. That is, it
works only for objects that implement the
Comparable
interface and are able
to provide
compareTo
as the basis for all comparison decisions. There are
many situations in which this is not feasible. As an example, consider the
SimpleRectangle
class in Figure 4.38.
The
SimpleRectangle
class does not have a
compareTo
function, and conse-
quently cannot implement the
Comparable
interface. The main reason for this
is that because there are many plausible alternatives, it is difficult to decide
on a good meaning for
compareTo
. We could base the comparison on area,
perimeter, length, width, and so on. Once we write
compareTo
, we are stuck
with it. What if we want to have
findMax
work with several different comparison
alternatives?
The solution to the problem is to pass the comparison function as a sec-
ond parameter to
findMax
, and have
findMax
use the comparison function
instead of assuming the existence of
compareTo
. Thus
findMax
will now have
two parameters: an array of
Object
of an arbitrary type (which need not have
compareTo
defined), and a comparison function.
The main issue left is how to pass the comparison function. Some lan-
guages allow parameters to be functions. However, this solution often has
efficiency problems and is not available in all object-oriented languages.
Java does not allow functions to be passed as parameters; we can only pass
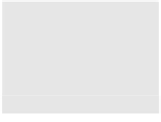


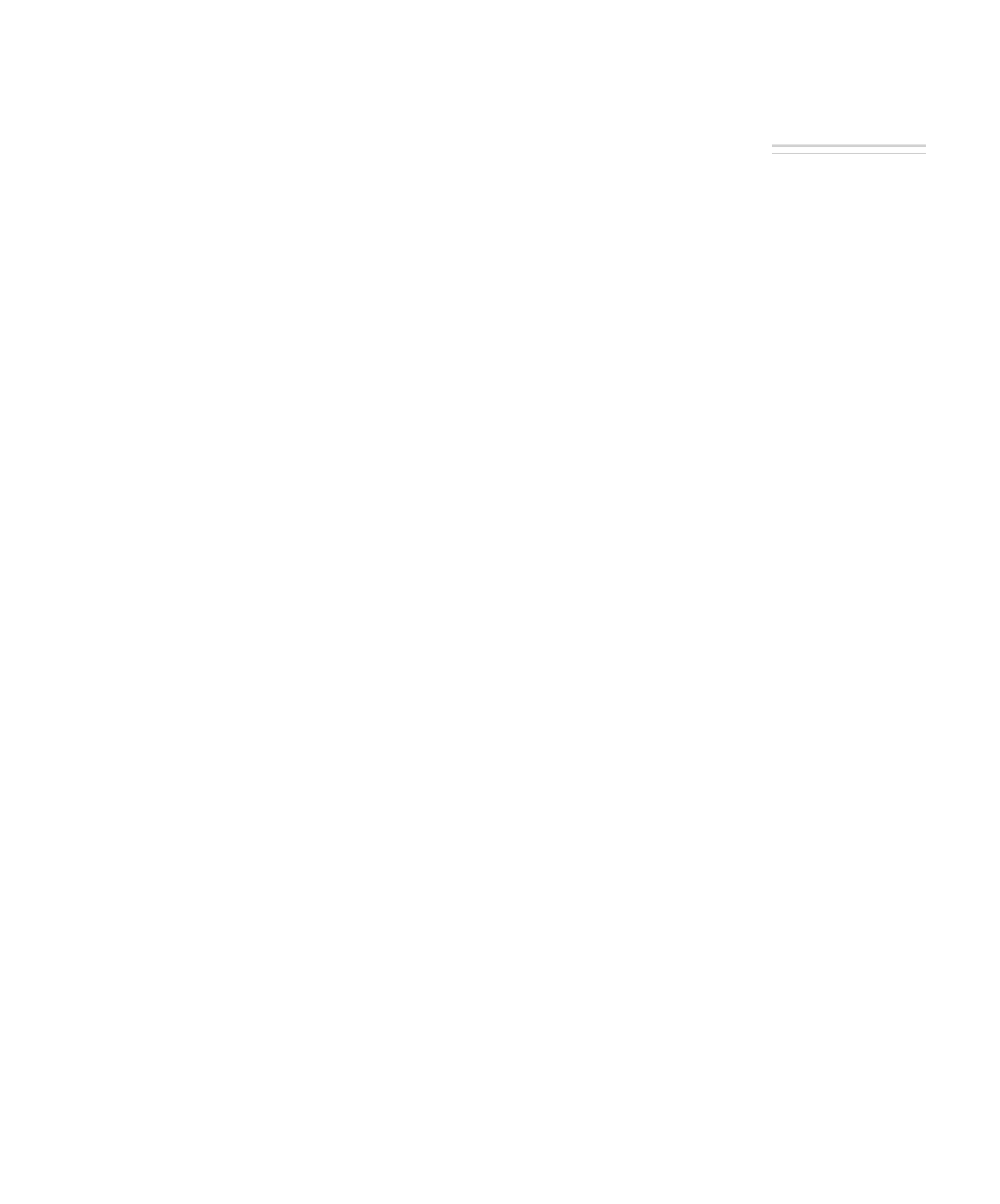
Search WWH ::

Custom Search