Java Reference
In-Depth Information
if
(
Math
.
random
()
>
0.5
)
{
System
.
out
.
println
(
"It's heads"
);
}
else
{
System
.
out
.
println
(
"It's tails"
);
}
To conclude this section, let's briefly discuss Java's
random()
function. When this is
first called, it sets up a new instance of
java.util.Random
. This is a
pseudorandom
number generator
(PRNG)—a deterministic piece of code that produces numbers
that
look
random but are actually produced by a mathematical formula.
2
In Java's
case, the formula used for the PRNG is pretty simple, for example:
// From java.util.Random
public
double
nextDouble
()
{
return
(((
long
)(
next
(
26
))
<<
27
)
+
next
(
27
))
*
DOUBLE_UNIT
;
}
If the sequence of pseudorandom numbers always starts at the same place, then
exactly the same stream of numbers will be produced. To get around this problem,
the PRNG is seeded by a value that should contain as much true randomness as
possible. For this source of randomness for the seed value, Java uses a CPU counter
value that is normally used for high-precision timing.
While Java's built-in pseudorandom numbers are fine for
most general applications, some specialist applications
(notably cryptography and some types of simulations) have
much more stringent requirements. If you are working on
an application of that sort, seek expert advice from pro‐
grammers who are already working in the area.
Now that we've looked at text and numeric data, let's move on to look at another of
the most frequently encountered kinds of data: date and time information.
Java 8 Date and Time
Almost all business software applications have some notion of date and time. When
modeling real-world events or interactions, collecting a point at which the event
occurred is critical for future reporting or comparison of domain objects. Java 8
brings a complete overhaul to the way that developers work with date and time.
This section introduces those concepts for Java 8. In earlier versions, the only sup‐
port is via classes such as
java.util.Date
that do not model the concepts. Code
that uses the older APIs should move as soon as possible.
2
It is very difficult to get computers to produce true random numbers, and in the rare cases where
this is done, specialized hardware is usually necessary.
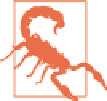
