Java Reference
In-Depth Information
If
tempElement
is not
null
then an element already exists so we modify the existing element to
incorporate the latest cursor position by calling a method
modify()
for the element object. We will
need to add an implementation of this method for each element type. Finally we draw the latest version
of the element referenced by
tempElement
. Since we expect to call the
modify()
method for an
element polymorphically, we should add it to the base class,
Element
. It will be abstract in the
Element
class so add the following declaration to the class definition:
public abstract void modify(Point start, Point last);
Since we reference the
Point
class here we need an
import
statement for it in the
Element.java
file:
import java.awt.Point;
We can implement
createElement()
as a
private
member of the
MouseHandler
class, since it's
not needed anywhere else. The parameters for the method are just two points that will be used to define
each element. Here's the code:
private Element createElement(Point start, Point end) {
switch(theApp.getWindow().getElementType()) {
case LINE:
return new Element.Line(start, end,
theApp.getWindow().getElementColor());
case RECTANGLE:
return new Element.Rectangle(start, end,
theApp.getWindow().getElementColor());
case CIRCLE:
return new Element.Circle(start, end,
theApp.getWindow().getElementColor());
case CURVE:
return new Element.Curve(start, end,
theApp.getWindow().getElementColor());
default:
assert false; // We should never get to here
}
return null;
}
Since we refer to the constants identifying element types here, we must make the
SketchView
class
implement the
Constants
interface, so modify the class to do that now. The first line of the
definition will be:
class SketchView extends JComponent
implements Observer, Constants
The
createElement()
method returns a reference to a shape as type
Element
. We determine the
type of shape to create by retrieving the element type ID stored in the
SketchFrame
class by the menu
item listeners that we put together in the previous chapter. The
getElementType()
method isn't there
in the
SketchFrame
class yet, but you can add it now as:

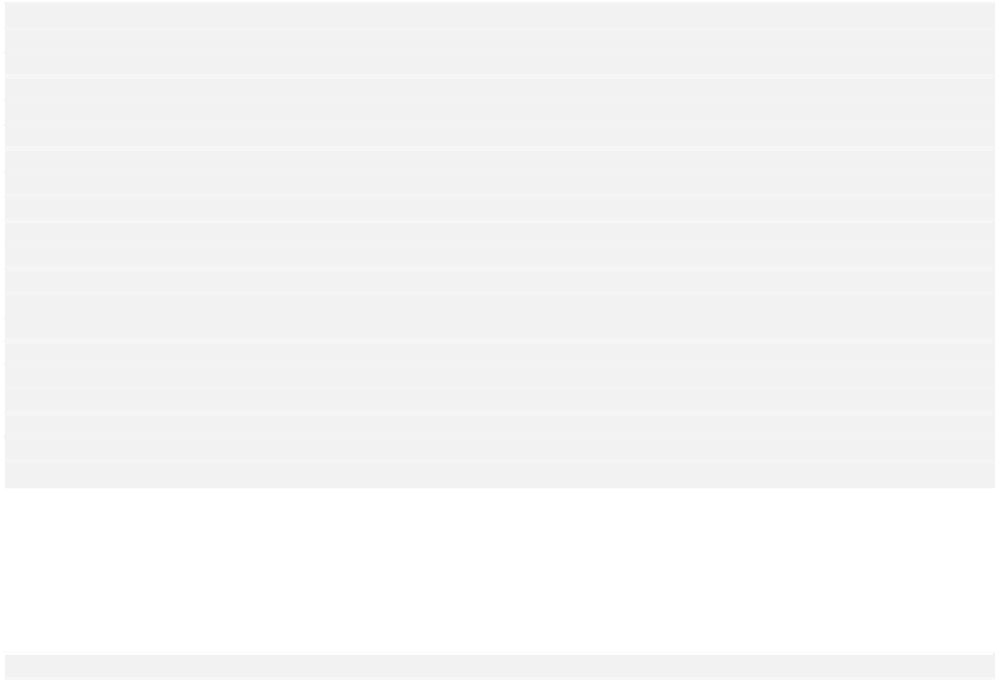






