Java Reference
In-Depth Information
We use the object,
g2D
, to set XOR mode, as we will use this mode in the
mouseDragged()
method
to erase a previously drawn shape without reconstructing the whole sketch. The last thing that is done
here is to retrieve the current drawing color that is recorded in the
SketchFrame
object. You will
remember that this is set when you select a menu item or a toolbar button. We use
theApp
object
stored in the view to get the
SketchFrame
object, and then call its
getElementColor()
member to
retrieve the color. This method doesn't exist in
SketchFrame
, but it's not difficult. Add the following
method to the
SketchFrame
class definition:
public Color getElementColor() {
return elementColor;
}
With the button press code in place, we can have a go at implementing
mouseDragged()
.
Handling Mouse Dragging Events
We can obtain the cursor position in the
mouseDragged()
method in the same way as for the
mousePressed()
method, which is by calling
getPoint()
for the event object, so we could write:
last = e.getPoint(); // Get cursor position
But then we only want to handle drag events for button 1, so we will make this conditional upon the
button1Down
field having the value
true
. When
mouseDragged()
is called for the first time, we
won't have created an element, so we can just create one from the points stored in
start
and
last
,
and then draw it using the graphics context saved by the
mousePressed()
method. The
mouseDragged()
method will be called lots of times while you drag the mouse though, and for every
occasion other than the first, we must take care to redraw the old element before creating the new one.
Since we are in XOR mode, drawing the element a second time will draw it in the background color, so
it will disappear. Here's how we can do all that:
public void mouseDragged(MouseEvent e) {
last = e.getPoint(); // Save cursor position
if(button1Down) {
if(tempElement == null) { // Is there an element?
tempElement = createElement(start, last); // No, so create one
} else {
g2D.draw(tempElement.getShape()); // Yes - draw to erase it
tempElement.modify(start, last); // Now modify it
}
g2D.draw(tempElement.getShape()); // and draw it
}
}
If button 1 is pressed,
button1Down
will be
true
so we are interested. We first check for an existing
element by comparing the reference in
tempElement
with
null
. If there isn't one we create an
element of the current type by calling a method,
createElement()
, that we will add to the
SketchView
class in a moment. We save a reference to the element that is created in the
tempElement
member of the listener object.


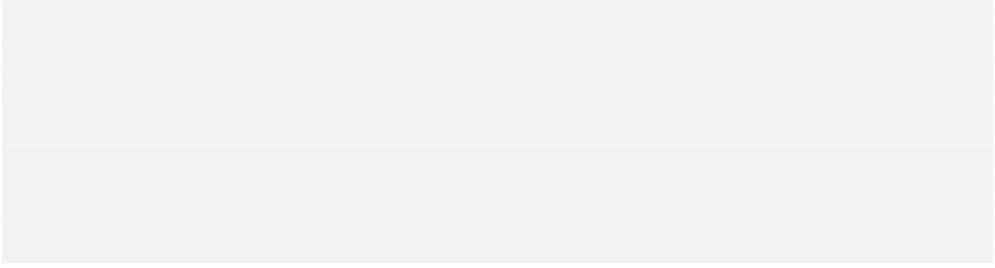








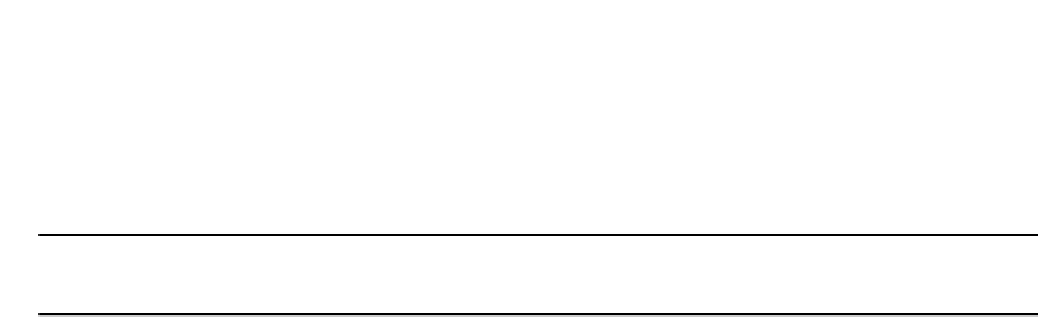