Java Reference
In-Depth Information
You can also set the gaps between components and rows explicitly by calling the
setHgap()
or the
setVgap()
method. To set the horizontal gap to 35 pixels, you would write:
flow.setHgap(35); // Set the horizontal gap
Don't be misled by this. You can't get differential spacing between components by setting the gap
before adding each component to a container. The last values for the gaps between components that
you set for a layout manager will apply to all the components in a container. The methods
getHgap()
and
getVgap()
will return the current setting for the horizontal or vertical gap as a value of type
int
.
The initial size at which the application window is displayed is determined by the values we pass to the
setBounds()
method for the
JFrame
object. If you want the window to assume a size that just
accommodates the components it contains, you can call the
pack()
method for the
JFrame
object.
Add the following line immediately before the call to
setVisible()
:
aWindow.pack();
If you recompile and run the example again, the application window should fit the components.
As we've said, you add components to an applet created as a
JApplet
object in the same way as for a
JFrame
application window. We can verify this by adding some buttons to an example of an applet. We can
try out a
Font
object and add a border to the buttons to brighten them up a bit at the same time.
Try It Out
- Adding Buttons to an Applet
We can define the class for our applet as follows:
import javax.swing.JButton;
import javax.swing.JApplet;
import java.awt.Font;
import java.awt.Container;
import java.awt.FlowLayout;
import javax.swing.border.BevelBorder;
public class TryApplet extends JApplet {
public void init() {
Container content = getContentPane(); // Get content pane
content.setLayout(new FlowLayout(FlowLayout.RIGHT)); // Set layout
JButton button; // Stores a button
Font[] fonts = { new Font("Arial", Font.ITALIC, 10), // Two fonts
new Font("Playbill", Font.PLAIN, 14)
};
BevelBorder edge = new BevelBorder(BevelBorder.RAISED); // Bevelled border
// Add the buttons using alternate fonts
for(int i = 1; i <= 6; i++) {
content.add(button = new JButton("Press " + i)); // Add the button
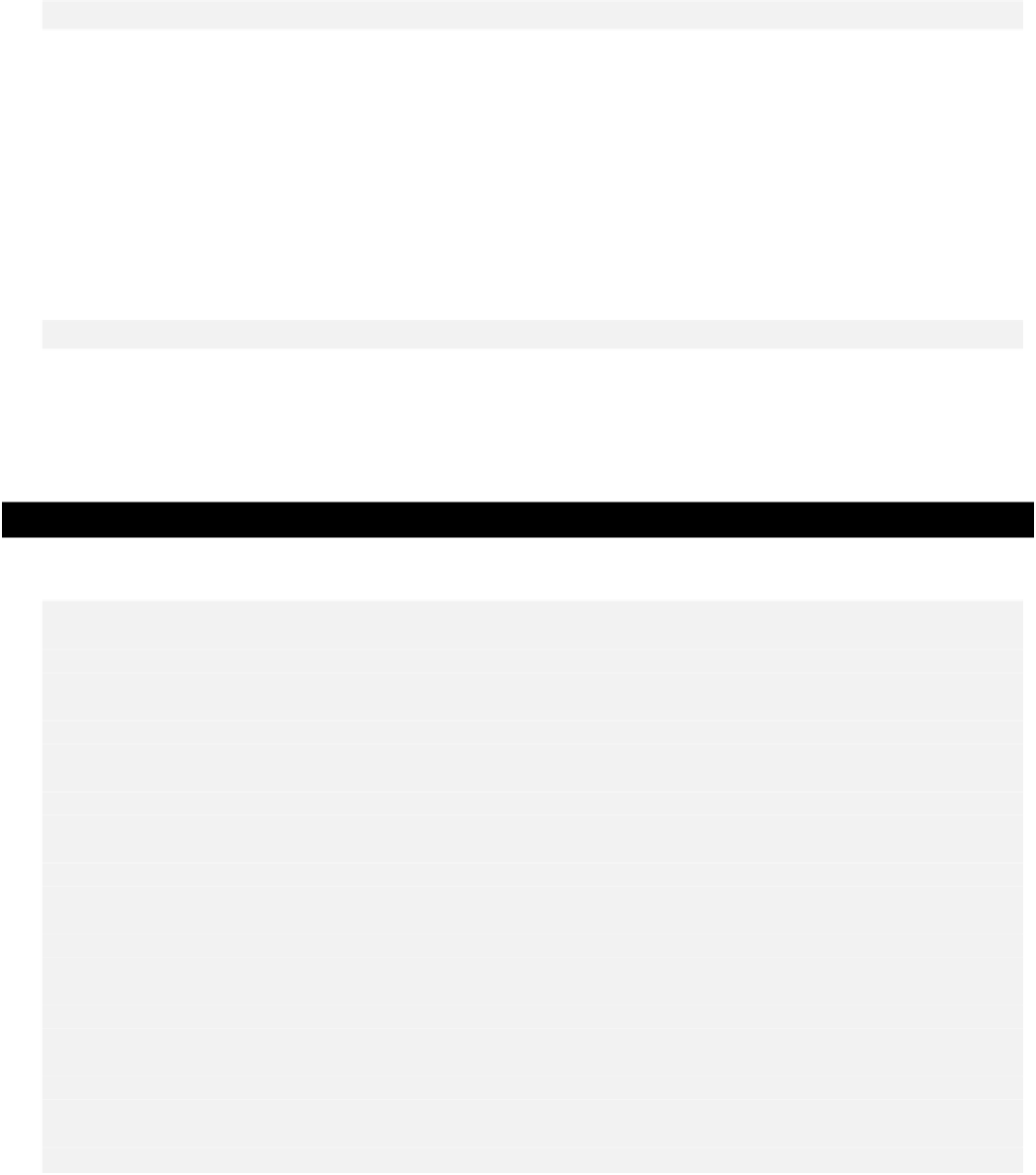









