Java Reference
In-Depth Information
The Lifetime of an Object
The lifetime of an object is determined by the variable that holds the reference to it - assuming there is
only one. If we have the declaration:
Sphere ball = new Sphere(10.0, 1.0, 1.0, 1.0); // Create a sphere
then the
Sphere
object that the variable
ball
refers to will die when the variable
ball
goes out of scope.
This will be at the end of the block containing this declaration. Where an instance variable is the only one
referencing an object, the object survives as long as the instance variable owning the object survives.
A slight complication can arise with objects though. As you have seen, several
variables can reference a single object. In this case the object survives as long as there
is still a variable in existence somewhere that references the object.
You can reset a variable to refer to nothing by setting its value to
null
. If you write the statement:
ball = null;
the variable
ball
no longer refers to an object, and assuming there is no other object referencing it, the
Sphere
object it originally referenced will be destroyed. Note that while the object has been discarded,
the variable
ball
still continues to exist. The lifetime of the object is determined by whether any
variable anywhere in the program still references it.
The process of disposing of dead objects is called
garbage collection
. Garbage collection is automatic in Java,
but this doesn't necessarily mean that objects disappear from memory straight away. It can be some time after
the object becomes inaccessible to your program. This won't affect your program directly in any way. It just
means you can't rely on memory occupied by an object that is done with being available immediately. For
the most part it doesn't matter; the only circumstances where it might would be if your objects were very
large, millions of bytes say, or you were creating and getting rid of very large numbers of objects. In this case
you can call the static
gc()
method defined in the
System
class to encourage the JVM to do some garbage
collecting and recover the memory that the objects occupy:
System.gc();
This is a best efforts deal on the part of the JVM. When the
gc()
method returns, the JVM will have tried to
reclaim the space occupied by discarded objects, but there's no guarantee that it will all be recovered.
Defining and Using a Class
To put what we know about classes to use, we can use our
Sphere
class in an example.
You will be creating two source files. The first is the file
CreateSpheres.java
, which will contain
the definition of the
CreateSpheres
class that will have the method
main()
defined as a static
method. As usual, this is where execution of the program starts. The second file will be the file
Sphere.java
that contains the definition of the class
Sphere
that we have been assembling.
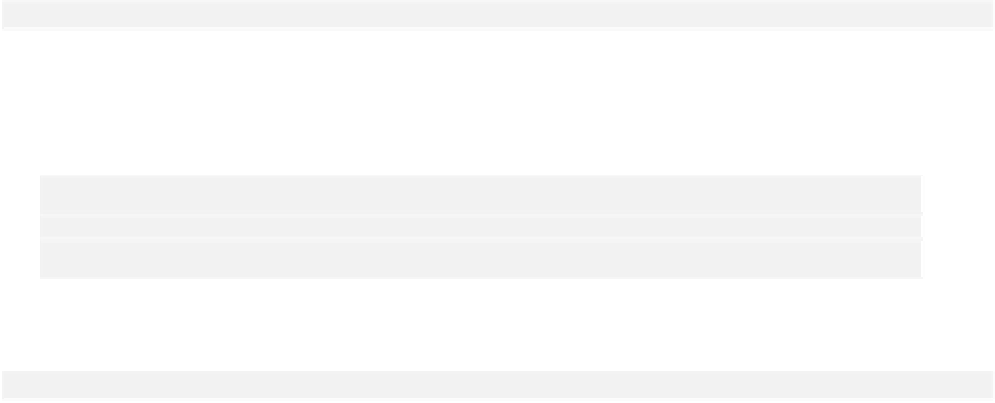














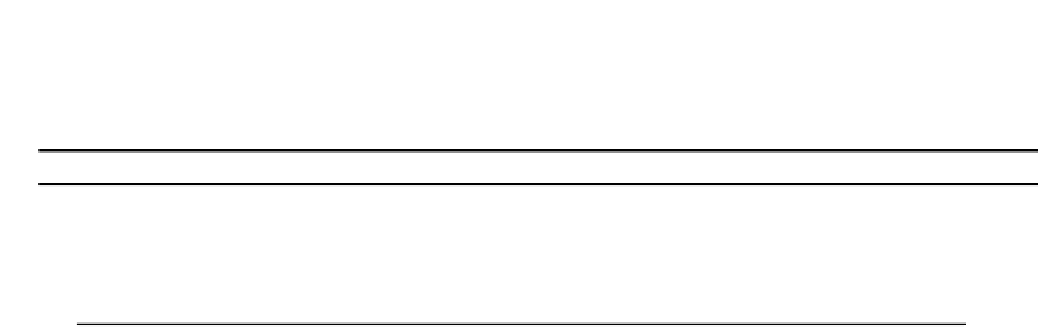