Java Reference
In-Depth Information
Passing Objects to a Method
When you pass an object as an argument to a method, the mechanism that applies is called
pass-by-
reference
, because a copy of the reference contained in the variable is transferred to the method, not the
object itself. The effect of this is shown in the following diagram.
Sphere
object
radius: 10.0
xCenter: 1.0
yCenter: 1.0
zCenter: 1.0
public static void main(String[] args){
...
Sphere ball = new Sphere(10.0, 1.0, 1.0, 1.0);
obj.change(ball);
...
}
This causes a copy
of ball to be made,
but not the Sphere
object
ball and the copy
both refer to
the original object
ball
copy
of
ball
reference
copy made
reference
s refers to
the copy of
ball
acts on
Sphere change( Sphere s){
This statement
modifies the
original object
s.changeRadius(1.0);
return s;
through a copy of
ball
}
This illustration presumes we have defined a method,
changeRadius()
, in the class
Sphere
that will
alter the radius value for an object, and that we have a method
change()
in some other class that calls
changeRadius()
. When the variable
ball
is used as an argument to the method
change()
, the
pass-by-reference mechanism causes a copy of
ball
to be made and stored in
s
. The variable
ball
just
stores a reference to the
Sphere
object, and the copy contains that same reference and therefore refers
to the same object. No copying of the actual object occurs. This is a major plus in term s of efficiency
when passing arguments to a method. Objects can be very complex involving a lot of instance variables.
If objects themselves were always copied when passed as arguments, it could be very time consuming
and make the code very slow.
Since the copy of
ball
refers to the same object as the original, when the
changeRadius()
method is
called the original object will be changed. You need to keep this in mind when writing methods that
have objects as parameters because this is not always what you want.
In the example shown, the method
change()
returns the modified object. In practice you would
probably want this to be a distinct object, in which case you would need to create a new object from
s
.
You will see how you can write a constructor to do this a little later in this chapter.
Remember that this only applies to objects. If you pass a variable of type
int
or
double
to a method for example, a copy of the value is passed. You can modify the
value passed as much as you want in the method, but it won't affect the original value.
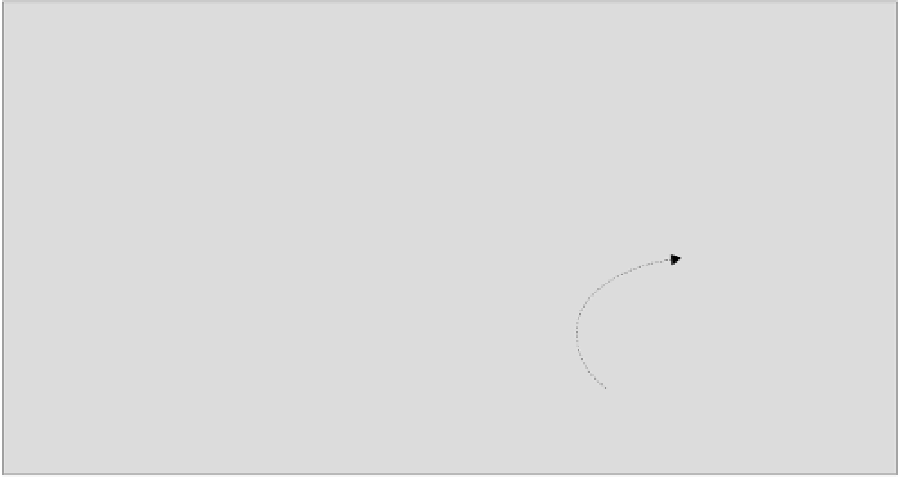




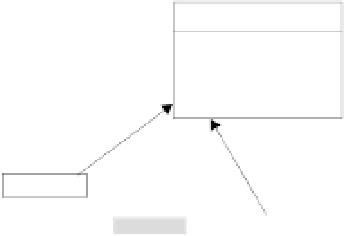





