Java Reference
In-Depth Information
16.
Use the iterator:
DoublyLinkedList.DoublyLinkedIterator i = list.iterator( );
i.restart( );
i.insertHere("Element At Front");
17.
Pop the top of the stack and then push it back on:
String s = stack.pop( );
Stack.push(s);
// s contains the string on the top of the stack
18.
public
void
addToBack(String itemName)
{
Node newEntry =
new
Node(itemName, null);
if
(front ==
null
)
//empty queue
{
back = newEntry;
front = back;
}
else
{
back.link = newEntry;
back = back.link;
}
}
19.
Just note that
aN
+
b
≤
(
a
+
b
)
N
, as long as 1
≤
N
.
20.
This is mathematics, not Java. So, = will mean
equals,
not assignment.
First note that log
a
N
= (log
a
b
)(log
b
N
).
To see this first identity, just note that if you raise
a
to the power log
a
N,
you get
N,
and if you raise
a
to the power (log
a
b
)(log
b
N
) you also get
N
.
If you set
c
= (log
a
b
), you get log
a
N
=
c
(log
b
N
).
21.
The simplest hash function is to map the ID number to the range of the hash
table using the modulus operator:
hash = ID % N;
// N is the hash table size
22.
public
void
outputHashTable( )
{
for
(
int
i=0; i< SIZE; i++)
{
if
(hashArray[i].size( ) > 0)
hashArray[i].outputList( );
}
}
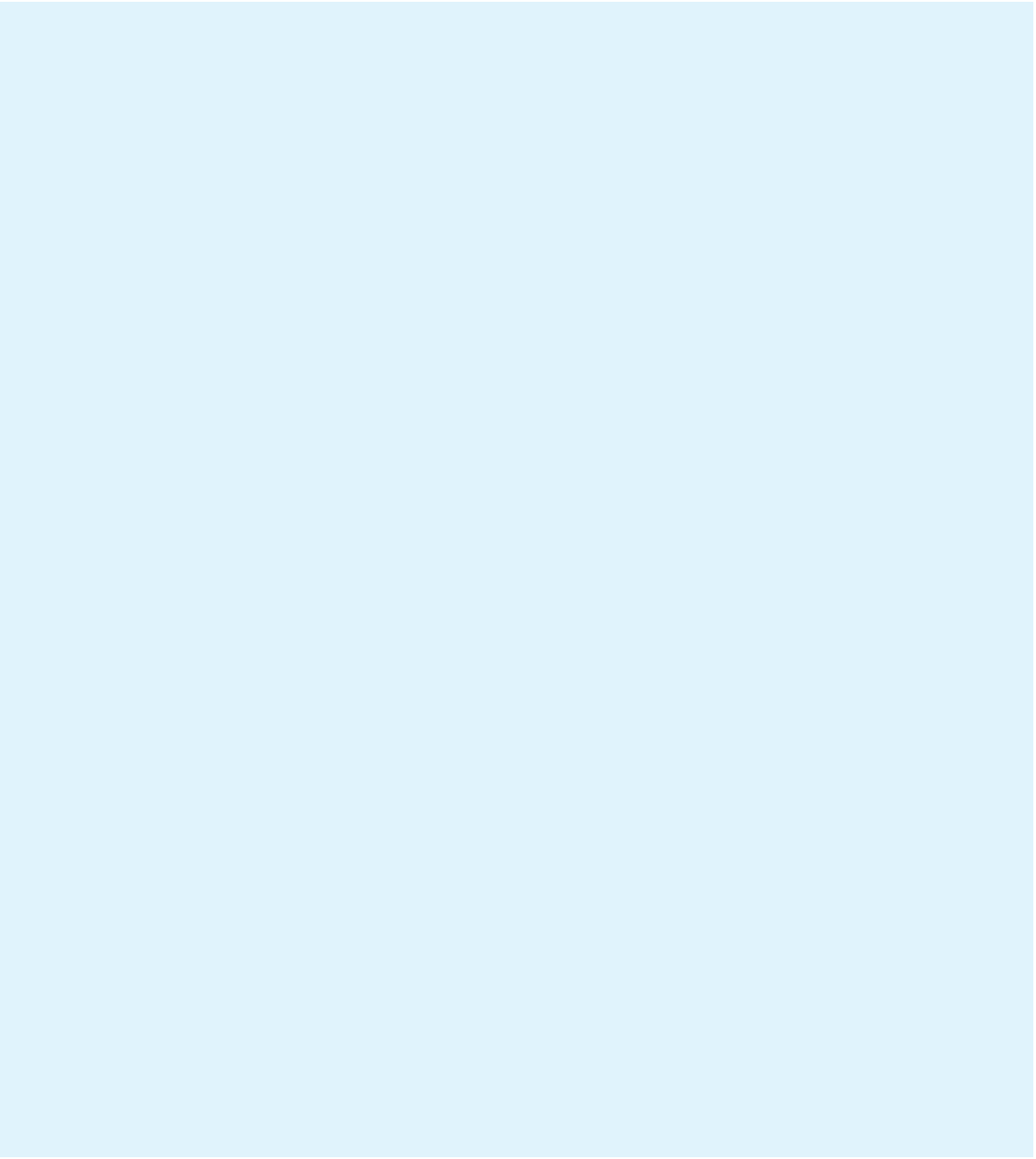

















