Java Reference
In-Depth Information
previous.link = position.link;
position = position.link;
}
return
;
}
previous = current;
// Advance references
current = current.link;
}
}
}
12.
One problem with adding after the iterator's position is that there is no way to
add to the front of the list. It would be possible to make a special case in which
the new node were added to the front (e.g., if
position
is
null
, add the new data
to the head) if desired.
public
void
addAfterHere(String newData)
{
if
(
position
==
null
&& head !=
null
)
{
// At end of list; can't add here
throw
new
IllegalStateException( );
}
else
if
(head ==
null
)
// at head of empty list, add to front
LinkedList2Iter.this.addToStart(newData);
else
{
// Add after current position
Node temp =
new
Node(newData, position.link);
position.link = temp;
}
}
13.
public
void
changeHere(String newData)
{
if
(position ==
null
)
throw
new
IllegalStateException( );
else
position.item = newData;
}
14.
When invoking
i.next( )
, the value of the node that
i
is referencing is copied
to a local variable, the iterator moves to the next node in the link, and then the
value of the local variable is returned. Therefore, the value that
i
is referencing
prior to the invocation is returned.
15.
Insertion and deletion is slightly easier with the doubly linked list because we no
longer need a separate instance variable to keep track of the previous node due to
the previous link. However, all operations require updating more links (e.g.,
both the next and previous instead of just the previous).
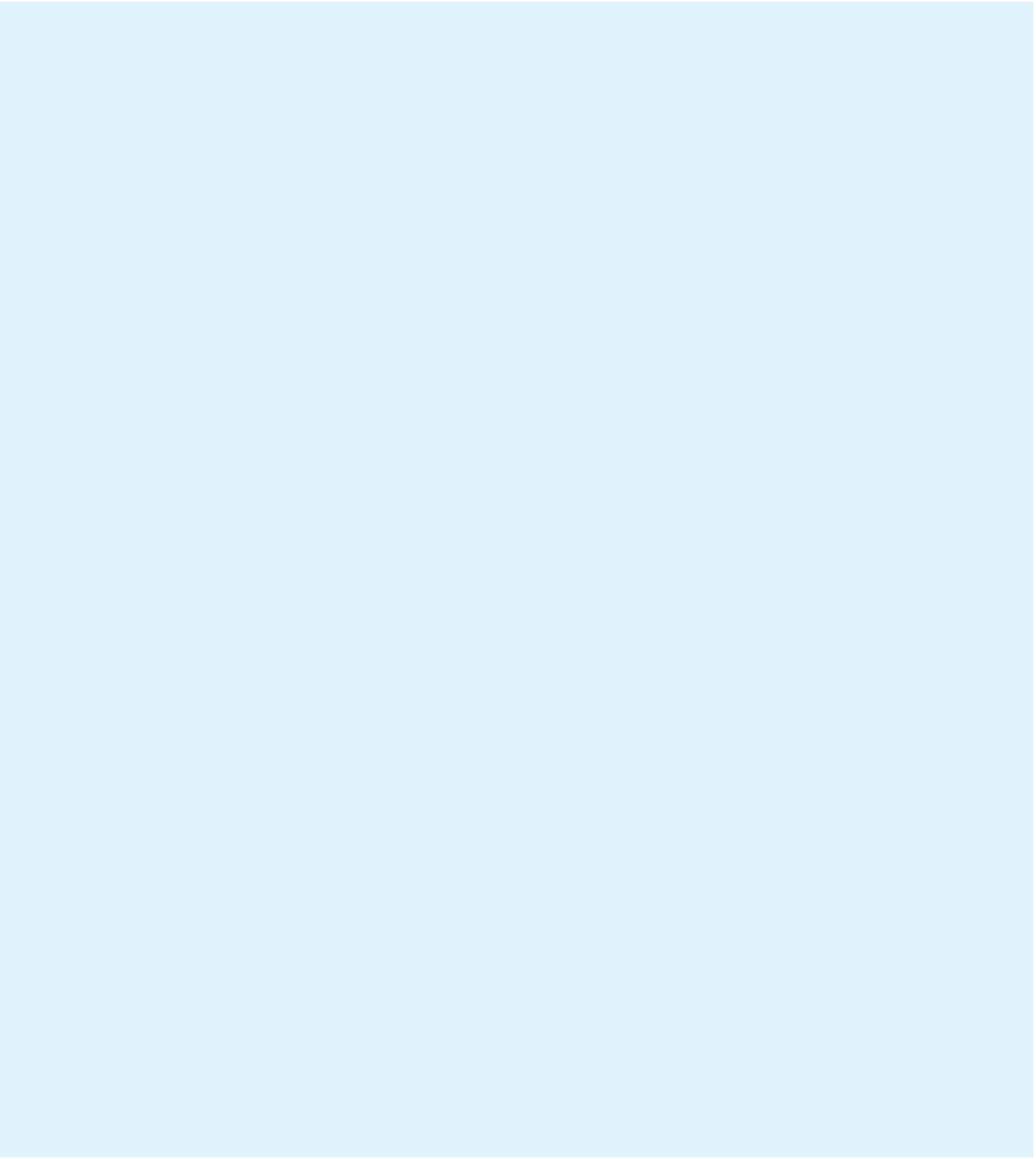

















