Java Reference
In-Depth Information
Display 14.3
Golf Score Program
(part 1 of 2)
1
import
java.util.ArrayList;
2
import
java.util.Scanner;
3
public class
GolfScores
4{
5
/**
6
Shows differences between each of a list of golf scores and their average.
7
*/
8
public static void
main(String[] args)
9
{
10
ArrayList<Double> score =
new
ArrayList<Double>();
11
System.out.println("This program reads golf scores and shows");
12
System.out.println("how much each differs from the average.");
13
System.out.println("Enter golf scores:");
14
fillArrayList(score);
15
showDifference(score);
16
}
17
/**
Parameters of type
ArrayList<Double>()
are
handled just like any other class parameter.
18
Reads values into the array a.
19
*/
20
public static void
fillArrayList(ArrayList<
Double
> a)
21
{
22
System.out.println("Enter a list of nonnegative numbers.");
23
System.out.println("Mark the end of the list with a negative number.");
24
Scanner keyboard =
new
Scanner(System.in);
25
double
next;
26
int
index = 0;
Because of automatic boxing, we can treat
values of type
double
as if their type were
Double
.
27
next = keyboard.nextDouble();
28
while
(next >= 0)
29
{
30
a.add(next);
31
next = keyboard.nextDouble();
32
}
33
}
34
/**
35
Returns the average of numbers in a.
36
*/
37
public static double
computeAverage(ArrayList<
Double
> a)
38
{
39
double
total = 0;
A for-each loop is the nicest way to
cycle through all the elements in an
ArrayList
.
40
for
(Double element : a)
41
total = total + element;
(continued)





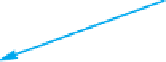
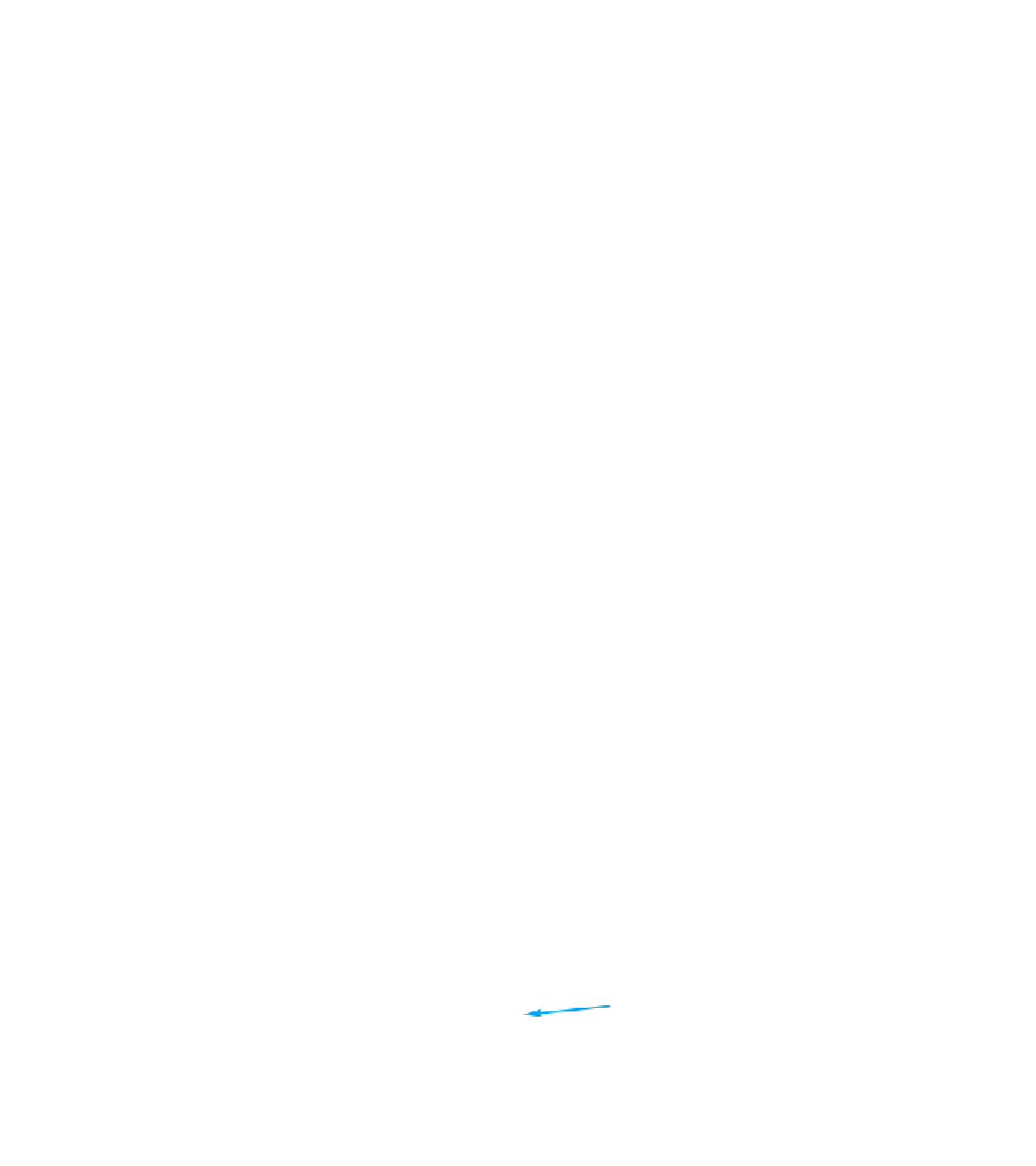

















