Java Reference
In-Depth Information
Self-Test Exercises
(continued)
System.out.println("ArrayList values before sorting:");
for
(String e : b)
System.out.print(e + " ");
System.out.println();
StringSelectionSort.sort(b);
System.out.println("ArrayList values after sorting:");
for
(String e : b)
System.out.print(e + " ");
System.out.println();
}
}
EXAMPLE:
Golf Scores
The program in Display 14.3 reads in a list of golf scores and then outputs the average
of the scores and how much each differs from the average. The scores are read and
stored in an
ArrayList
so that they will be available later in the program to be output
along with how much each differs from the average. This is the kind of thing that is
well suited to being done with an ordinary array. However, it is much easier and
cleaner to use an
ArrayList
as we did in Display 14.3.
Our program deals with a list of values of type
double
. But we use an
ArrayList
with base type
Double
to store these values. We did not use an
ArrayList
with base
type
double
because there is no such thing. The base type for an
ArrayList
must be a
class type (or other reference type). However, thanks to Java's automatic boxing we can
program as if an object of type
ArrayList<Double>
can store values of type
double
.
The
ArrayList
automatically keeps track of how many elements are stored in the
ArrayList
. If we had used an ordinary partially filled array in our program instead of an
ArrayList
, we would need an extra
int
variable to keep track of how much of the array is
used. When we use an
ArrayList
, we are spared all the overhead associated with partially
filled arrays. Those details are taken care of for us automatically. The code for those details
is in the definition of the
ArrayList
class, but there is no need to look at that code. That
code is all implementation detail that we need not worry about when using an
ArrayList
.
Notice the use of for-each loops in our program. The cleanest and easiest way to
cycle through all the elements in an
ArrayList
is to use a for-each loop.
It is instructive to compare the program in Display 14.3, which uses an
ArrayList
,
with the program in Display 6.4, which does the same thing but uses an ordinary
array. The version that uses an
ArrayList
is much cleaner and even much shorter than
the one that uses an ordinary array. This is because an
ArrayList
does so many things
for you automatically, which you would have to explicitly code for if you used an ordi-
nary array. This is a good example of information hiding and code reuse. The pro-
grammers who defined the
ArrayList
class did a lot of programming for you so that
your programming task is simpler than it would otherwise be.


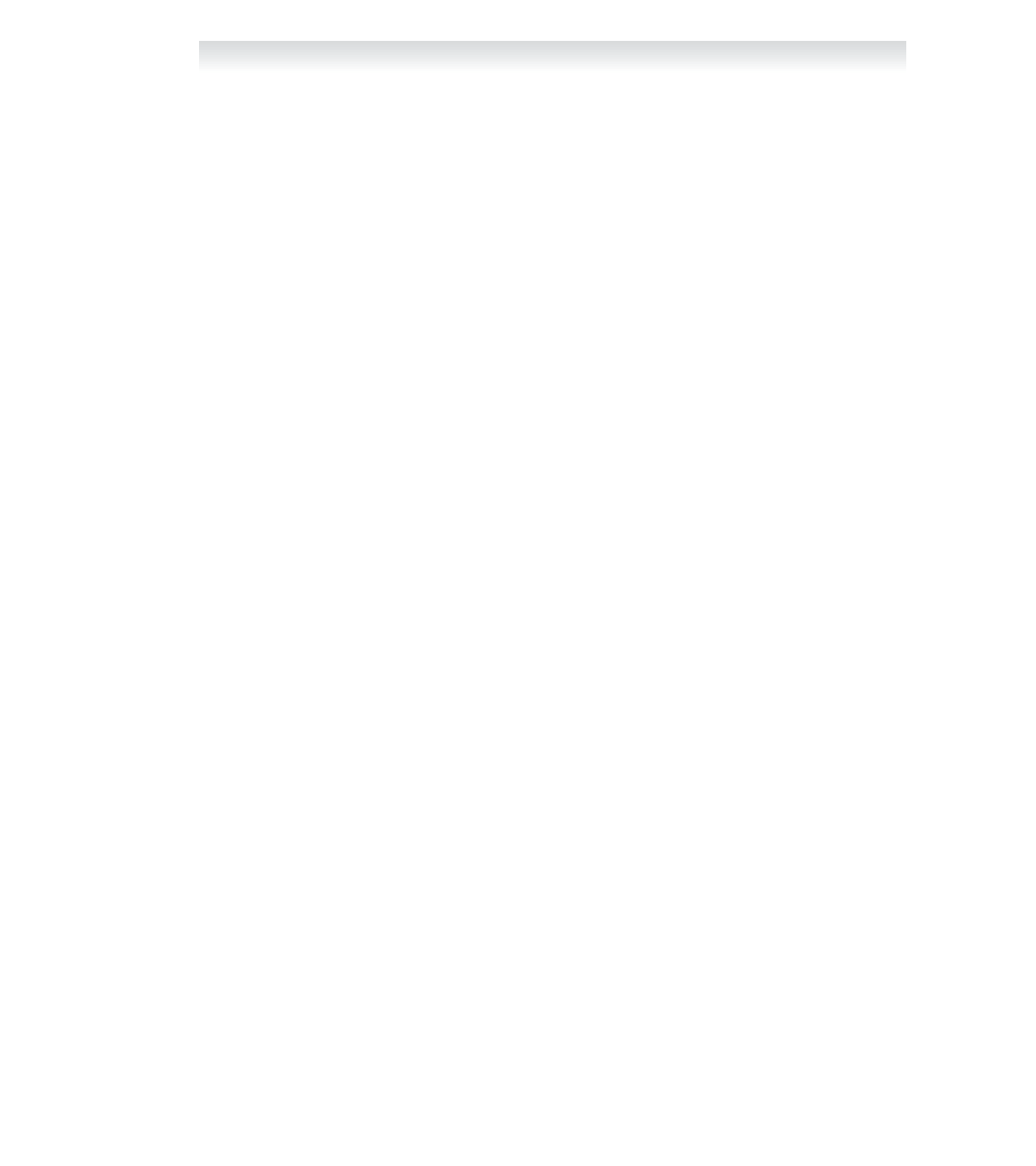

















