Java Reference
In-Depth Information
Place this code into a
try-catch
block with multiple catches so that different
error messages are printed if we attempt to divide by zero or if the user enters
textual data instead of integers (
java.util.InputMismatchException
). If either
of these conditions occurs then the program should loop back and let the user
enter new data.
3.
Modify the previous exercise so that the snippet of code is placed inside a
method. The method should be named
ReturnRatio
and read the input from
the keyboard and throw different exceptions for divide by zero or input mis-
match between text and an integer. Create your own exception class for divide by
zero. Invoke
ReturnRatio
from your
main
method and catch the exceptions in
main
. The
main
method should invoke the
ReturnRatio
method again if any
exception occurs.
4.
(This is a version of an exercise from Chapter 5.) Programming Project 2 from
Chapter 5 asked you to create a class named
Fraction
. This class is used to rep-
resent a ratio of two integers. It should include mutator functions that allow the
user to set the numerator and the denominator along with a method that dis-
plays the fraction on the screen as a ratio (e.g., 5/9). Modify the class so that it
throws the exception
DenominatorIs-ZeroException
if the denominator is set to
zero. Don't forget to account for the constructors! You will have to create the
DenominatorIsZeroException
class and it should be derived from
Exception
.
Write a
main
method that tests the new
Fraction
class, attempts to set the
denominator to zero, and catches the
DenominatorIsZeroException
exception.
5.
Write a program that converts dates from numerical month/day/year format to
normal “month day, year” format (for example, 12/25/2000 corresponds to
December 25, 2000). You will define three exception classes, one called
Month-
Exception
, another called
DayException
, and a third called
YearException
. If
the user enters anything other than a legal month number (integers from
1
to
12
), your program will throw and catch a
MonthException
and ask the user to
reenter the month. Similarly, if the user enters anything other than a valid day
number (integers from
1
to either
28
,
29
,
30
, or
31
, depending on the month and
year), then your program will throw and catch a
DayException
and ask the user
to reenter the day. If the user enters a year that is not in the range 1000 to 3000
(inclusive), then your program will throw and catch a
Year-Exception
and ask
the user to reenter the year. (There is nothing very special about the numbers
1000 and 3000 other than giving a good range of likely dates.) See Self-Test
Exercise 19 in Chapter 4 for details on leap years.
6.
Write a program that can serve as a simple calculator. This calculator keeps track
of a single number (of type
double
) that is called
result
and that starts out as
0.0
. Each cycle allows the user to repeatedly add, subtract, multiply, or divide by
a second number. The result of one of these operations becomes the new value of
result
. The calculation ends when the user enters the letter R for “result” (either
in uppercase or lowercase). The user is allowed to do another calculation from
the beginning as often as desired.
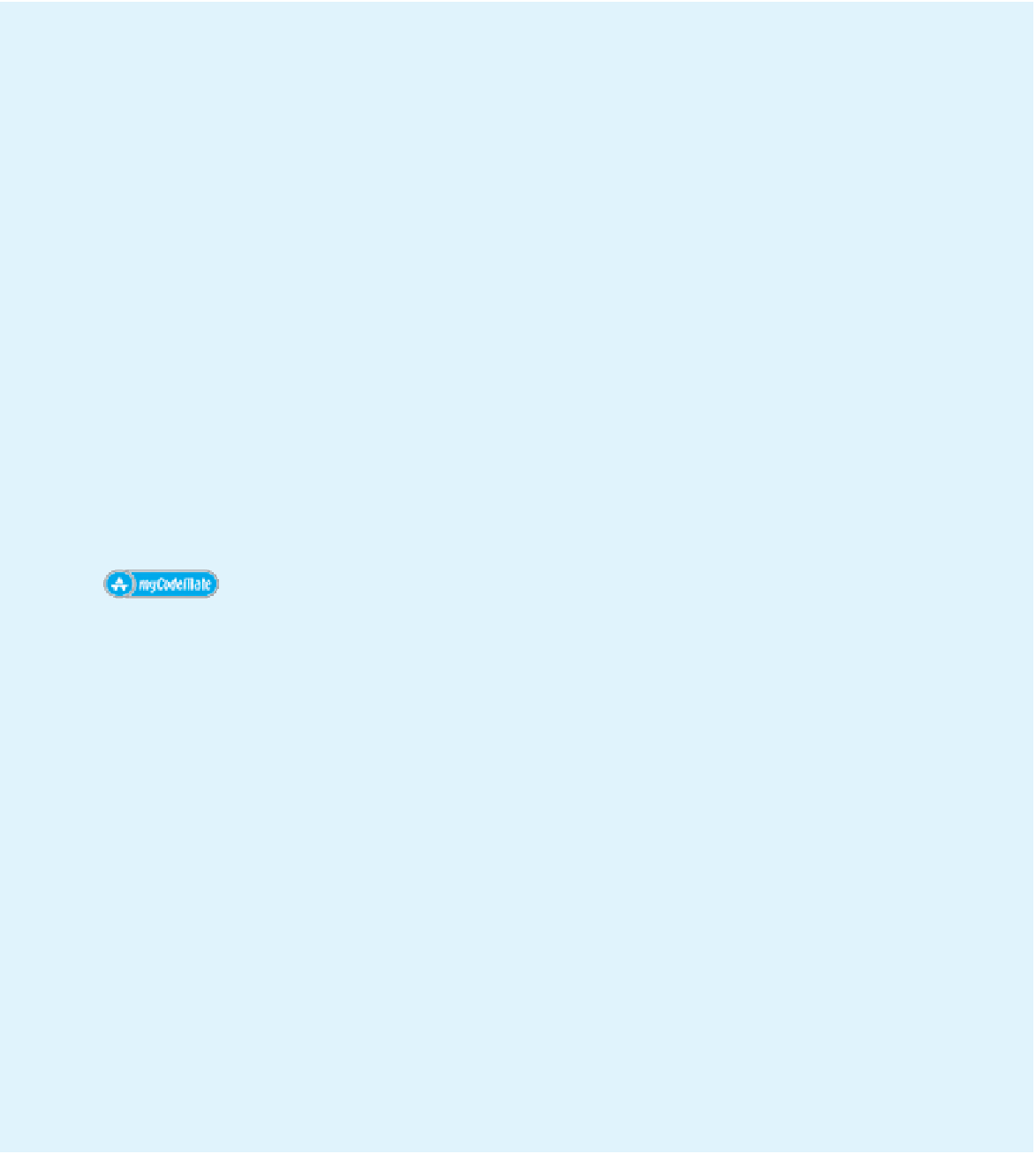
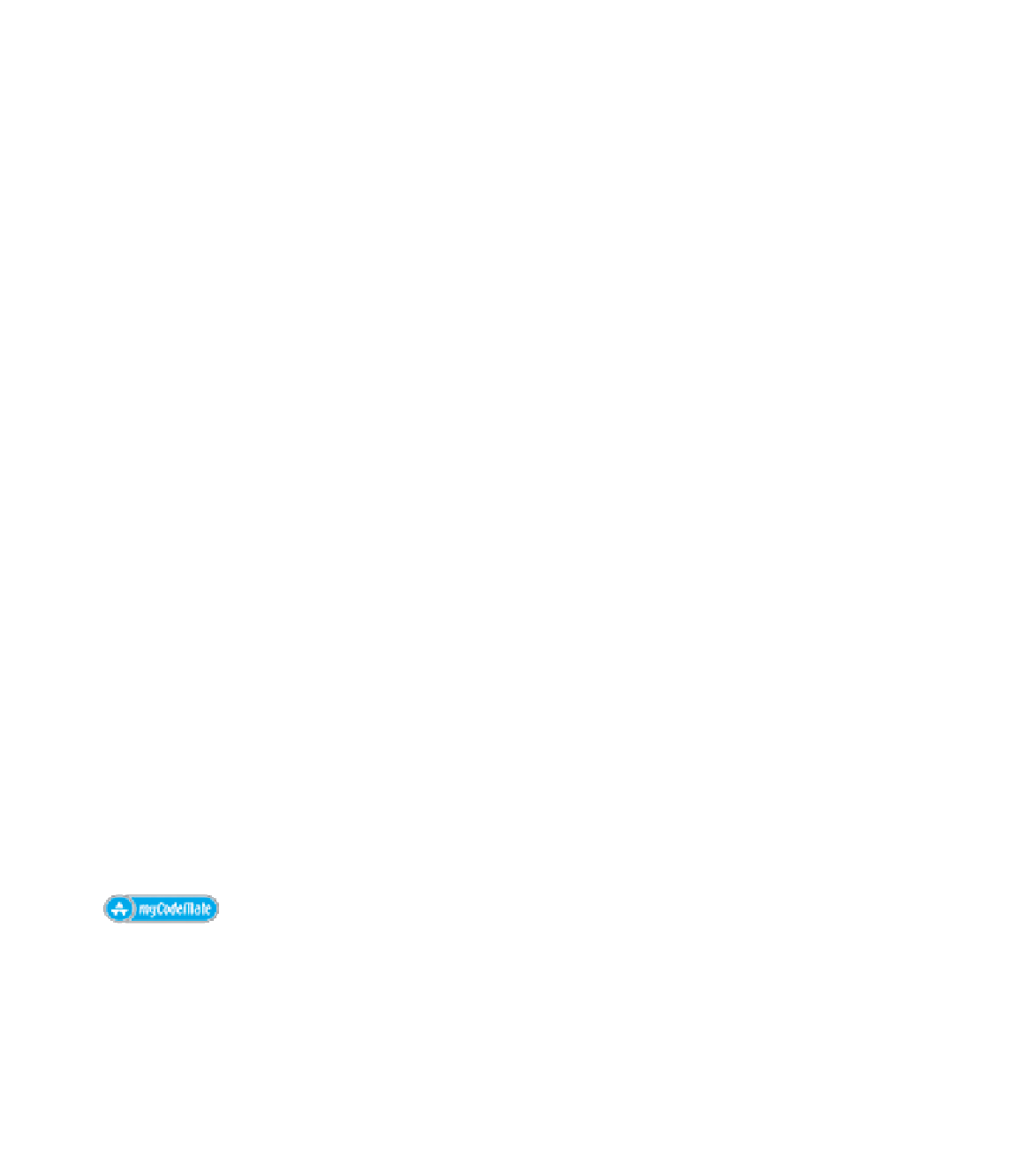

















