Java Reference
In-Depth Information
try
{
System.out.println("Enter a whole number:");
number = keyboard.nextInt();
done =
true
;
}
catch
(InputMismatchException e)
{
keyboard.nextLine();
System.out.println(
"Not a correctly written whole number.");
System.out.println("Try again.");
}
}
return
number;
}
public static void
main(String[] args)
{
Scanner keyboardArg =
new
Scanner(System.in);
int
number = getInt(keyboardArg);
System.out.println("You entered " + number);
}
}
Programming Projects
Many of these Programming Projects can be solved using AW's CodeMate.
To access these please go to:
www.aw-bc.com/codemate
.
1.
Write a program that calculates the average of
N
integers. The program should
prompt the user to enter the value for
N
and then afterward must enter all
N
numbers. If the user enters a non-positive value for
N,
then an exception should
be thrown (and caught) with the message “
N
must be positive.” If there is any
exception as the user is entering the
N
numbers, an error message should be dis-
played and the user prompted to enter the number again.
2.
Here is a snippet of code that inputs two integers and divides them:
Scanner scan =
new
Scanner(System.in);
int
n1, n2;
double
r;
n1 = scan.nextInt();
n2 = scan.nextInt();
r = (
double
) n1 / n2;
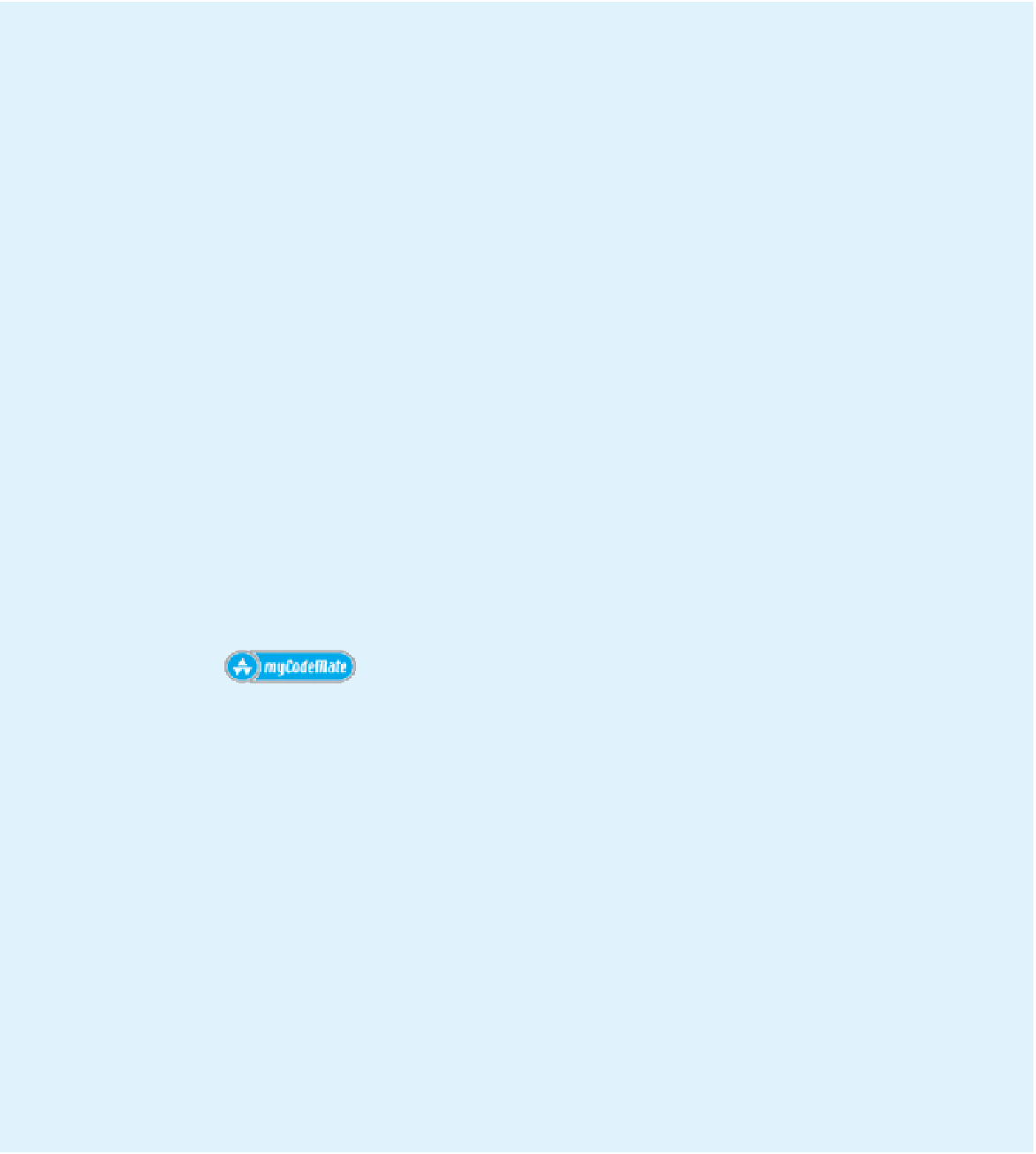


















