Java Reference
In-Depth Information
Self-Test Exercises
(continued)
public
DoubleException(
double
number)
{
super
("DoubleException thrown!");
doubleMessage = number;
}
public double
getNumber()
{
return doubleMessage;
}
}
What output would be produced by the following code (which is just an exercise and
not likely to occur in a program)?
DoubleException e =
new
DoubleException(41.9);
System.out.println(e.getNumber());
System.out.println(e.getMessage());
The class
DoubleException
is on the CD that comes with this text.
18. There is an exception class named
IOException
that is defined in the standard Java
libraries. Can you define an exception class as a derived class of the predefined class
IOException
, or must a defined exception class be derived from the class
Exception
?
extra code
on CD
Multiple
catch
Blocks
A
try
block can potentially throw any number of exception values, and they can be of
differing types. In any one execution of the
try
block, at most one exception will be
thrown (since a
throw
statement ends the execution of the
try
block), but different
types of exception values can be thrown on different occasions when the
try
block is
executed. Each
catch
block can only catch values of the exception class type given in
the
catch
block heading. However, you can catch exception values of differing types by
placing more than one
catch
block after a
try
block. For example, the program in Dis-
play 9.7 has two
catch
blocks after its
try
block. The class
NegativeNumberException
,
which is used in that program, is given in Display 9.8.
Display 9.7
Catching Multiple Exceptions
(part 1 of 3)
1
import
java.util.Scanner;
2
public class
MoreCatchBlocksDemo
3{
4
public static void
main(String[] args)
5
{
6
Scanner keyboard =
new
Scanner(System.in);
7

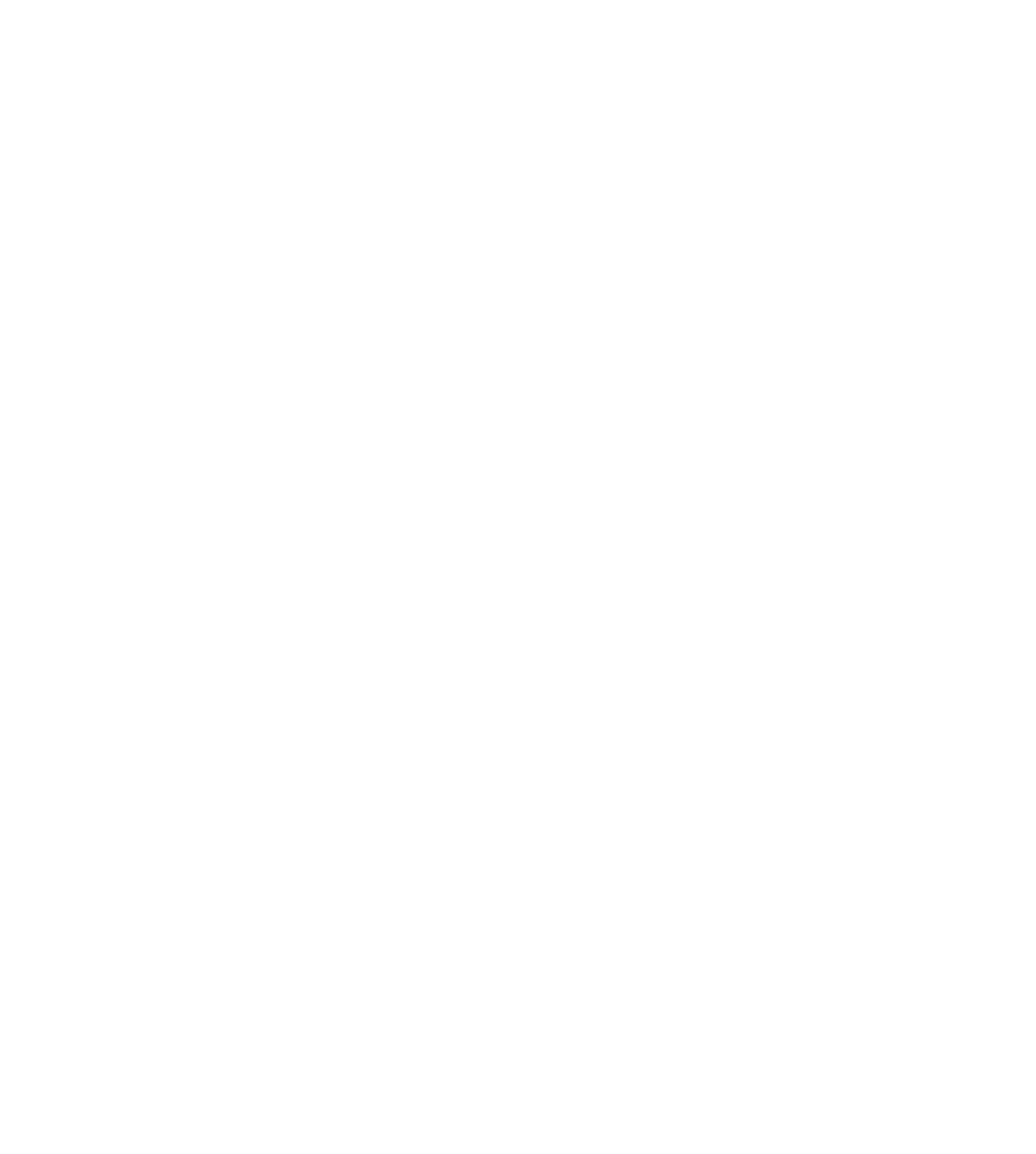

















