Java Reference
In-Depth Information
To read a string from the console, invoke the
next()
method on a
Scanner
object. For
example, the following code reads three strings from the keyboard:
read strings
Scanner input =
new
Scanner(System.in);
System.out.println(
"Enter three words separated by spaces: "
);
String s1 = input.next();
String s2 = input.next();
String s3 = input.next();
System.out.println(
"s1 is "
+ s1);
System.out.println(
"s2 is "
+ s2);
System.out.println(
"s3 is "
+ s3);
Enter three words separated by spaces:
s1 is Welcome
s2 is to
s3 is Java
Welcome to Java
The
next()
method reads a string that ends with a whitespace character. The characters
' '
,
\t
,
\f
,
\r
, or
\n
are known as
whitespace characters
.
You can use the
nextLine()
method to read an entire line of text. The
nextLine()
method reads a string that ends with the
Enter
key pressed. For example, the following state-
ments read a line of text.
whitespace character
Scanner input =
new
Scanner(System.in);
System.out.println(
"Enter a line: "
);
String s = input.nextLine();
System.out.println(
"The line entered is "
+ s);
Enter a line:
The line entered is Welcome to Java
Welcome to Java
Important Caution
To
avoid input errors,
do not use
nextLine()
after
nextByte()
,
nextShort()
,
nextInt()
,
nextLong()
,
nextFloat()
,
nextDouble()
, or
next()
. The rea-
sons will be explained in Section 14.11.3, “How Does
Scanner
Work?”
avoid input errors
2.32
✓
✓
Show the output of the following statements (write a program to verify your results):
Check
Point
System.out.println(
"1"
+
1
);
System.out.println(
'1'
+
1
);
System.out.println(
"1"
+
1
+
1
);
System.out.println(
"1"
+ (
1
+
1
));
System.out.println(
'1'
+
1
+
1
);
2.33
Evaluate the following expressions (write a program to verify your results):
1
+
"Welcome "
+
1
+
1
1
+
"Welcome "
+ (
1
+
1
)
1
+
"Welcome "
+ (
'\u0001'
+
1
)
1
+
"Welcome "
+
'a'
+
1
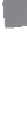
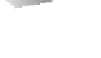





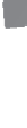
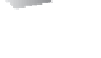

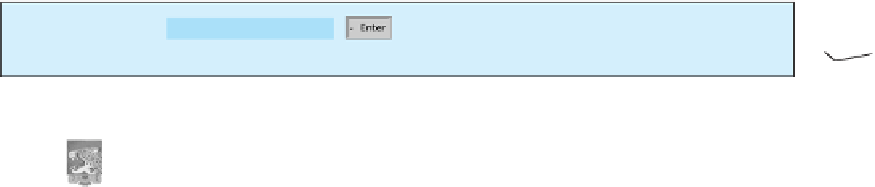








