Java Reference
In-Depth Information
2.31
Show the output of the following program:
public class
Test {
public static void
main(String[] args) {
char
x =
'a'
;
char
y =
'c'
;
System.out.println(++x);
System.out.println(y++);
System.out.println(x - y);
}
}
Key
Point
The
char
type represents only one character. To represent a string of characters, use the data
type called
String
. For example, the following code declares the message to be a string with
the value
"Welcome to Java"
.
String message =
"Welcome to Java"
;
String
is a predefined class in the Java library, just like the classes
System
,
JOptionPane
,
and
Scanner
. The
String
type is not a primitive type. It is known as a
reference type.
Any Java
class can be used as a reference type for a variable. Reference data types will be thoroughly dis-
cussed in Chapter 8, Objects and Classes. For the time being, you need to know only how to
declare a
String
variable, how to assign a string to the variable, and how to concatenate strings.
As first shown in Listing 2.1, two strings can be concatenated. The plus sign (
+
) is the con-
catenation operator if one of the operands is a string. If one of the operands is a nonstring
(e.g., a number), the nonstring value is converted into a string and concatenated with the other
string. Here are some examples:
// Three strings are concatenated
String message =
"Welcome "
+
"to "
+
"Java"
;
concatenate strings and
numbers
// String Chapter is concatenated with number 2
String s =
"Chapter"
+
2
;
// s becomes Chapter2
// String Supplement is concatenated with character B
String s1 =
"Supplement"
+
'B'
;
// s1 becomes SupplementB
If neither of the operands is a string, the plus sign (
+
) is the addition operator that adds
two numbers.
The augmented
+=
operator can also be used for string concatenation. For example, the fol-
lowing code appends the string
"and Java is fun"
with the string
"Welcome to Java"
in
message
.
message +=
" and Java is fun"
;
So the new
message
is
"Welcome to Java and Java is fun"
.
If
i = 1
and
j = 2
, what is the output of the following statement?
System.out.println(
"i + j is "
+ i + j);
The output is
"i + j is 12"
because
"i + j is "
is concatenated with the value of
i
first. To force
i + j
to be executed first, enclose
i + j
in the parentheses, as follows:
System.out.println(
"i + j is "
+ i + j );
(
)



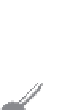


