Java Reference
In-Depth Information
14.11.4 Case Study: Replacing Text
Suppose you are to write a program named
ReplaceText
that replaces all occurrences of a
string in a text file with a new string. The file name and strings are passed as command-line
arguments as follows:
java ReplaceText sourceFile targetFile oldString newString
For example, invoking
java ReplaceText FormatString.java t.txt StringBuilder StringBuffer
replaces all the occurrences of
StringBuilder
by
StringBuffer
in the file
FormatString.java
and saves the new file in
t.txt
.
Listing 14.15 gives the program. The program checks the number of arguments passed to
the
main
method (lines 7-11), checks whether the source and target files exist (lines 14-25),
creates a
Scanner
for the source file (line 28), creates a
PrintWriter
for the target file, and
repeatedly reads a line from the source file (line 32), replaces the text (line 33), and writes a
new line to the target file (line 34). You must close the output file (line 38) to ensure that data
are saved to the file properly.
L
ISTING
14.15
ReplaceText.java
1
import
java.io.*;
2
import
java.util.*;
3
4
public class
ReplaceText {
5
public static void
main(String[] args)
throws
Exception {
6
// Check command-line parameter usage
7
if
(args.length !=
4
) {
8 System.out.println(
9
"Usage: java ReplaceText sourceFile targetFile oldStr newStr"
);
10 System.exit(
1
);
11 }
12
13
check command usage
// Check if source file exists
14
15
if
( ) {
16 System.out.println(
"Source file "
+ args[
0
] +
" does not exist"
);
17
File sourceFile =
new
File(args[
0
]);
!sourceFile.exists()
source file exists?
System.exit(
2
);
18 }
19
20
// Check if target file exists
21
22
if
( ) {
23 System.out.println(
"Target file "
+ args[
1
] +
" already exists"
);
24 System.exit(
3
);
25 }
26
27
File targetFile =
new
File(args[
1
]);
targetFile.exists()
target file exists?
// Create a Scanner for input and a PrintWriter for output
28
29 PrintWriter output =
new
PrintWriter(targetFile);
30
31
while
( ) {
32 String s1 = input.nextLine();
33 String s2 = s1.replaceAll(args[
2
], args[
3
]);
34 output.println(s2);
35 }
36
Scanner input =
new
Scanner(sourceFile);
create a
Scanner
create a
PrintWriter
input.hasNext()
has next?
read a line
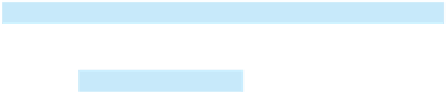

