Java Reference
In-Depth Information
Caution
Attempting to access characters in a string
s
out of bounds is a common program-
ming error. To avoid it, make sure that you do not use an index beyond
s.length()
-
1
. For example,
s.charAt(s.length())
would cause a
StringIndexOutOfBoundsException
.
string index range
You can use the
concat
method to concatenate two strings. The statement shown below, for
example, concatenates strings
s1
and
s2
into
s3
:
String s3 = s1.concat(s2);
s1.concat(s2)
Because string concatenation is heavily used in programming, Java provides a convenient way
to accomplish it. You can use the plus (
+
) operator to concatenate two strings, so the previous
statement is equivalent to
String s3 = s1 + s2;
s1 + s2
The following code combines the strings
message
,
" and "
, and
"HTML"
into one string:
String myString = message +
" and "
+
"HTML"
;
Recall that the
+
operator can also concatenate a number with a string. In this case, the num-
ber is converted into a string and then concatenated. Note that at least one of the operands
must be a string in order for concatenation to take place.
9.2.5 Obtaining Substrings
You can obtain a single character from a string using the
charAt
method, as shown in Figure 9.3.
You can also obtain a substring from a string using the
substring
method in the
String
class,
as shown in Figure 9.5.
For example,
String message =
"Welcome to Java"
.substring(
0
,
11
) +
"HTML"
;
The string
message
now becomes
Welcome to HTML
.
java.lang.String
+substring(beginIndex: int):
String
Returns this string's substring that begins with the character at the
specified
beginIndex
and extends to the end of the string, as
shown in Figure 9.6.
Returns this string's substring that begins at the specified
beginIndex
and extends to the character at index
endIndex - 1
,
as shown in Figure 9.6. Note that the character at
endIndex
is not
part of the substring.
+substring(beginIndex: int,
endIndex: int): String
F
IGURE
9.5
The
String
class contains the methods for obtaining substrings.
0123456789 0 1 2 3 4
We l
Indices
Message
c om e
t o
J a v a
message.substring(0, 11) message.substring(11)
F
IGURE
9.6
The
substring
method obtains a substring from a string.


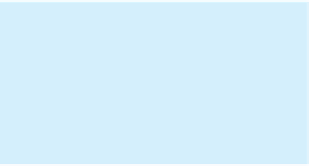
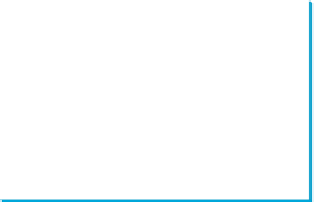




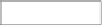


