Game Development Reference
In-Depth Information
In3Dgames, the positional data we will record will, ofcourse be in three dimensions, heat
maps as we have described them so far are 2D images, so how can we represent 3D data
as a heat map? One possibility is to ignore the up component, grab only the
x,z
coordinates
and generate the heat map, we can then overlay this heat map over a top-down view of the
game world, such as the game's mini-map and we would be able to have a birds-eye view
of the areas of the game where players die the most, or the most often taken paths. Another
option is to take the heat map system into the 3D world, all the recorded world space po-
sitions will give us a point cloud, we can render a sprite in world space, mapped with the
radial gradient texturewithanadditiveblendmodeenabledateachpointinthepointcloud
then colorized according to the look up table, the result will be a three dimensional heat
cloudthatwecanstudyaswemovearoundtheactualgameworld;themoredataavailable,
the better the heat cloud will look.
To create a 2D heatmap we will need a buffer large enough to contain the data we want to
record, a buffer of a size that is representative of an area of the game world we are inter-
ested in analyzing. Often we will want to overlay our heatmap over the game's minimap
if one is available. Ultimately the size will depend on the game and the way we choose to
display it.
class heatmap
{
public:
heatmap(render::device_direct3d* device, int size, int pointSize, const std::wstring colorMapPath)
: m_device(device)
, m_size(size)
, m_gradient(pointSize, render::color::TRANSPARENT, render::color::WHITE)
{
m_data = new unsigned int[size*size];
memset(m_data, 0, sizeof(unsigned int)*size*size);
m_colorLUT = std::unique_ptr<color_lut>(new color_lut(device, colorMapPath));
}
~heatmap()
{
delete [] m_data;
}
On construction we allocate the buffer into which we will write the heatmap pixels and
clear it to all black, including the alpha channel, we also create the radial gradient for the
intensity map, it ranges from black to white, we will draw this gradient onto our heatmap
buffer at any point in the data we sample.
There are two ways we could consider generating the heatmap, the first would be to gen-
erate it in immediate mode, by adding one point at a time or deferred by iterating over a
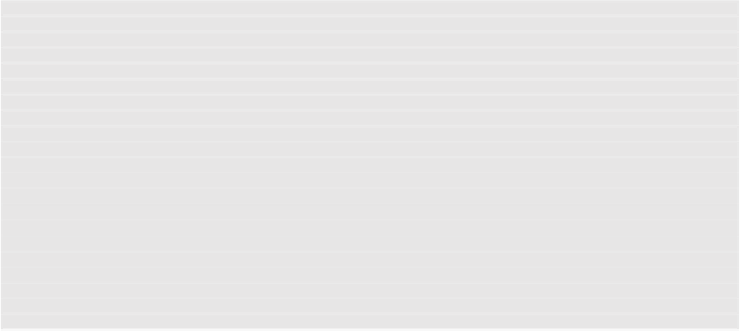


