Java Reference
In-Depth Information
types. The
Long
class also defines a static method
toHexString()
that you use to obtain a string that
is a hexadecimal representation of a value of type
long
. These classes also contain other useful utility
methods that I introduce later when a suitable context arises.
Of course, you can use the static import statement that I introduced in the context of the
Math
class to im-
port the names of static members of other classes such as
Integer
and
Long
. For example, the following
statement at the beginning of a source file enables you to use the
toHexString()
method without having
to qualify it with the
Integer
class name:
import static java.lang.Integer.toHexString;
BITWISE OPERATIONS
As you already know, all these integer variables I have been talking about are represented internally as bin-
ary numbers. A value of type
int
consists of 32 binary digits, known to us computer fans as bits. You can
operate on the bits that make up integer values using the bitwise operators, of which there are four available,
as shown in
Table 2-7
:
AND
OR
|
Exclusive OR
^
Complement
~
Each of these operators operates on the individual bits in its operands as follows:
• The bitwise AND operator,
&
, combines corresponding bits in its two operands such that if both
bits are 1, the result is 1 — otherwise the result is 0.
• The bitwise OR operator,
|
, combines corresponding bits such that if either or both bits are 1, then
the result is 1. Only if both bits are 0 is the result 0.
• The bitwise exclusive OR (XOR) operator,
^
, combines corresponding bits such that if both bits
are the same the result is 0; otherwise, the result is 1.
• The complement operator,
~
, takes a single operand in which it inverts all the bits, so that each 1
bit becomes 0, and each 0 bit becomes 1.
Table 2-8
shows examples of the effect of each of these operators:
0b0110_0110_1100_1101
b
0b0000_0000_0000_1111
a & b
0b0000_0000_0000_1101
a | b
0b0110_0110_1100_1111
a ^ b
0b0110_0110_1100_0010
~a
0b1001_1001_0011_0010






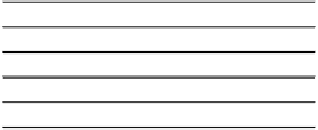




