Java Reference
In-Depth Information
The cast to type
char
of the initial value for
letter2
is essential. Without it, the code does not compile.
The expression
letter1+2
produces a result of type
int
, and the compiler does not insert an automatic
cast to allow the value to be used as the initial value for
letter2
.
The next statement outputs three characters:
System.out.println("Here\'s a sequence of letters: "+ letter1 +
letter2 +
(++letter3));
The first two characters displayed are those stored in
letter1
and
letter2
. The third character is the
value stored in
letter3
after the variable has been incremented by 1.
By default the
println()
method treats a variable of type
char
as a character for output. You can still
output the value stored in a
char
variable as a numerical value simply by casting it to type
int
. The next
statement demonstrates this:
System.out.println("Here are the decimal codes for the
letters:\n"+
letter1 + ": " + (int)letter1 +
" " + letter2 + ": " + (int)letter2 +
" " + letter3 + ": " + (int)letter3);
This statement outputs the value of each of the three variables as a character followed by its decimal
value.
Of course, you may prefer to see the character codes as hexadecimal values. You can display any value
of type
int
as a hexadecimal string by enlisting the help of a static method that is defined in the
In-
teger
class in the standard library. Add an extra output statement to the example as the last statement in
main()
:
System.out.println("Here are the hexadecimal codes for the
letters:\n"+
letter1 + ": " +
Integer.toHexString(letter1) +
" " + letter2 + ": " +
Integer.toHexString(letter2) +
" " + letter3 + ": " +
Integer.toHexString(letter3));
This statement outputs the character codes as hexadecimal values so you see this additional output:
Here are the hexadecimal codes for the letters:
A: 41 B: 42 C: 43
The
toHexString()
method generates a string representation of the argument you supply. Here you just
have the name of a variable of type
char
as the argument in each of the three uses of the method, but you
could put in any expression that results in a value of type
int
. Because the method requires an argument
of type
int
, the compiler inserts a cast to type
int
for each of the arguments
letter1
,
letter2
, and
letter3
.
The
Integer
class is related to the primitive type
int
in that an object of type
Integer
“wraps” a value
of type
int
. You will understand the significance of this better when you investigate classes in Chapter 5.
There are also classes of type
Byte
,
Short
, and
Long
that relate to values of the corresponding primitive
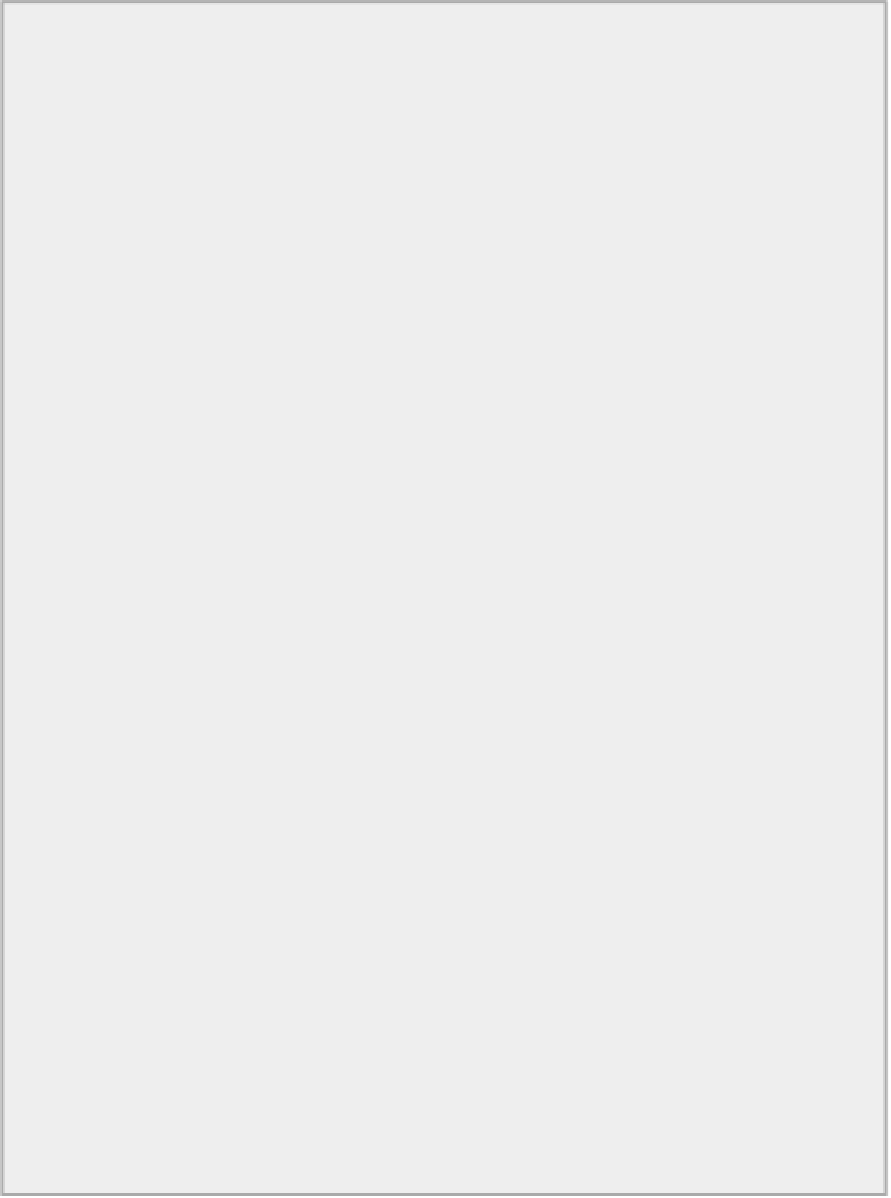