Java Reference
In-Depth Information
supplied to the
BinaryTree<T>
generic type. The same would apply if you had fields with generic types
parameterized by
T
.
After creating and displaying an array of 30 random integer values, you define a
BinaryTree<Integer>
object that stores objects of type
Integer
. The following statement does this:
BinaryTree<Integer> tree = new BinaryTree<>();
You then insert the integers into the binary tree in a loop:
for(int number:numbers) {
tree.add(number);
}
The parameter type for the
add()
method is type
Integer
, but autoboxing automatically takes care of
converting your arguments to objects of type
Integer
.
Calling the
sort()
method for
tree
returns the objects from the tree contained in a
LinkedList<In-
teger>
object:
LinkedList<Integer> values = tree.sort();
The
Integer
objects in the linked list container are ordered in ascending sequence. You list these in a
for
loop:
for(Integer value : values) {
System.out.printf("%6d", value);
if(++count%6 == 0) {
System.out.println();
}
}
You are able to use the collection-based
for
loop here because the
LinkedList<T>
type implements the
Iterable<T>
interface; this is the sole prerequisite on a container for it to allow you to apply this
for
loop to access the elements.
Just to demonstrate that
BinaryTree<>
works with more types than just
Integer
, you create an object
of type
BinaryTree<String>
that you use to store a series of
String
objects. You use essentially the
same process as you used with the integers to obtain the words sorted in ascending sequence. Note the
use of the
'-'
flag in the format specifier for the strings in the first argument to the
printf()
method.
This outputs the string left-justified in the output field, which makes the output of the strings look tidier.
Hidden Constraints in the BinaryTree<> Type
So the
BinaryTree<T>
class works well? Well, not as well as it might. The parameterized type has a built-in
constraint that was not exposed by the examples storing
String
and
Integer
objects. Suppose you define a
Person
class like this:
public class Person implements Comparable<Person> {
public Person(String name) {
this.name = name;
}
public int compareTo(Person person) {
if( person == this) {
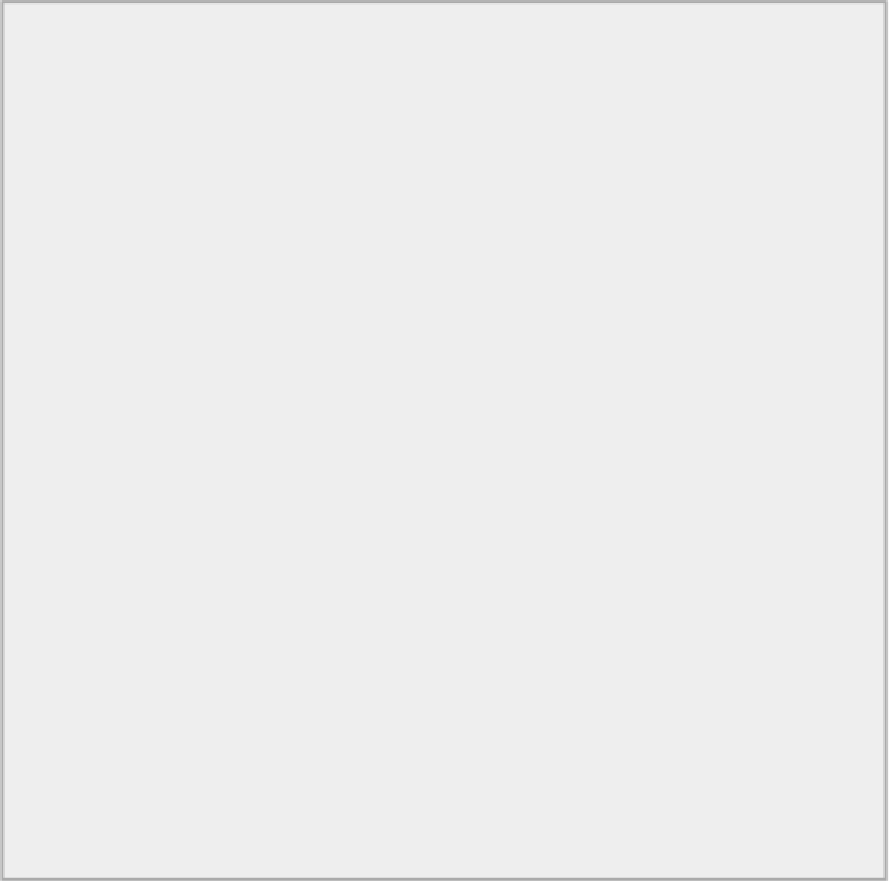