Java Reference
In-Depth Information
int maxLength = 0;
for (String saying : sayings) {
if(maxLength < saying.length())
maxLength = saying.length();
}
// The buffer length must hold the longest string
// plus its length as a binary integer
// Each character needs 2 bytes and an integer 4 bytes
ByteBuffer buf = ByteBuffer.allocate(2*maxLength + 4);
try (WritableByteChannel channel = Files.newByteChannel(
file, EnumSet.of(WRITE, CREATE,
APPEND))) {
for (String saying : sayings) {
buf.putInt(saying.length()).asCharBuffer().put(saying);
buf.position(buf.position() + 2*saying.length()).flip();
channel.write(buf);
// Write the buffer to the
file channel
buf.clear();
}
System.out.println("Proverbs written to file.");
} catch (IOException e) {
e.printStackTrace();
}
}
}
WriteProverbs.java
When you execute this, it should produce the following rather terse output following the file path spe-
cification:
Proverbs written to file.
You can check the veracity of this assertion by inspecting the contents of the file with a plain text editor.
Of course, the length values look strange because they are binary integer values, not characters.
How It Works
You create a
String
array,
sayings[]
, that contains seven proverbs. The same
ByteBuffer
object is
used to write all of the strings so it must be large enough to hold the characters from the longest string
plus a 4-byte binary integer value for the length of the string.
The strings are written to the file in the
for
loop in the
try
block that creates the
WritableByteChannel
object. You put the length of each proverb in the buffer using the
putInt()
method for the
ByteBuffer
object. You then use a view buffer of type
CharBuffer
to transfer the string to the buffer. The contents
of the view buffer,
charBuffer
starts at the current position for the byte buffer. This corresponds to the
byte immediately following the string length value because the
putInt()
method increments the buffer
position.
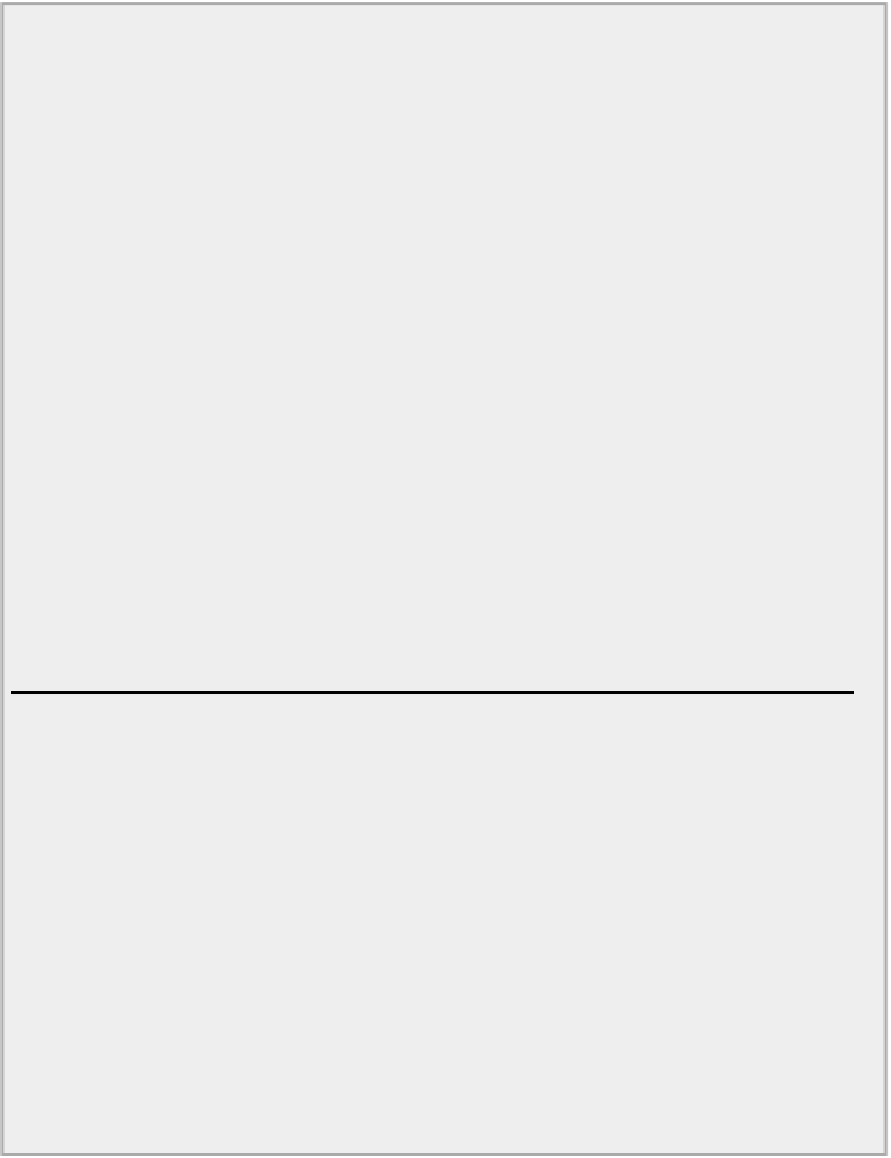
