Java Reference
In-Depth Information
NOTE
The primary purpose for the
try
block is to identify code that may result in an excep-
tion being thrown. However, you can use it to contain code that doesn't throw exceptions for
the convenience of using a
finally
block. This can be useful when the code in the
try
block
hasseveralpossibleexitpoints—
break
or
return
statements,forexample—butyoualways
want to have a specific set of statements executed after the
try
block has been executed to
make sure things are tidied up, such as closing any open files. You can put these in a
finally
block. Note that if avalue isreturned within a
finally
block, this return overrides anyreturn
statement executed in the
try
block.
Structuring a Method
You've looked at the blocks you can include in the body of a method, but it may not always be obvious how
they are combined. The first thing to get straight is that a
try
block plus any corresponding
catch
blocks
and the
finally
block all bunch together in that order:
try {
// Code that may throw exceptions...
} catch(ExceptionType1 e) {
// Code to handle exceptions of type ExceptionType1 or subclass
} catch(ExceptionType2 e) {
// Code to handle exceptions of type ExceptionType2 or subclass
... // more catch blocks if necessary
} finally {
// Code always to be executed after try block code
}
You can't have just a
try
block by itself. Each
try
block must always be followed by at least one block
that is either a
catch
block or a
finally
block.
You must not include other code between a
try
block and its
catch
blocks, or between the
catch
blocks
and the
finally
block. You can have other code that doesn't throw exceptions after the
finally
block, and
you can have multiple
try
blocks in a method. In this case, your method might be structured as shown in
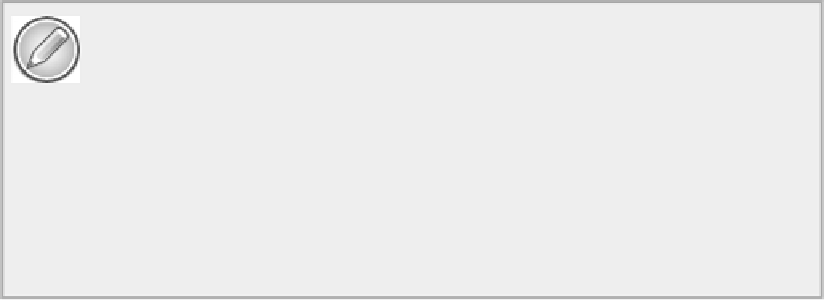