Java Reference
In-Depth Information
@Override
public String toString() {
StringBuffer str = new StringBuffer("Polyline:");
Point point = (Point) polyline.getFirst();
// Set the 1st point
as start
while(point != null) {
str.append(" ("+ point+ ")");
// Append the
current point
point = (Point)polyline.getNext();
// Make the next
point current
}
return str.toString();
}
private LinkedList polyline;
// The linked list
of points
}
Directory "TryPolyLine2"
You can exercise this using the same code as last time — in the
TryPolyLine.java
file. Copy this file
to the directory for this example. Don't forget to copy
Point.java
, too. I changed the name of the file
from
TryPolyLine.java
to
TryPolyLine2.java
and the class name of course.
How It Works
The
PolyLine
class implements all the methods that you had in the class before, so the
main()
method in
the
TryPolyLine
class works just the same. Under the covers, the methods in the
PolyLine
class work a
little differently. The work of creating the linked list is now in the constructor for the
LinkedList
class.
The
PolyLine
class constructors just assemble a point array if necessary and call the
LinkedList
con-
structor. Similarly, the
addPoint()
method creates a
Point
object from the coordinate pair it receives
and passes it to the
addItem()
method for the
LinkedList
object,
polyline
.
Note that the cast from
Point
to
Object
when the
addItem()
method is called is automatic. A cast from
any class type to type
Object
is always automatic because the class is up the class hierarchy — remem-
ber that all classes have
Object
as a base. In the
toString()
method, you must insert an explicit cast to
store the object returned by the
getFirst()
or the
getNext()
method. This cast is down the hierarchy
so you must specify the cast explicitly.
You could use a variable of type
Object
to store the objects returned from
getFirst()
and
getNext()
,
but this would not be a good idea. You would not need to insert the explicit cast, but you would lose a
valuable check on the integrity of the program. You put objects of type
Point
into the list, so you would
expect objects of type
Point
to be returned. An error in the program somewhere could result in an object
of another type being inserted. If the object is not of type
Point
— due to the said program error, for
example — the cast to type
Point
fails and you get an exception. A variable of type
Object
can store
anything. If you use this, and something other than a
Point
object is returned, it does not register at all.
Now that you have gone to the trouble of writing your own general linked list class, you may be wonder-
ing why someone hasn't done it already. Well, they have! The
java.util
package defines a
LinkedList
class that is much better than this one. Still, putting your own together was a good experience, and I hope
you found it educational, if not interesting. The way you have implemented the
LinkedList
class here is
not the best approach. In Chapter 13 you learn about
generic types
, which enable you to define a linked
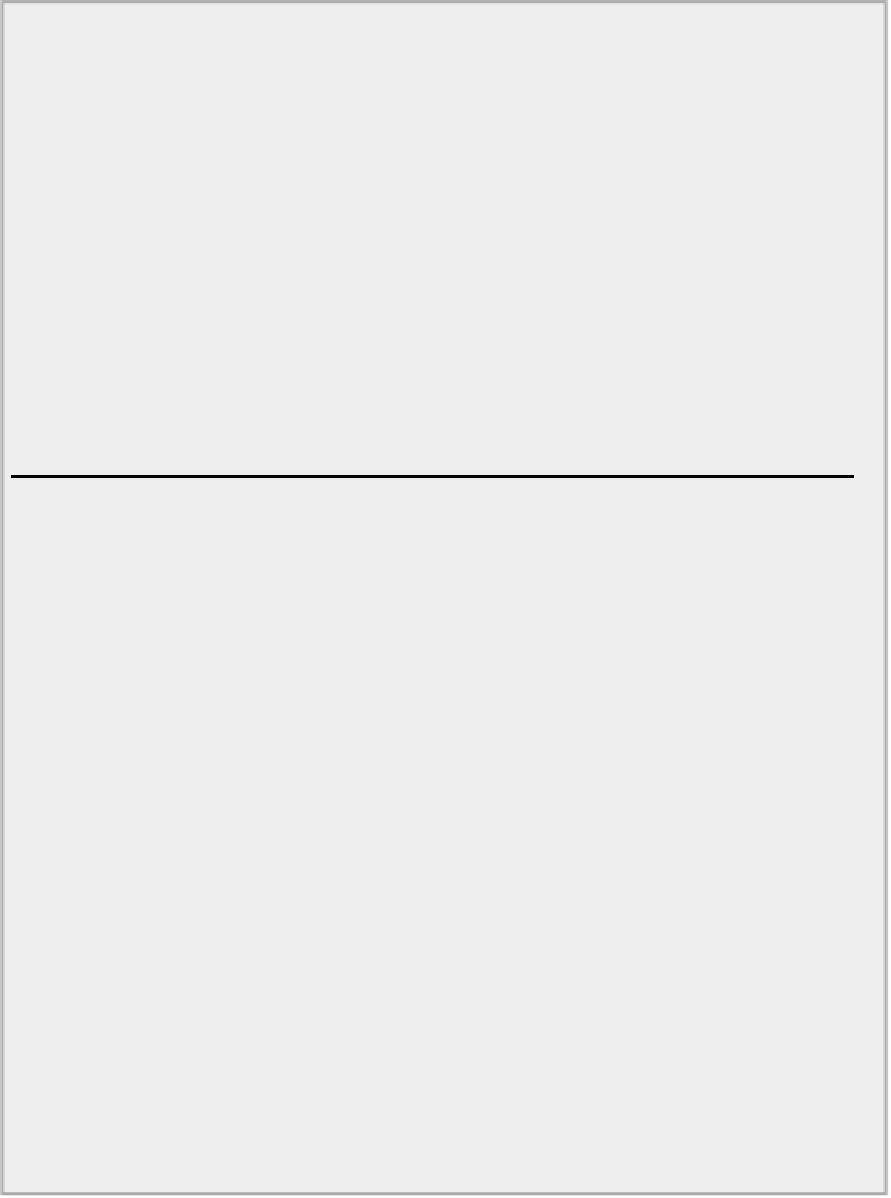
