Java Reference
In-Depth Information
The
getFirst()
and
getNext()
methods are intended to be used together to access all the objects stored
in the list. The
getFirst()
method returns the object stored in the first
ListItem
object in the list and
sets the
current
data member to refer to the first
ListItem
object. After calling the
getFirst()
method,
successive calls to the
getNext()
method return subsequent objects stored in the list. The method updates
current
to refer to the next
ListItem
object, each time it is called. When the end of the list is reached,
getNext()
returns
null
.
TRY IT OUT: Using the General Linked List
You can now define the
PolyLine
class so that it uses a
LinkedList
object. All you need to do is put
a
LinkedList
variable as a class member that you initialize in the class constructors, and implement all
the other methods you had in the previous version of the class to use the
LinkedList
object:
public class PolyLine {
// Construct a polyline from an array of coordinate pairs
public PolyLine(double[][] coords) {
Point[] points = new Point[coords.length];
// Array to hold
points
// Create points from the coordinates
for(int i = 0; i < coords.length ; ++i) {
points[i] = new Point(coords[i][0], coords[i][1]);
}
// Create the polyline from the array of points
polyline = new LinkedList(points);
}
// Construct a polyline from an array of points
public PolyLine(Point[] points) {
polyline = new LinkedList(points);
// Create the
polyline
}
// Add a Point object to the list
public void addPoint(Point point) {
polyline.addItem(point);
// Add the point to
the list
}
// Add a point from a coordinate pair to the list
public void addPoint(double x, double y) {
polyline.addItem(new Point(x, y));
// Add the point to
the list
}
// String representation of a polyline
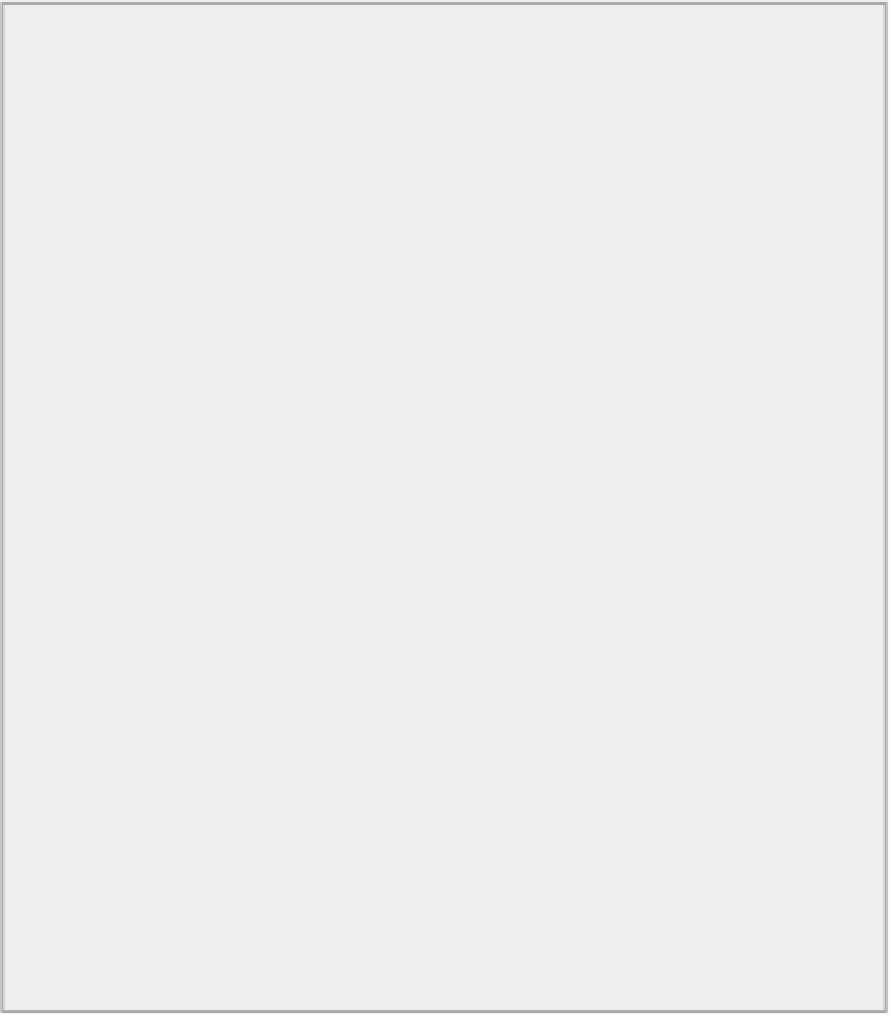
