Java Reference
In-Depth Information
Primes.java
You should get the following output:
2
3
5
7
11
13
17
19
23
29
31
37
41
43
47
How It Works
There are much more efficient ways to calculate primes, but this program demonstrates the
break
state-
ment in action. The first step in
main()
is to declare two variables:
int nValues = 50; // The maximum value to
be checked
boolean isPrime = true; // Is true if we find a
prime
The first variable is the upper limit for integers to be checked to see if they are prime. The
isPrime
vari-
able is used to record whether a particular value is prime or not.
The basic idea of the program is to go through the integers from 2 to the value of
nValues
and check
each one to see if it has an integer divisor less than itself. The nested loops do this:
for(int i = 2; i <= nValues; ++i) {
isPrime=true; // Assume the current i is prime
// Try dividing by all integers from 2 to i-1
for(int j = 2; j < i; ++j) {
if(i % j == 0) { // This is true if j divides exactly
isPrime = false; // If we got here, it was an exact
division
break; // so exit the loop
}
}
// We can get here through the break, or through completing the
loop
if(isPrime) // So is it prime?
System.out.println(i); // Yes, so output the value
}
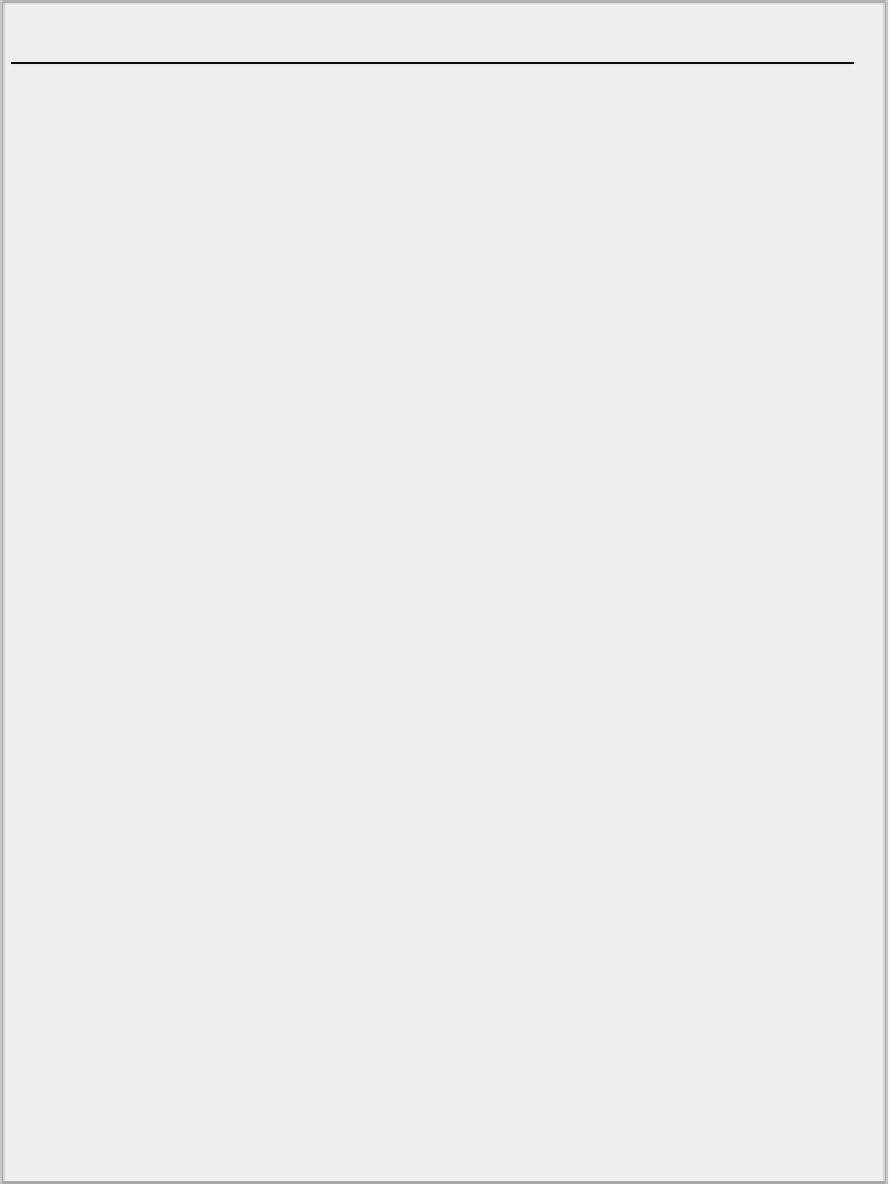
