Java Reference
In-Depth Information
Although testing characters using logical operators is a useful way of demonstrating how these operators
work, in practice there is an easier way. The standard Java packages provide a range of standard methods to
do the sort of testing for particular sets of characters such as letters or digits that you have been doing with
if
statements. They are all available within the
Character
class, which is automatically available in your
programs. For example, you could have written the
if
statement in the
LetterCheck2
program as shown in
the following example.
TRY IT OUT: Deciphering Characters Trivially
In the following example, the
if
expressions in
main()
that were in the
LetterCheck2
class have been
replaced by expressions that call methods in the
Character
class to do the testing:
import static java.lang.Character.isLowerCase;
import static java.lang.Character.isUpperCase;
public class LetterCheck3 {
public static void main(String[] args) {
char symbol = 'A';
symbol = (char)(128.0*Math.random()); // Generate a random
character
if(isUpperCase(symbol)) {
System.out.println("You have the capital letter " + symbol);
} else {
if(isLowerCase(symbol)) {
System.out.println("You have the small letter " + symbol);
} else {
System.out.println("The code is not a letter");
}
}
}
}
LetterCheck3.java
How It Works
Because you have the
import
statements for the
isUpperCase
and
isLowerCase
method names at the
beginning of the source file, you can call these methods without using the
Character
class name as qual-
ifier. The
isUpperCase()
method returns
true
if the
char
value that you pass to it is uppercase, and
false
if it is not. Similarly, the
isLowerCase()
method returns
true
if the
char
value you pass to it is
lowercase.
for testing characters. In each case, you put the argument of type
char
that is to be tested between the
parentheses following the method name.
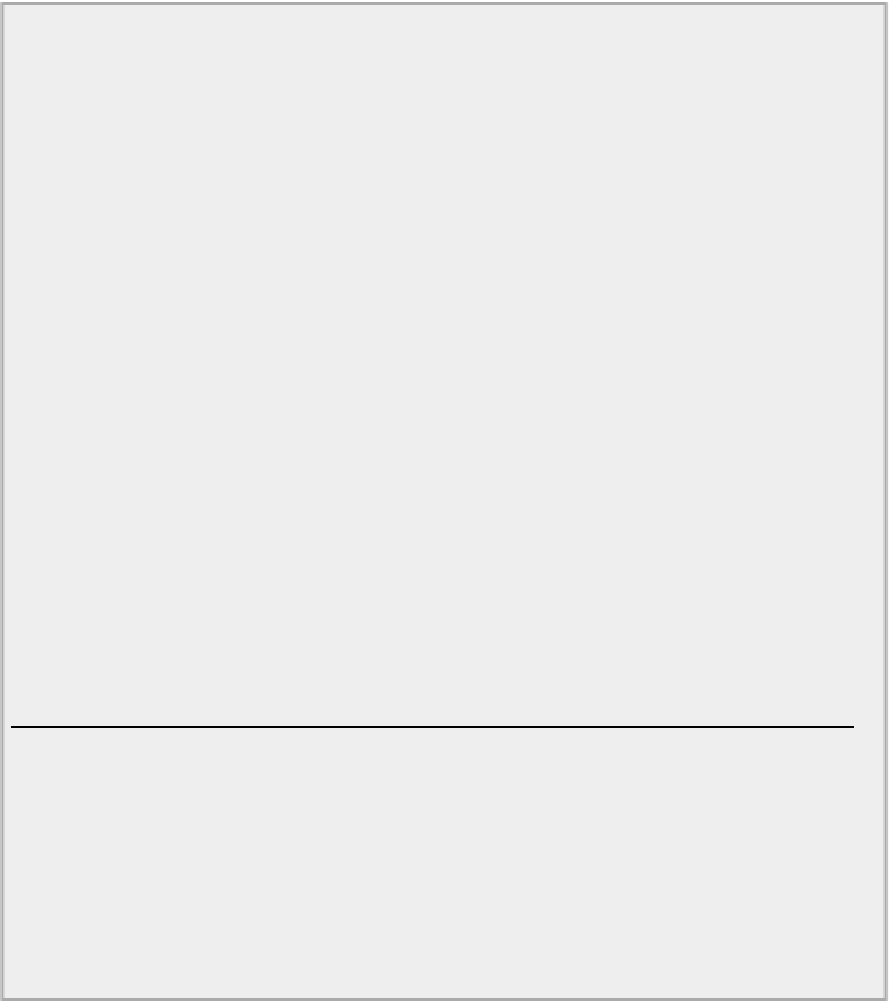

