HTML and CSS Reference
In-Depth Information
The last fill option to look at involves using a fill pattern. You need an external image,
which is applied as a pattern throughout the shape. For example, you can use a texture that
you created as a background to your canvas by using the following code:
var ctxt = drawingSurface.getContext("2d");
ctxt.lineWidth = 3;
ctxt.rect(150, 150, 200, 125);
var img = new Image();
img.src = "texture.png";
img.onload = function () {
var pat = ctxt.createPattern(img, "repeat");
ctxt.fillStyle = pat;
ctxt.fill();
ctxt.stroke();
}
Figure 1-38 shows the output of this code.
FIGURE 1-38
The canvas filled with a pattern drawn on it
The preceding code calls the
createPattern
method and passes it a reference to an Image
object and a repeat pattern. The repeat pattern can be
no-repeat
,
repeat-x
, or
repeat-y
, but
it defaults to
repeat
if you don't specify anything. You need to assign an event handler to the
onload event of the Image object to ensure that you draw the pattern only after the image
loads. Otherwise, the code could run before the picture is rendered, and the pattern won't
display.
This section has covered a lot about how to work with shapes and fill them. All your graphics
have been created by using code to manually draw shapes. Next, you see how to draw exist-
ing graphics from external files on your canvas, and then you look at drawing text.
Drawing images
Drawing images on a canvas is just as straightforward as the other drawing methods you've
seen. To draw an image on a canvas, you use the
drawImage
method of the context object.
This method takes an Image object and some (x,y) coordinates to define where the image
should be drawn. Just like with the rectangle, the image's top-left corner is drawn at the
specified (x,y). The default size of the image is the actual image size, but as you will see right
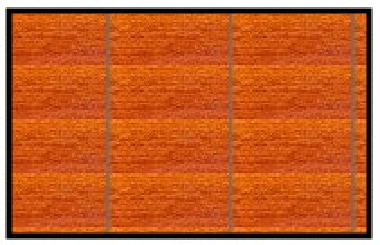

Search WWH ::

Custom Search